This post is about uploading files to ASP.NET 5 web application using HTML5 file API. Most of the HTML5 File upload examples are using the “HttpContext.Current.Request.Files
” which is not implemented in the ASP.NET5. The alternative is to use IFormFile, it is working fine, if you are using the normal Form - File upload combination. But in case of Ajax file upload it is not working. Later I found a SO post, which talks about File upload in ASP.NET5. It is mentioned like you need to read the body contents and save it using file stream. But when I did that, it was also not working. Later I found that the body contains the filename and content type information as well like this.
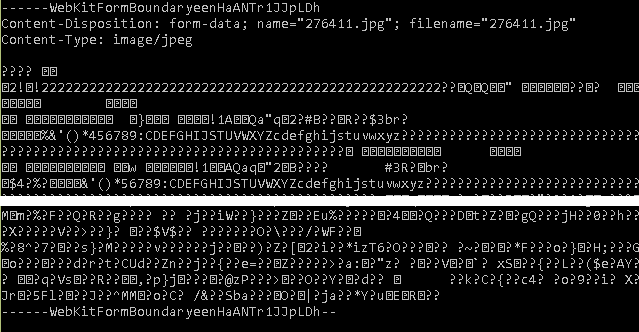
Since I have the content type and file name, saving the file stream as file won’t create a valid image file. You need a parser to parse the contents. Initially I thought of implementing one, but later I found one which is already available in codeplex - Multipart Form Data Parser. Using this you can parse the body and get the file name, content type and file contents.
Here is the complete code.
HTML Form
1 <form id="UploadForm" asp-action="upload" asp-controller="home">
2 <input class="form-control" type="file" name="UploadFile" id="UploadFile" accept="image/*" />
3 <input type="submit" value="Submit" class="btn btn-default" />
4 </form>
And here is script, which handles the Ajax file upload.
1 $("#UploadForm").submit(function (e) {
2 var data = new FormData();
3 var selectedFiles = $("#UploadFile")[0].files;
4 data.append(selectedFiles[0].name, selectedFiles[0]);
5
6 $.ajax({
7 type: "POST",
8 url: "/home/Upload",
9 contentType: false,
10 processData: false,
11 data: data,
12 success: function (result) {
13 alert(result);
14 },
15 error: function () {
16 alert("There was error uploading files!");
17 }
18 });
19
20 e.preventDefault();
21 });
And here is the controller code.
1 [HttpPost]
2 public IActionResult Upload()
3 {
4 MultipartParser multipartParser =
5 new MultipartParser(new StreamReader(Request.Body).BaseStream);
6 if(multipartParser.Success)
7 {
8 var bytes = multipartParser.FileContents;
9 }
10
11 return Json("Uploaded");
12 }
Hope it helps. Happy Programming