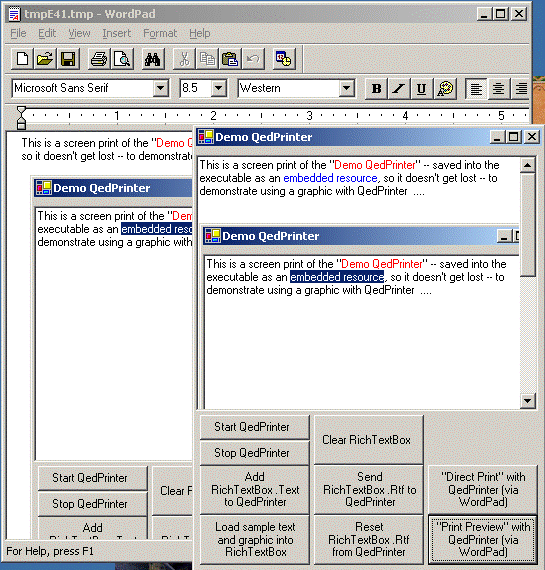
Introduction
It seems to be always true that one thing leads to another; this component is at two or three removes from the actual application I'm writing to interface with my employer's customer base. In the course of developing and testing the application, I realized that I wanted to be able to print "stuff" now. After several hours of on-line searching, while I'd come across several interesting articles and classes implementing the PrintDocument
class (from which I intend to someday learn how to use that class), I'd not found a simple way to print simple text without investing time I don't have at the moment in learning to use the PrintDocument
class.
So, since it was looking as though I'd have to write my own class/component to do what I wanted, I stopped looking for a ready-made one and did just that. I've found The Code Project to be a great resource and have been intending for some time to get around to writing and submitting articles on some classes/components (both original and extensions of others' work) I've written; QedPrinter
seems to me a good case to use as my first.
Note: QedPrinter
contains no form; the above image is of the demo project superimposed on an instance of WordPad which it spawned via QedPrinter.
Using the code
As I've written it, the interface to QedPrinter
is strictly via static class methods and properties. These class methods construct, manipulate and dispose the actual instance of the class. Thus, as written, in an application making use of QedPrinter
, there can exist at most one instance at any one time.
QedPrinter
makes use of a RichTextBox
as its internal buffer. An application making use of QedPrinter
may modify the internal buffer in one of two ways:
- by appending string values to its
.Text
property, or
- by setting its
.Rtf
property to that of a RichTextBox
the application has constructed/populated.
Using option 2, any graphics and text formatting supported by the RichTextBox
class are supported by QedPrinter
.
To use QedPrinter
as is, add a reference in your project to the class library 'TDHQedPrinter.dll'. The namespace used in that library is:
using TDHControls.TDHQedPrinter;
The members of QedPrinter
's interface are:
_Start()
- This method constructs the instance of QedPrinter
(if it doesn't already exist), returning true
if the instance was successfully constructed (or already existed), or false
upon failure to construct it.
_Stop()
- This method disposes the instance, returning true
upon success, or false
upon failure.
_Clear()
- This method initializes the internal buffer of the QedPrinter
instance. An overload of this method accepts a string value to which the internal buffer is initialized.
_Print()
- This method directly prints, via WordPad, the contents of the internal buffer of the QedPrinter
instance.
_PrintPreview()
- This method opens, in WordPad, the contents of the internal buffer of the QedPrinter
instance.
_Started
- This boolean property returns a value indicating whether the instance exists (i.e. has been started).
_AppendLine
- This accessor accepts a string assignment, which is appended (as a new line) to the .Text
of the internal buffer of the QedPrinter
instance.
_AppendText
- This accessor accepts a string assignment, which is appended to the .Text
of the internal buffer of the QedPrinter
instance.
_RichTextBox
- This accessor accepts or returns a RichTextBox
(i.e. the internal buffer). The {set}
accessor sets the the internal buffer's .Rtf
property to that of the assignment. The {get}
accessor returns the RichTextBox
internal buffer, the properties of which can then be accessed.
Sample code making use of QedPrinter
:
using TDHControls.TDHQedPrinter;
private void SomeMethod()
{
System.Windows.Forms.RichTextBox aRichTextBox = new RichTextBox();
QedPrinter._Start();
SomeOtherMethod(aRichTextBox);
aRichTextBox = new RichTextBox();
aRichTextBox.Rtf = QedPrinter._RichTextBox.Rtf;
YetSomeOtherMethod();
aRichTextBox.Text = QedPrinter._RichTextBox.Text;
aRichTextBox.Rtf = QedPrinter._RichTextBox.Rtf;
QedPrinter._Stop();
}
private void SomeOtherMethod(System.Windows.Forms.RichTextBox
parmRichTextBox;)
{
QedPrinter._RichTextBox = parmRichTextBox;
QedPrinter._Print();
}
private void YetSomeOtherMethod()
{
if (QedPrinter._Started)
{
QedPrinter._Clear("Hey! I'm a brash new Method()");
QedPrinter._AppendLine = "I don't care" +
" about whatever might have come before!";
QedPrinter._AppendLine = "";
}
else
{
QedPrinter._Start();
}
QedPrinter._AppendLine = "This will be on a new line.";
QedPrinter._AppendText = "\r\n" + "So will this.";
QedPrinter._AppendText = "This, however," +
" will be all run-on with the previous line.";
QedPrinter._AppendText = "It will no doubt be an ugly mess.";
QedPrinter._AppendText = "Andgettingworsebytheminute.";
QedPrinter._AppendLine = "But enough of this foolishness!";
QedPrinter._PrintPreview();
}
Behind the Scenes
The QedPrinter._Print()
and QedPrinter._PrintPreview()
methods work thusly:
- Spawn a new thread, which:
- Creates a temporary file, using the
RichTextBox
's .SaveFile()
method.
- Spawns a new process to start WordPad, passing it this temporary file.
- Deletes the temporary file after the WordPad process terminates.
History
- 2005 July: creation of
QedPrinter
and submission to The Code Project.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.