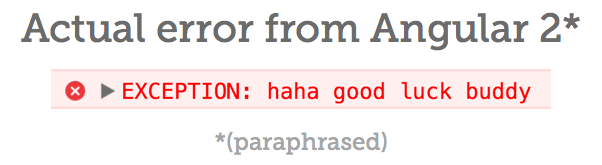
If you’ve begun to try out Angular 2, you might have encountered some pretty baffling errors. I know I sure did…
It always feels a bit frustrating to start fresh in a new library or language (or both, in this case) – all your previous experience goes out the window, and you’re back to making beginner mistakes and hitting confusing errors.
Here’s a list of some errors I ran into, my best guesses as to what caused them, and how I fixed them.
The relevance of these errors may (hopefully) decay over time – these ones were encountered in Angular 2 Beta 0 or a late Alpha version.
General Advice
-
If you get an error in the console, scroll to the top! The later errors are not very helpful. Look at the first one.
-
Some of these can be really tricky to track down. As a general strategy, make small changes and test as you go. At least that way, it’ll be easier to figure out which bit of code broke (like when the stack traces are not so helpful).
-
Styles don’t seem to cascade to subcomponents by default…
Errors, Errors, Everywhere…
EXCEPTION: TypeError: allStyles.map is not a function
‘styles’ needs to be an array of strings, not a single string.
EXCEPTION: Component ‘Something’ cannot have both ‘styles’ and ‘@View’ set at the same time”
I provided a @View
decorator, and also put a ‘styles’ key in the @Component
– this doesn’t work. Put ‘styles’ in the object passed to @View
.
EXCEPTION: Unexpected directive value ‘undefined’ on the View of component ‘AppComponent’
I defined a component as class Something
when it should’ve been export class Something
– forgot to export it from the file it was defined in. The import
didn’t fail, but the directives: [Something]
did…
EXCEPTION: No provider for UserList! (UserCmp -> UserList)
I forgot to add UserList
to the viewProviders
array in the parent view. The fix was @Component({viewProviders: [UserList]})
EXCEPTION: Cannot resolve all parameters for UserList(?). Make sure they all have valid type or annotations.
I got this when I was trying to inject the Http
service.
For WHY this happens, see Injecting services in services in Angular 2.
There are 2 different ways to fix this:
Way #1: Put @Inject(Http)
before the param that should be injected with Http:
constructor(public http: Http)
constructor(@Inject(Http) public http: Http)
Way #2: Put @Injectable()
(don’t forget the parens!) above the class
@Injectable()
constructor(public http: Http)
EXCEPTION: Error during instantiation of UsersCmp
TypeError: this.http.get(…).map is not a function
I thought TypeScript was supposed to save us from TypeErrors!
Removing .map
fixed the error… but it seems like it should work. I don’t know too much about this new Http service so, maybe the request failed?
Nope: You have to explicitly include the map
operator from RxJS. Awesome. Add this line to app.ts (or whatever your main file is):
import 'rxjs/add/operator/map';
EXCEPTION: Cannot find a differ supporting object ‘[object Object]’ in [users in UsersCmp@2:14]
I’m pretty sure this means that a non-array was passed to ngFor
. Make sure the thing you pass is an array.
Compile error: “Type ‘Observable<response>' is not assignable to type 'any[]'."
Got this when I was trying to return this.http.get()
as any[]
– it’s not an array, so that doesn’t work. Changing to any
works better (or the actual type, for that matter).
and my personal favorite:
EXCEPTION: [object Object]
WTF? Caused by:
return this.http.get('/users/list')
.map(res => res.json())
.subscribe(users => this.users = users);
Without an error handler, a 404 response causes an exception.
Call subscribe this way instead:
.subscribe(
res => res.text(),
err => handleErr(err),
() => console.log('done'));
Angular 2 Errors was originally published by Dave Ceddia at Angularity on January 19, 2016.
CodeProject