In this MEAN Stack Tutorial, we are going to develop a RESTful API using Express.js and MongoDB. We will follow a step by step approach to make it easier for readers in developing their first RESTful API on MEAN stack.
Following are the steps to build the API:
Step 1: Initialize the Project
- Create a folder with the project name such as todoapp.
- Open command prompt window on the project folder.
- Run the following command:
npm init
This command will create a package.json file with the following details:
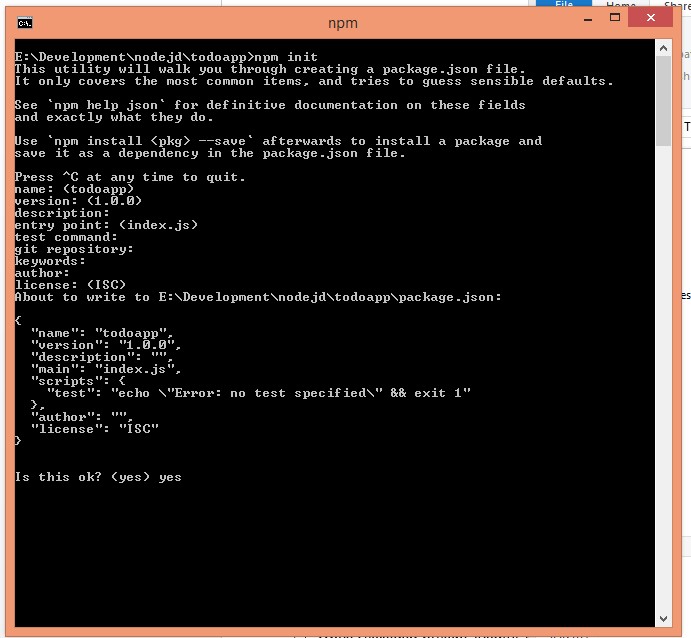
Step 2: Install Express
Step 3: Install Express Generator
Step 4: Create the Project
- Use the following command to create the express project:
express todoapp
This will give the following output:
create : todoapp
create : todoapp/package.json
create : todoapp/app.js
create : todoapp/public
create : todoapp/routes
create : todoapp/routes/index.js
create : todoapp/routes/users.js
create : todoapp/views
create : todoapp/views/index.jade
create : todoapp/views/layout.jade
create : todoapp/views/error.jade
create : todoapp/public/stylesheets
create : todoapp/public/stylesheets/style.css
create : todoapp/public/javascripts
create : todoapp/public/images
create : todoapp/bin
create : todoapp/bin/www
install dependencies:
$ cd todoapp && npm install
run the app:
$ DEBUG=todoapp ./bin/www
Step 5: Install Dependencies
- The package.json file will look like the following:
{
"name": "todoapp",
"version": "0.0.0",
"private": true,
"scripts": {
"start": "node ./bin/www"
},
"dependencies": {
"body-parser": "~1.16.0",
"cookie-parser": "~1.4.3",
"debug": "~2.6.0",
"express": "~4.14.1",
"jade": "~1.11.0",
"morgan": "~1.7.0",
"serve-favicon": "~2.3.2"
}
}
- Install all the dependencies using the following command:
npm install
Note: You can find more helpful details on MEAN Stack, especially AngularJS 1.x & AngularJS 2 here.
Step 6: Install HBS, MONGO
Step 7: Run Mongo
- Run mongod.exe, it will start the MongoDB.

- Connect to
todoapp
database using the following command:

Step 8: Create Mongo Service and Connect to Database
- On app.js file, add mongo database as follows:
var mongo = require('mongodb');
var monk = require('monk');
var db = monk('localhost:27017/todoapp');
- Create a middleware to connect to database:
app.use(function(req,res,next){
req.db = db;
next();
});
- Create a new router for todo in 'routes/todo.js' file:
var express = require('express');
var router = express.Router();
/* GET todos listing. */
router.get('/', function(req, res) {
res.send('Show todo list with delete and update feature (RUD)');
});
/* render NEW To-do form page. todos/new is a route path*/
router.get('/new', function(req, res) {
res.render('new_todo',{title:'Add new To-Do'});
});
module.exports = router;
- In app.js, add the router for initialization:
var todos = require('./routes/todos');
app.use('/todos', todos);
- Create a new form template inside views folder with the name 'new_todo.jed':
extends layout
block content
h1= title
form(name="new_todo", method="post", action="/todos/add_todo")
div.input
span.label Title
input(type="text", name="title")
div.input
span.label Due Date
input(type="text", name="dueDate")
div.input
span.label Description
textarea(name="description", cols="40", rows="5")
div.actions
input(type="submit", value="add")
- Run the app using the following command:
npm start
- From browser, if we run http://localhost:3000/todos/new, we can view the New todo page as follows:

- The next step is to write the method that will be executed once the
add-todo
form is submitted in routes/todos.js file. Add the following router method:
/* Add To-do to database*/
router.post('/add-todo', function(req, res) {
// Get the only one db instance in our app
var db = req.db;
// Get POST values, It's easy
var title = req.body.title;
var dueDate = req.body.dueDate;
console.log('POST VALUES: ' + title + ' ' + dueDate);
// Fetch from 'users' collection
var todoCollection = db.get("todos");
todoCollection.insert({
'title' : title,
'dueDate' : dueDate
}, function(err, doc) {
if(err) res.send('Problem occured when inserting in todo collection');
else {
console.log("Inserted");
res.location('todos');
res.redirect('/todos');
}
});
});
- Now submitting the form will redirect to /todos/add-todo route and make a
POST
request. The todo
will be saved in the database.
Hopefully, this MEAN Stack tutorial will serve as your first RESTful API using ExpressJS with MongoDB. We will come with a more detailed technical tutorial on related technologies soon. Keep in touch. 
Top Related Technical Articles
The post 8 Steps to develop RESTful API using Express and MongoDB appeared first on Web Development Tutorial.