Introduction
This project shows how to send mail via an SMTP server using C#.
How to Send Mail via SMTP
Based on RFC 821 about Simple Mail Transfer Protocol, it's very easy to send mail via SMTP. Here's the handshaking for sending mail:
Receiver: 220 server.com Simple Mail Transfer Service Ready
Sender : HELO server.com
Receiver: 250 server.com
Sender : MAIL FROM: <agusk@host.com>
Receiver: 250 OK
Sender : RCPT TO: <myhoney@host.com>
Receiver: 250 OK
Sender : DATA
Receiver: 354 Start mail input: end with <CRLF>.<CRLF>
Sender : all data like From, To, Subject, Body etc.
ended with <CRLF>.<CRLF>
Receiver: 250 OK
Sender : QUIT
Receiver: 250 server.com closing transmission channel
Building the Application Using C#
Here's a model of the GUI:
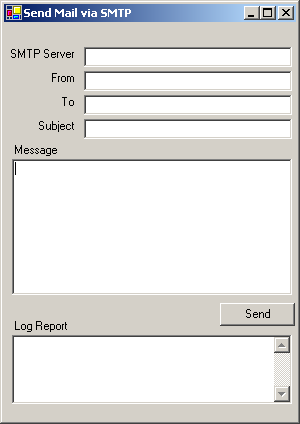
add these code lines for when the use clicks the Send button:
private void SendBtn_Click(object sender, System.EventArgs e)
{
Cursor cr = Cursor.Current;
Cursor.Current = Cursors.WaitCursor;
TcpClient SmtpServ = new TcpClient(ServSMTP.Text,25);
string Data;
byte[] szData;
string CRLF = "\r\n";
LogList.Items.Clear();
try
{
NetworkStream NetStrm = SmtpServ.GetStream();
StreamReader RdStrm= new StreamReader(SmtpServ.GetStream());
LogList.Items.Add(RdStrm.ReadLine());
Data = "HELLO server " + CRLF;
szData = System.Text.Encoding.ASCII.GetBytes(Data.ToCharArray());
NetStrm.Write(szData,0,szData.Length);
LogList.Items.Add(RdStrm.ReadLine());
Data = "MAIL FROM: " + "<" + sFrom.Text + ">" + CRLF;
szData = System.Text.Encoding.ASCII.GetBytes(Data.ToCharArray());
NetStrm.Write(szData,0,szData.Length);
LogList.Items.Add(RdStrm.ReadLine());
Data = "RCPT TO: " + "<" + sTo.Text + ">" + CRLF;
szData = System.Text.Encoding.ASCII.GetBytes(Data.ToCharArray());
NetStrm.Write(szData,0,szData.Length);
LogList.Items.Add(RdStrm.ReadLine());
Data = "DATA " + CRLF;
szData = System.Text.Encoding.ASCII.GetBytes(Data.ToCharArray());
NetStrm.Write(szData,0,szData.Length);
LogList.Items.Add(RdStrm.ReadLine());
Data = "SUBJECT: " + sSubject.Text + CRLF +
sMessage.Text + CRLF + "." + CRLF;
szData = System.Text.Encoding.ASCII.GetBytes(Data.ToCharArray());
NetStrm.Write(szData,0,szData.Length);
LogList.Items.Add(RdStrm.ReadLine());
Data = "QUIT " + CRLF;
szData = System.Text.Encoding.ASCII.GetBytes(Data.ToCharArray());
NetStrm.Write(szData,0,szData.Length);
LogList.Items.Add(RdStrm.ReadLine());
NetStrm.Close();
RdStrm.Close();
LogList.Items.Add("Close connection");
LogList.Items.Add("Send mail successly..");
Cursor.Current = cr;
}
catch(InvalidOperationException err)
{
LogList.Items.Add("Error: "+ err.ToString());
}
}
Reference
- Document RFC 821 and MSDN for .NET Development
He gradueted from Sepuluh Nopember Institute of Technology (ITS) in Department of Electrical Engineering, Indonesia. His programming interest is VC++, C#, VB, VB.NET, .NET, VBScript, Delphi, C++ Builder, Assembly,and ASP/ASP.NET. He's founder for PE College(www.pecollege.net), free video tutorial about programming, infrastructure, and computer science. He's currently based in Depok, Indonesia. His blog is http://geeks.netindonesia.net/blogs/agus and http://blog.aguskurniawan.net