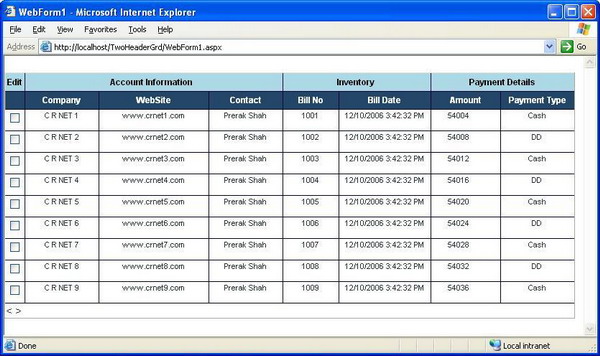
Introduction
This article describes the technique to merge the header of a DataGrid
by modifying the HTML in a tricky way.
Background
Some time ago, the requirement came to provide a common title to many sub-titles; for example, imagine that you want to display records containing account, inventory, and payment information.
- Account information contains company name, website, and contact person.
- Inventory contains bill number and bill date.
- Payment details contain amount and payment type.
Now in this situation, you have to merge different DataGrid
header columns to one common DataGrid
header.
Using the code
To display common headers in DataGrid
, write your code as below. First, put your DataGrid
in a Table
tag, and write your first <asp:TemplateColumn>
in the <Columns>
section like:
<table id="headingtable"
style="FONT-WEIGHT: bold; COLOR: #244668" borderColor="#000033"
height="100%" cellSpacing="0"
cellPadding="0" border="0" runat="server">
<tr>
<td><asp:datagrid id="grdSearchResult" Runat="server"
AllowPaging="True" AutoGenerateColumns="False"
AllowSorting="True" BackColor="white">
<ItemStyle Height="30px" VerticalAlign="Top"
CssClass="GrdItemStyle"></ItemStyle>
<AlternatingItemStyle Height="30px"
CssClass="GrdItemStyle"></AlternatingItemStyle>
<HeaderStyle CssClass="GrdHeaderStyle"></HeaderStyle>
<Columns>
<asp:TemplateColumn ItemStyle-BorderColor="#000033"
ItemStyle-Width="25Px" ItemStyle-Wrap="True"
HeaderStyle-CssClass="GrdExtraHeader">
<HeaderTemplate>Edit
</td>
<td width="500" class="GrdExtraHeader" colspan="3"
align="center">Account Information</td>
<td width="325" class="GrdExtraHeader"
colspan="2" align="center">Inventory</td>
<td width="225" class="GrdExtraHeader" colspan="2"
align="center">Payment Details</td>
</tr>
<td Align="Center" Height="25px" bgcolor="#244668"
class="GrdHeaderStyle" BorderColor="#000033"></td>
</HeaderTemplate>
<ItemTemplate>
<asp:CheckBox id="chkEdit" Runat="server" Width="25">
</asp:CheckBox>
</ItemTemplate>
</asp:TemplateColumn>
Please note that you have to close the td
tag, i.e., </td>
after giving the header template name. And then put the rest of the common header in a new TD
by specifying the colspan
attribute, which contains the total number of columns you want to merge, i.e., <td width="500" class="GrdExtraHeader" colspan="3" align="center">Account Information</td>
. Here Account Information has three sub-headers. Close the row (</tr>
) after the last common header. In the above code, you can find this after "Payment Details". The reason behind closing the TR
tag is that we have to display all sub-headers in the second row. Write the rest of your DataGrid columns code in a standard way. Find the code below.
<asp:TemplateColumn HeaderText="Company" HeaderStyle-CssClass="GrdHeaderStyle"
ItemStyle-Width="100Px" ItemStyle-Wrap="True"
ItemStyle-CssClass="GrdItemStyle">
<ItemTemplate>
<asp:Label ID="lblCompanyName" Width="100px"
Runat="server"
text='<%# DataBinder.Eval(
Container.DataItem,"company_name") %>'>
</asp:Label>
</ItemTemplate>
</asp:TemplateColumn>
<asp:TemplateColumn HeaderText="WebSite"
HeaderStyle-CssClass="GrdHeaderStyle"
ItemStyle-Width="100Px" ItemStyle-Wrap="True"
ItemStyle-CssClass="GrdItemStyle">
<ItemTemplate>
<asp:Label ID="lblWebSiteName" Width="150px"
Runat="server"
text='<%# DataBinder.Eval(
Container.DataItem,"website_name") %>'>
</asp:Label>
</ItemTemplate>
</asp:TemplateColumn>
<asp:TemplateColumn HeaderText="Contact"
HeaderStyle-CssClass="GrdHeaderStyle"
ItemStyle-Width="100Px" ItemStyle-Wrap="True"
ItemStyle-CssClass="GrdItemStyle">
<ItemTemplate>
<asp:Label ID="lblContactPersonName" Width="100px"
Runat="server"
text='<%# DataBinder.Eval(
Container.DataItem,"contact_person_name") %>'>
</asp:Label>
</ItemTemplate>
</asp:TemplateColumn>
<asp:TemplateColumn HeaderText="Bill No"
HeaderStyle-CssClass="GrdHeaderStyle"
ItemStyle-Width="75Px"
ItemStyle-Wrap="True"
ItemStyle-HorizontalAlign="Right"
ItemStyle-CssClass="GrdItemStyle">
<ItemTemplate>
<asp:Label ID="lblBillno" Width="75px"
Runat="server"
text='<%# DataBinder.Eval(
Container.DataItem,"BillNo") %>'>
</asp:Label>
</ItemTemplate>
</asp:TemplateColumn>
<asp:TemplateColumn HeaderText="Bill Date"
HeaderStyle-CssClass="GrdHeaderStyle"
ItemStyle-Width="75Px" ItemStyle-Wrap="True"
ItemStyle-HorizontalAlign="Right"
ItemStyle-CssClass="GrdItemStyle">
<ItemTemplate>
<asp:Label ID="lblBillDate" Width="125px"
Runat="server"
text='<%# DataBinder.Eval(
Container.DataItem,"BillDate") %>'>
</asp:Label>
</ItemTemplate>
</asp:TemplateColumn>
<asp:TemplateColumn HeaderText="Amount"
HeaderStyle-CssClass="GrdHeaderStyle"
ItemStyle-Width="75Px"
ItemStyle-Wrap="True" ItemStyle-CssClass="GrdItemStyle">
<ItemTemplate>
<asp:Label ID="lblamount" Width="75px"
Runat="server"
text='<%# DataBinder.Eval(
Container.DataItem,"Amount") %>'>
</asp:Label>
</ItemTemplate>
</asp:TemplateColumn>
<asp:TemplateColumn HeaderText="Payment Type"
HeaderStyle-CssClass="GrdHeaderStyle"
ItemStyle-CssClass="GrdItemStyle">
<ItemTemplate>
<asp:Label ID="lblPayout" Width="75px" Runat="server"
text='<%# DataBinder.Eval(
Container.DataItem,"payment_type") %>'>
</asp:Label>
</ItemTemplate>
</asp:TemplateColumn>
</columns> </asp:datagrid></TD></TR>
</table>