In this section, we’ll proceed further and look at different projection strategies. So, the first case which I am using here is the Object Initializers. With C# Object Initializers, we can project the same into more complex types. For example, suppose, as a first step in a query, we want to strip vowels from a list of names while still keeping the original versions alongside, for the benefit of subsequent queries. Therefore, we can write as shown below:
void Main()
{
var names = new[] { "Rahul", "Sahay", "Tom", "Dave", "Hari" }.AsQueryable();
IEnumerable<TempProjectionItem> temp =
from n in names
select new TempProjectionItem
{
Original = n,
Vowelless = n.Replace ("a", "").Replace ("e", "").Replace ("i", "").Replace
("o", "").Replace ("u", "")
};
temp.Dump();
}
class TempProjectionItem
{
public string Original;
public string Vowelless;
}
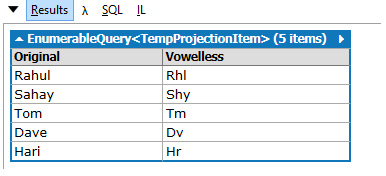
However, Anonymous Types allow you to structure your intermediate results without writing special classes. So, here we can remove TempProjectionItem
as shown below in the example.
void Main()
{
var names = new[] { "Rahul", "Sahay", "Tom", "Dave", "Hari" }.AsQueryable();
var intermediate = from n in names
select new
{
Original = n,
Vowelless = n.Replace ("a", "").Replace ("e", "").Replace ("i", "").Replace
("o", "").Replace ("u", "")
};
(
from item in intermediate
where item.Vowelless.Length > 2
select item.Original
)
.Dump();
(
from n in names
select new
{
Original = n,
Vowelless = n.Replace ("a", "").Replace ("e", "").Replace ("i", "").Replace
("o", "").Replace ("u", "")
}
into temp
where temp.Vowelless.Length > 2
select temp.Original
)
.Dump ("With the 'into' keyword");
}
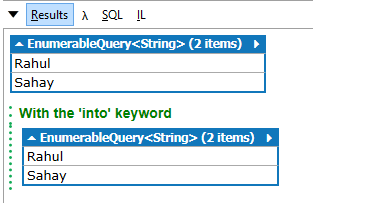
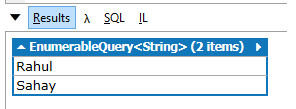
The let
keyword introduces a new variable alongside the range variable. With let
, we can write a query extracting string
s whose length, excluding vowels, exceeds two characters, as follows:
void Main()
{
var names = new[] { "Rahul", "Sahay", "Tom", "Dave", "Hari" }.AsQueryable();
(
from n in names
let vowelless = n.Replace ("a", "").Replace ("e", "").Replace ("i", "").Replace
("o", "").Replace ("u", "")
where vowelless.Length > 2
orderby vowelless
select n
)
.Dump();
}
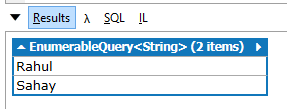
The compiler resolves a let
clause by projecting into a temporary anonymous type that contains both the range variable and the new expression variable. Thanks for joining me. In the next section, we’ll delve further and check other pieces as well. Till then, stay tuned and happy coding!
