Introduction
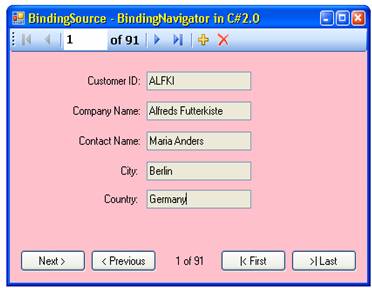
In this simple article, I'd like to show how to use the new components BindingSource
and BindingNavigator
in C# 2.0.
The BindingSource
offers the easiest way to navigate through records in a data source. And it is designed to simplify the process of binding controls to an underlying data source. Whereas a BindingNavigator
is paired mostly with a BindingSource
control to move through data records on a form and interact with them. To run the application and see it in action, I added the Northwind database as installNorthwind.sql containing only the Customers table.
You can not find the Northwind and Pubs sample databases in Microsoft SQL Server 2005 because they are not installed by default. But you can download these databases from the Microsoft web site.
What is BindingSource?
The new component BindingSource
in C# 2.0 is the replacement for CurrencyManager
. Although you can still use the CurrencyManager
, the BindingSource
component is the preferred way to bind your Windows Forms controls to data sources.
It supports the bindung of control elements in a form, and can be seen as a link between a data source and a control element. It provides advantages over traditional data binding such as:
- Encapsulates the
CurrencyManager
functionality, and exposes the CurrencyManager
events at design time.
- Interoperates with other data-related Windows Forms controls, i.e., the
BindingNavigator
and the DataGridView
controls.
The default property for the BindingSource
class is DataSource
. The default event is CurrentChanged
. The BindingSource
provides a number of methods, properties, and events such as CurrentItemChanged
and DataSourceChanged
, that allow for customization. The Position
property gets or sets the index of the current item in the underlying list. The MoveLast()
method changes the current value of the Position
property to the index of the last item in the underlying data source, which is equal to the value of the Count
property minus 1.
For example:
this.customersDataGridView1.DataSource = this.customersBindingSource1;
or
int count = this.flagsBindingSource.Count;
or
int pos = this.customersBindingSource1.Position;
or
this.bindingSource1.Position = this.bindingSource1.Count - 1;
More on: Microsoft MSDN.
Data Binding With »BindingSource« in a Windows Application
If you want to navigate through records in a data source as mentioned before, the BindingSource
offers the better and easiest way to do this. You can bind a BindingSource
component to the data source, and then bind, e.g., TextBox
controls to the BindingSource
as we will see later. We can then use the built-in navigation methods on the BindingSource
such as MoveNext()
, MovePrevious()
, MoveFirst()
, and MoveLast()
.
The easiest way to see the BindingSource
and BindingNavigator
in action is as follows: choose the menu options: Data-->Show Data Source-->Add New Data Source. After you establish the connection, choose a DataSet
, and select a table, you will see in the Data Source window the table you chose. From there, now you can drag/drop the table onto the Windows Form, and you get the data from the dragged table on a DataGridView
including the BindingSource
, DataSet
, TableAdapter
, and BindingSource
automatically.
But we want to go another way which I think is the better way to understand and see how BindingSource
and BindingNavigator
work together.
First drag/drop five TextBox
es, six Label
s, four Button
s, and a BindingSource
from the Toolbox onto the form.

Now we have to adjust the two properties for the objects to find the data source clearly, which are:
After setting the DataSource
in the Properties window, click "Add Project Data Source..."

In the next dialog window, you choose the database, and click Next.

Through the Next button, you come to a new dialog. Here you search for an available database connection, or you create a new connection.

After you click the Next button, you will get the following dialog where you choose your database object, in other words: you choose here the table "Customer" and tick it, and click the Finish button.

Now, you have created and added, as you can see, a new typed DataSet
"northwindDataSet
". Mark the DataMember
in the Properties window, and click the "Customers" table. Now, an of TableAdapter
is created. A TableAdapter
is similar to a DataAdapter
, but related to a DataTable
. For example: if it describes the table "Customers", then the name of the TableAdapter
is customersTableAdapter
; for the table "Authors", the name would be authorsTableAdapter
etc.
Now you have to combine all the control elements (TextBox
es) with the BindingSource
object. You can do this in the Properties window entry "DataBinding
" of each TextBox
.

You mark textBoxCustId
and extend the "DataBinding
" entry in the Properties window. Click Text
, and then choose here the column "CustomerID
". Combine textBoxCompanyName
with the column "CompanyName
" and so on.
At this point, we have completed binding all the TextBox
controls to the BindingSource
.
Navigation methods of the BindingSource
MoveNext(), MovePrevious(), MoveFirst(), and MoveLast()
Here are the event handlers for the Click
events of the buttons (Next, Previous, First, Last) for navigation.
Next button:
private void btNext_Click(object sender, EventArgs e)
{
if (this.bindingSource1.Position + 1 < this.bindingSource1.Count)
{
this.bindingSource1.MoveNext();
this.fnDisplayPosition();
}
}
Previous button:
private void btPrevious_Click(object sender, EventArgs e)
{
this.bindingSource1.MovePrevious();
this.fnDisplayPosition();
}
First button:
private void btFirst_Click(object sender, EventArgs e)
{
this.bindingSource1.MoveFirst();
this.fnDisplayPosition();
}
Last button:
private void btLast_Click(object sender, EventArgs e)
{
this.bindingSource1.MoveLast();
this.fnDisplayPosition();
}
Display the position of the current record:
private void fnDisplayPosition()
{
this.labelPosition.Text = this.bindingSource1.Position +
1 + " of " + this.bindingSource1.Count;
}
You can run the application, and the four navigation buttons should function now. But we have not implemented the BindingNavigator
yet.
What is BindingNavigator?
The BindingNavigator
control represents a standard way to navigate and manipulate data on a form. It is a special-purpose ToolStrip
control for navigating and manipulating controls on the Windows Form.
In most cases, a BindingNavigator
is combined with a BindingSource
control to move through data records on a Windows Form. It is easy to modify the BindingNavigator
component if you want to add additional or alternative commands for the user.
A BindingNavigator
is:
- a VCR (video-cassette recorder) control which is used with
BindingSource
.
- based on
ToolStrip
.
- extensible and pluggable.
Now, from the Toolbox, drag/drop a BindingNavigator
onto the Form, and the Windows Forms Designer puts it by default on the top automatically.

And at last, that brings us to our final operation. The BindingNavigator
still does not know where the data source is. We have to show the BindingNavigator
where its data source is. Mark bindingNavigator1
on the Form1[Design] and set the BindingNavigator
control's BindingSource
property to the BindingSource
(here: bindingSource1
) on the Form.

Now the BindingNavigator
is ready to do its task as a link between the data source and the control elements.
And..
If you want to dig into the other features of BindingNavigator
and BindingSource
, there are enough resources on the web. And I would particularly recommend the Microsoft MSDN web site.
I wish the article was a bit helpful.
Happy programming.