The proper way of creating an HTML table
with JavaScript and inserting it into the HTML body
element is using the DOM methods insertRow and insertCell, as in the following code example:
function createTable() {
var i=0, rowEl=null,
tableEl = document.createElement("table");
for (i=1; i <= 10; i++) {
rowEl = tableEl.insertRow();
rowEl.insertCell().textContent = "table cell "+ i +"-1" ;
rowEl.insertCell().textContent = "table cell "+ i +"-2" ;
}
document.body.appendChild( tableEl);
}
The DOM method insertRow
can be called on a table
element for creating and inserting a new row, while insertCell
can be called on a row element for creating and inserting a new cell.
Many web developers are not familiar with the DOM, or still think (mistakenly) that it's not well supported by browsers or too slow, and create HTML elements in the form of string
s that are inserted into the DOM using the innerHTML
attribute. This is not a safe, and certainly not a best practice, approach.
The following UML class diagram summarizes the DOM interfaces for programmatically manipulating table elements. Notice that insertRow
can also be called on tHead
and tFoot
, which are table section elements.
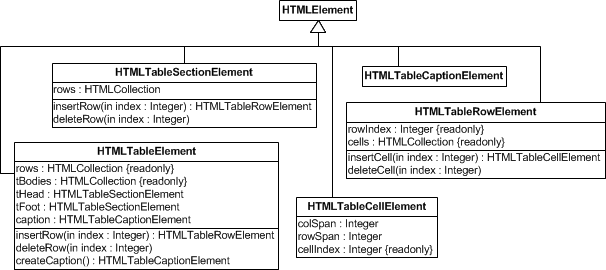
For reading more about how to use DOM attributes and methods for building web apps, see my book Building Front-End Web Apps with Plain JavaScript.