Introduction
WebDriver is a tool for automating testing web applications, and, in particular, to verify that they work as expected. It aims to provide a friendly API that’s easy to explore and understand, which will help make your tests easier to read and maintain. It’s not tied to any particular test framework so that it can be used equally well with MSTest, NUnit, TestNG, JUnit and so on. This “Getting Started” guide introduces you to WebDriver’s C# API and helps get you started becoming familiar with it.
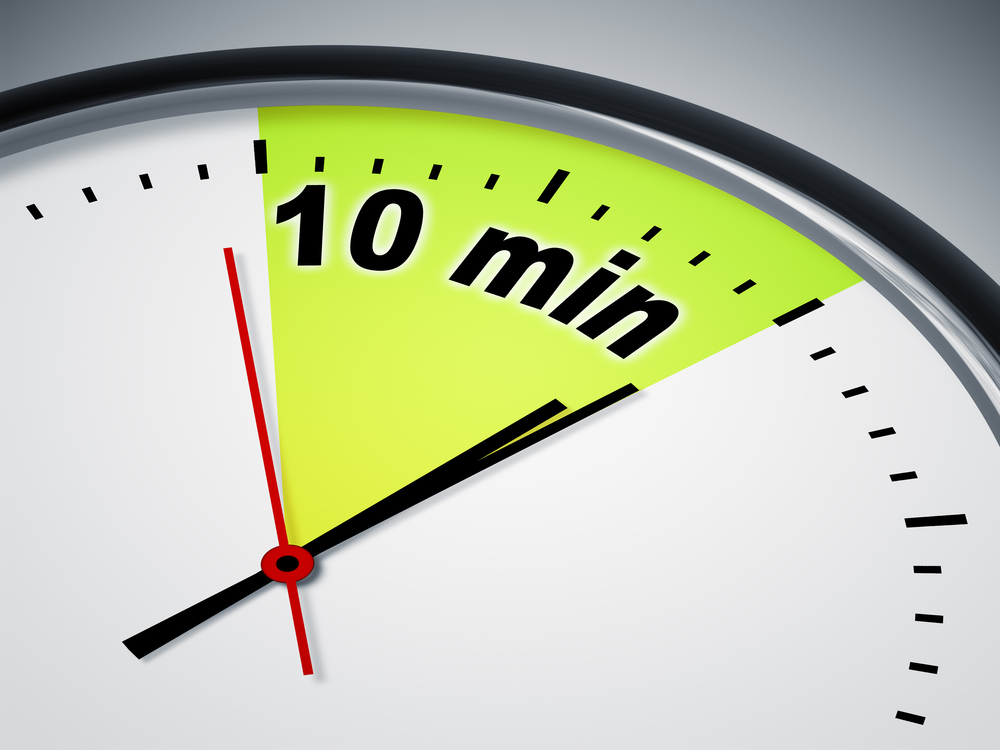
Create Your First WebDriver Test Project
- Create New Test Project in Visual Studio.
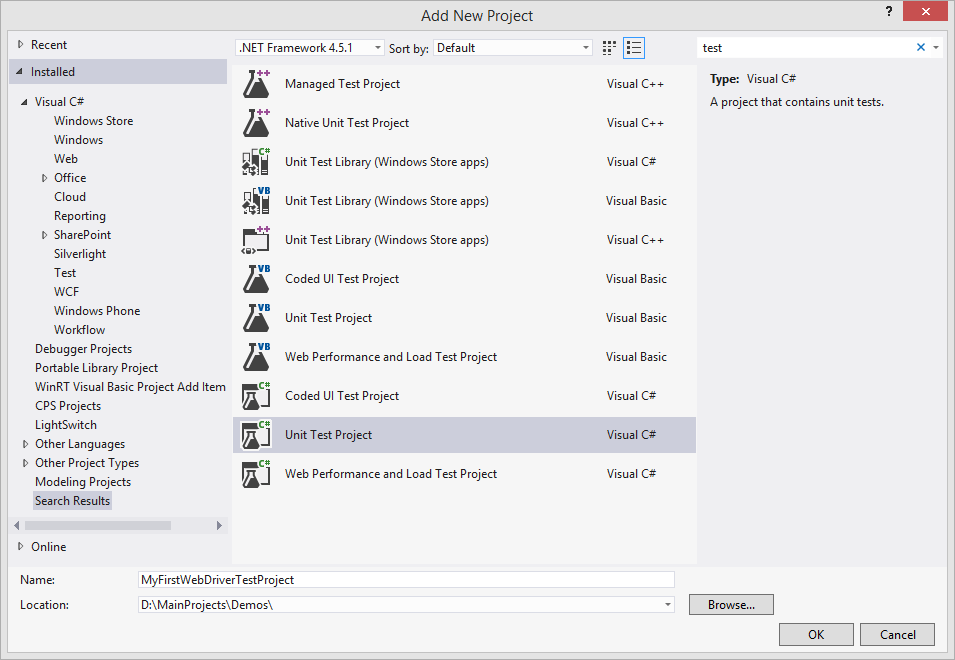
- Install NuGet package manager and navigate to it.
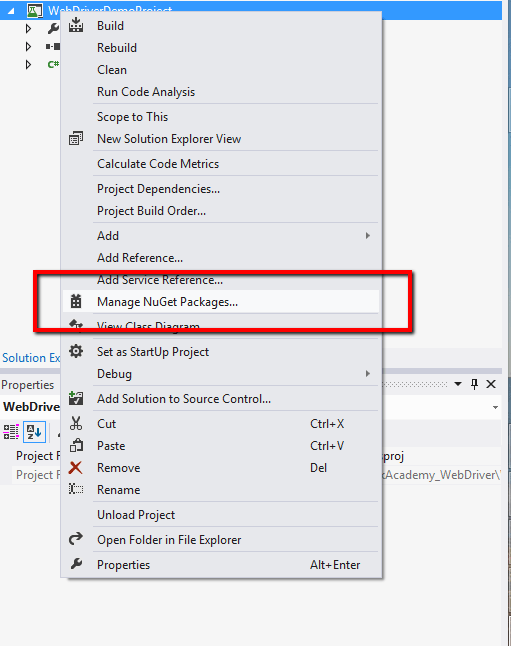
- Search for Selenium and install the first item in the result list.
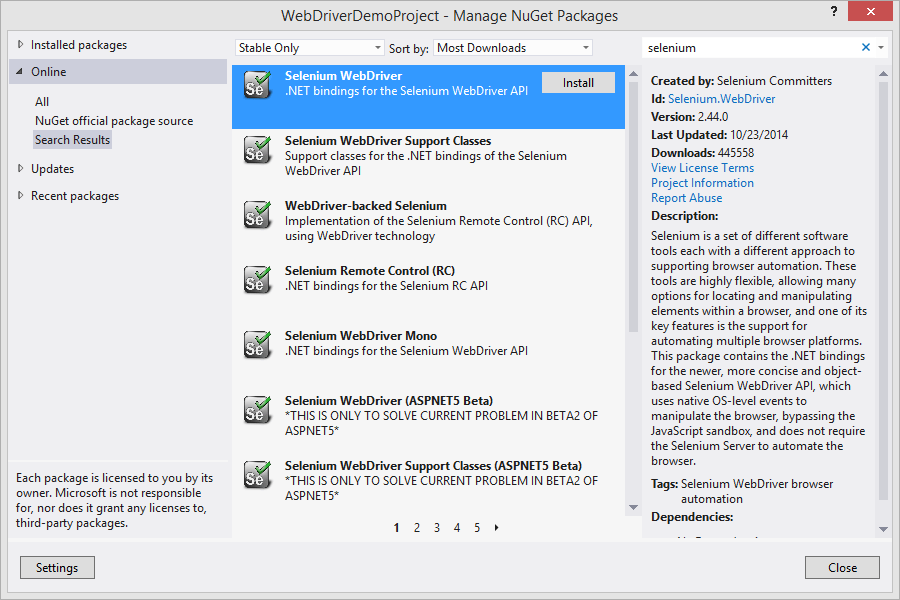
Code Examples In WebDriver
Create an instance of a driver.
IWebDriver driverOne = new FirefoxDriver();
IWebDriver driverTwo = new InternetExlorerDriver("C:\\PathToMyIeDriverBinaries\");
Only the FirefoxDriver
can be created without parameters. For all other drivers, you need to point the location where the particular driver is downloaded.
?dditional steps are required to use Chrome Driver, Opera Driver, Android Driver and iPhone Driver.
Navigate to specific URL.
driverOne.Navigate().GoToUrl("<a href="http:
Locating Elements with WebDriver
By ID
IWebElement element = driverOne.FindElement(By.Id("myUniqueId"));
By Class (Find more than one element on the page)
IList<IWebElement> elements = driverOne.FindElements(By.ClassName("green"));
By Tag Name
IWebElement frame = driverOne.FindElement(By.TagName("iframe"));
By Name
IWebElement cheese = driverOne.FindElement(By.Name("goran"));
By Link Text
IWebElement link = driverOne.FindElement(By.LinkText("best features"));
By XPath
IList<IWebElement> inputs = driverOne.FindElements(By.XPath("//input"));
By CSS Selector
IWebElement css = driverOne.FindElement(By.CssSelector("#green span.dairy.baged"));
Chaining Locators
Use a Chain of Locators to find a particular element.
IWebElement hardToFind = this.driverOne.FindElement
(By.ClassName("firstElementTable")).FindElement(By.Id("secondElement"));
IWebElement Methods
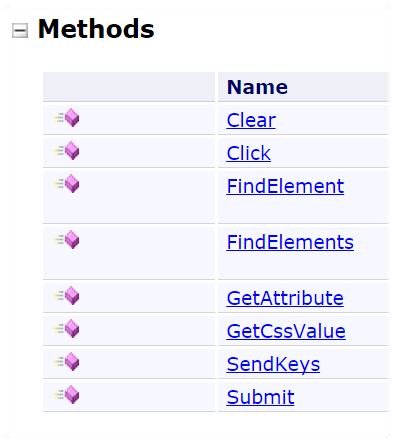
IWebElement Properties
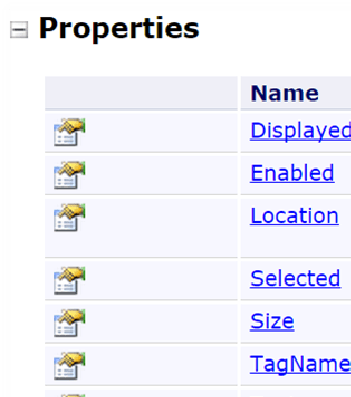
HTML Element Actions in WebDriver
Type Text into a field using Selenium WebDriver SendKeys()
function.
IWebElement element = driverOne.FindElement(By.Name("search"));
element.SendKeys("Automate The Planet!");
Select Drop Down Value. First, you need to add NuGet Package to your project- Selenium.Support
.
SelectElement selectElement = new SelectElement(driver.FindElement(By.XPath("/html/body/select")));
selectElement.SelectByText("Planet");
So Far in the 'Pragmatic Automation with WebDriver' Series