In this project, we will take a look at MFC list control for MFC applications. After seeing a list of the main features of the IListEx, we will learn about installation and creation, tooltips, menu and sorting. We will also learn about the public methods, structures and notification messages. Finally, we will see an example.
List control for MFC applications
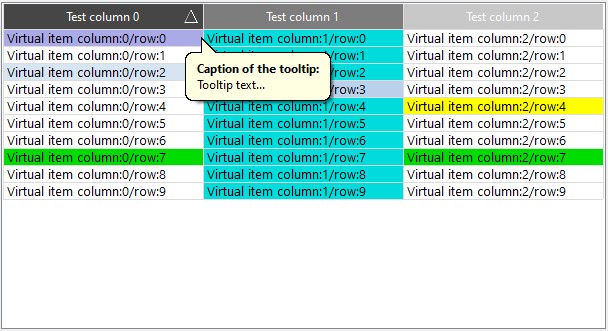
Table of Contents
Introduction
IListEx
is an owner-draw extension of the MFC CMFCListCtrl
class with many features:
Installation
The usage of the control is simple:
- Add ListEx.h and CListEx into your project
- Declare
IListExPtr
variable: IListExPtr myList { CreateListEx() };
IListExPtr
is a pointer to the IListEx
class, wrapped in std::unique_ptr
. This wrapper is used mainly for convenience, so you don't have to bother about object lifetime, it will be destroyed automatically. That's why there is a call to the factory function CreateListEx()
, to properly initialize a pointer.
Control uses its own namespace LISTEX
. So it's up to you, whether to use namespace prefix before declarations:
LISTEX::
or to define namespace in the source file's beginning:
using namespace LISTEX;
Create
Manually
Create
is the main method to create list control. It takes LISTEXCREATE
structure as argument.
Below is a simple example of the control's creation:
IListExPtr myList { CreateListEx() };
.
.
LISTEXCREATE lcs;
lcs.pParent = this;
lcs.uID = ID_MY_LIST;
lcs.rect = CRect(0, 0, 500, 300);
myList->Create(lcs);
In Dialog
To create the control in a Dialog you can manually do it with the Create method.
But most of the times you prefer to place a standard List Control onto the Dialog's template, by dragging it from the Toolbox within Visual studio.
To use the latter approach follow these steps:
- Put standard List Control from the toolbox onto your dialog template. Give it appropriate ID (
IDC_LISTEX
) and make it desirable size. - Declare
IListExPtr
member varable within your dialog class: IListExPtr m_myList { CreateListEx() };
- In your
OnInitDialog
method call m_myList->CreateDialogCtrl(IDC_LISTEX, this);
function.
To set a tooltip for a given cell, just write:
myList->SetCellTooltip(0, 1, L"Tooltip text", L"Tooltip caption:");
This will set a tooltip for cell (0, 1) with the text: Tooltip text, and the caption Tooltip caption.
Sorting
To enable sorting set the LISTEXCREATE::fSortable
flag to true. In this case, when you click on the header, list will be sorted according to the clicked column. By default IListEx
performs lexicographical sorting.
To set your own sorting routine use SetSortable
method.
Editing Cells
By default list control works in the read-only mode. To enable cells editing call the SetColumnEditable
method with the column ID which cells you wish to become editable.
Data Alignment
Classical MFC list control allows setting alignment only for header and column text simultaneously.
ListEx allows setting alignment separately for header and for data respectively. The iDataAlign
argument in the InsertColumn()
method is responsible exactly for that.
Public Methods
IListEx
class also has a set of additional public methods to help customize your control in many different aspects.
HideColumn
void HideColumn(int iIndex, bool fHide);
Hide or show column by iIndex
.
SetCellColor
SetCellColor(int iItem, int iSubitem, COLORREF clrBk, COLORREF clrText = -1);
Sets background and text color for a given cell.
SetCellData
void SetCellData(int iItem, int iSubitem, ULONGLONG ullData);
Sets arbitrary application-defined data associated with given cell.
void SetCellTooltip(int iItem, int iSubitem, std::wstring_view wstrTooltip, std::wstring_view wstrCaption = L"");
Sets tooltip and caption for a given cell.
SetColumnEditable
void SetColumnEditable(int iColumn, bool fEditable);
Enables or disables edit mode for a given column.
SetHdrColumnIcon
void SetHdrColumnIcon(int iColumn, int iIconIndex, bool fClick = false);
Sets the icon index in the header's image list for a given iColumn
. To remove icon from column set the iIconIndex
to -1
.
Flag fClick
means that icon is clickable. See LISTEX_MSG_HDRICONCLICK
message for more info.
SetHdrHeight
void SetHdrHeight(DWORD dwHeight);
SetSortable
void SetSortable(bool fSortable, PFNLVCOMPARE pfnCompare = nullptr, EListExSortMode enSortMode = EListExSortMode::SORT_LEX)
Parameters:
bool fSortable
Enables or disables sorting
PFNLVCOMPARE pfnCompare
Callback function pointer with type int (CALLBACK *PFNLVCOMPARE)(LPARAM lParam1, LPARAM lParam2, LPARAM lParamSort)
that is used to set your own comparison function. If it's nullptr
IListEx
performs default sorting.
The comparison function must be either a static member of a class or a stand-alone function that is not a member of any class. For more information see official MSDN documentation.
EListExSortMode enSortMode
Default sorting mode for the list.
Structures
LISTEXCREATE
struct LISTEXCREATE {
LISTEXCOLORS stColor { }; CRect rect; CWnd* pParent { }; LOGFONTW* pListLogFont { }; LOGFONTW* pHdrLogFont { }; UINT uID { }; DWORD dwStyle { }; DWORD dwListGridWidth { 1 }; DWORD dwHdrHeight { }; bool fDialogCtrl { false }; bool fSortable { false }; bool fLinkUnderline { true }; bool fTooltipBaloon { true }; bool fLinkTooltip { true }; bool fHighLatency { false }; };
LISTEXCOLORS
struct LISTEXCOLORS
{
COLORREF clrListText { GetSysColor(COLOR_WINDOWTEXT) }; COLORREF clrListTextLink { RGB(0, 0, 200) }; COLORREF clrListTextSel { GetSysColor(COLOR_HIGHLIGHTTEXT) }; COLORREF clrListTextLinkSel { RGB(250, 250, 250) }; COLORREF clrListTextCellTt { GetSysColor(COLOR_WINDOWTEXT) }; COLORREF clrListBkRow1 { GetSysColor(COLOR_WINDOW) }; COLORREF clrListBkRow2 { GetSysColor(COLOR_WINDOW) }; COLORREF clrListBkSel { GetSysColor(COLOR_HIGHLIGHT) }; COLORREF clrListBkCellTt { RGB(170, 170, 230) }; COLORREF clrListGrid { RGB(220, 220, 220) }; COLORREF clrTooltipText { GetSysColor(COLOR_INFOTEXT) }; COLORREF clrTooltipBk { GetSysColor(COLOR_INFOBK) }; COLORREF clrHdrText { GetSysColor(COLOR_WINDOWTEXT) }; COLORREF clrHdrBk { GetSysColor(COLOR_WINDOW) }; COLORREF clrHdrHglInact { GetSysColor(COLOR_GRADIENTINACTIVECAPTION) }; COLORREF clrHdrHglAct { GetSysColor(COLOR_GRADIENTACTIVECAPTION) }; COLORREF clrNWABk { GetSysColor(COLOR_WINDOW) }; };
This struct is also used in SetColor
method.
LISTEXCOLOR
struct LISTEXCOLOR
{
COLORREF clrBk { };
COLORREF clrText { };
};
using PLISTEXCOLOR = LISTEXCOLOR*;
LISTEXHDRICON
struct LISTEXHDRICON
{
POINT pt { }; int iIconIndex { }; bool fClickable { true }; };
EListExSortMode
Enum showing sorting type for list columns.
enum class EListExSortMode : short
{
SORT_LEX, SORT_NUMERIC
};
Notification Messages
These messages are sent to the parent window in form of WM_NOTIFY
windows message.
The lParam
will contain pointer to NMHDR
standard windows struct. NMHDR::code
can be one of the LISTEX_MSG_...
messages described below.
LISTEX_MSG_GETCOLOR
When in virtual mode, sent to the parent window to retrieve cell's color. Expects pointer to the LISTEXCOLOR
struct in response, or nothing to use defaults.
void CListDlg::OnListExGetColor(NMHDR* pNMHDR, LRESULT* )
{
const auto pNMI = reintepret_cast<NMITEMACTIVATE*>(pNMHDR);
if (pNMI->iSubItem == 1)
{
static LISTEXCOLOR clr { RGB(0, 220, 220), RGB(0, 0, 0) };
pNMI->lParam = reinterpret_cast<LPARAM>(&clr);
}
}
LISTEX_MSG_GETICON
void CListDlg::OnListExGetIcon(NMHDR* pNMHDR, LRESULT* )
{
const auto pNMI = reinterpret_cast<NMITEMACTIVATE*>(pNMHDR);
...
pNMI->lParam = SomeIndex; }
This message is used in Virtual List mode to obtain icon index in list image list.
LISTEX_MSG_LINKCLICK
List embedded hyperlink has been clicked. WM_NOTIFY
lParam
will point to the NMITEMACTIVATE
struct.
NMITEMACTIVATE::lParam
will contain wchar_t*
pointer to the link
text of the clicked hyperlink. The iItem
and iSubItem
members will contain indexes of the list item/subitem the link was clicked at.
Hyperlink syntax is: L"Text with the <link="any_text_here" title="Optional tool-tip text">embedded link</link>"
If no optional title
tag is provided then the link
text itself will be used as hyperlink's tool-tip.
Link and title text must be quoted ""
.
LISTEX_MSG_HDRICONCLICK
Header icon that previously was set by SetHdrColumnIcon
call has been clicked.
Example code for handling this message:
BOOL CMyDlg::OnNotify(WPARAM wParam, LPARAM lParam, LRESULT* pResult)
{
const auto pNMI = reinterpret_cast<LPNMITEMACTIVATE>(lParam);
if (pNMI->hdr.code == LISTEX_MSG_HDRICONCLICK && pNMI->hdr.idFrom == IDC_MYLIST)
{
const auto pNMI = reinterpret_cast<NMHEADERW*>(lParam);
}
...
LISTEX_MSG_EDITBEGIN
Sent when edit box for data editing is about to show up. If you don't want it to show up, you can set lParam
to 0
in response.
BOOL CMyDlg::OnNotify(WPARAM wParam, LPARAM lParam, LRESULT* pResult)
{
const auto pNMI = reinterpret_cast<LPNMITEMACTIVATE>(lParam);
pNMI->lParam = 0; ...
LISTEX_MSG_DATACHANGED
Sent in Virtual mode when cell's text has changed.
BOOL CMyDlg::OnNotify(WPARAM wParam, LPARAM lParam, LRESULT* pResult)
{
const auto pNMI = reinterpret_cast<LPNMITEMACTIVATE>(lParam);
const auto iItem = pNMI->iItem;
const auto iSubItem = pNMI->iSubItem;
const pwszNewText = reinterpret_cast<LPCWSTR>(pNMI->lParam);
...
Example
Let’s imagine that you need a list control with a non standard header height, and yellow background color. Nothing is simpler, see the code below:
LISTEXCREATE lcs;
lcs.rect = CRect(0, 0, 500, 300)
lcs.pParent = this;
lcs.dwHdrHeight = 50;
lcs.stColor.clrListBkRow1 = RGB(255, 255, 0);
lcs.stColor.clrListBkRow2 = RGB(255, 255, 0);
myList->Create(lcs);
myList->InsertColumn(...);
myList->InsertItem(...);
Here, we set both - even and odd rows (clrListBkRow1
and clrListBkRow2
) to the same yellow color.
Appearance
With the Ctrl+MouseWheel combination you can dynamically change list's font size.