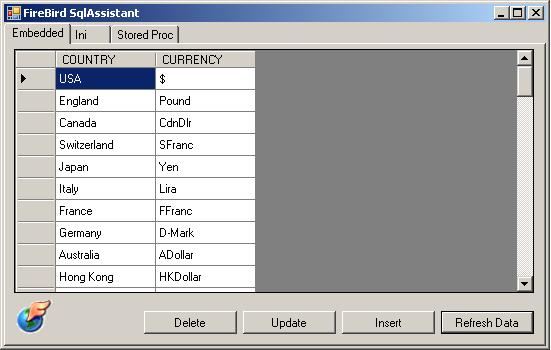



Introduction
In his article,
Data Application Block for Firebird SQL, Alex provided a great library to start working with FireBird. I tried to imporve that library in some ways. You could also take a look at
FireBird SqlHelper - A Data Access Application Block For FireBird to have a data access block similar to Microsoft Data Access Block V2.
Points of Interest
Following features could be of higher interest:
- FireBird.SqlAssistant is completly stateless.
- Small foot print FireBird.SqlAssistant.dll is 24kb.
- Supports quick INSERT, UPDATE, DELETE, SELECT, SELECTALL methods.
- Supports to place long SELECT queries in an INI file 'Query.ini'. This could be helpful in code maintainability in some projects.
- Simplified stored procedure calling by reducing function signature of Alex's DataBlockFirebirdSQL.
- Provides many handy helper functions like GetCount etc.
Using the Code
Following are few sample calls from Demo project available for download. You could simply add the reference of FireBird.SqlAssistant in your project and call
SQLAssistant static
functions.
private void Form1_Load(object sender, EventArgs e)
{
this.textBox1.Text = SQLAssistant.GetIniQuery("MyQuery1");
this.textBox2.Text = SQLAssistant.GetIniQuery("MyQuery2");
}
private void button1_Click(object sender, EventArgs e)
{
this.RefreshData();
}
private void RefreshData()
{
this.dataGridView1.DataSource = SQLAssistant.Execute("SELECT * FROM COUNTRY").Tables[0];
}
private void button2_Click(object sender, EventArgs e)
{
string c = SQLAssistant.GetCount("COUNTRY").ToString();
string v1 = "ACountry" + c;
string v2 = "ACurr" + c;
SQLAssistant.Insert("COUNTRY", "COUNTRY", v1, "CURRENCY", v2);
this.RefreshData();
MessageBox.Show("COUNTRY='" + v1 + "' added successfully");
}
private void button3_Click(object sender, EventArgs e)
{
DataTable table = SQLAssistant.Select("COUNTRY", "COUNTRY", "USA").Tables[0];
if (table == null || table.Rows.Count == 0)
{
MessageBox.Show("COUNTRY='USA' not found");
return;
}
DataRow row = table.Rows[0];
row["CURRENCY"] = "$";
SQLAssistant.Update("COUNTRY", table.Rows[0], "COUNTRY", "USA");
MessageBox.Show("CURRENCY='Dollar' is successfully updated to '$'");
this.RefreshData();
}
private void button4_Click(object sender, EventArgs e)
{
SQLAssistant.Insert("COUNTRY", "COUNTRY", "TestCountry", "CURRENCY", "None");
DataTable table = SQLAssistant.Select("COUNTRY", "COUNTRY", "TestCountry").Tables[0];
if (table == null || table.Rows.Count == 0)
{
MessageBox.Show("COUNTRY='TestCountry' not found");
return;
}
DataRow row = table.Rows[0];
SQLAssistant.Delete("COUNTRY", row);
MessageBox.Show("COUNTRY='TestCountry' is deleted successfully");
this.RefreshData();
}
private void button6_Click(object sender, EventArgs e)
{
this.dataGridView2.DataSource = SQLAssistant.ExecuteIni("MyQuery1").Tables[0];
}
private void button5_Click(object sender, EventArgs e)
{
this.dataGridView2.DataSource = SQLAssistant.ExecuteIni("MyQuery2").Tables[0];
}
private void button7_Click(object sender, EventArgs e)
{
this.dataGridView3.DataSource = SQLAssistant.ExecuteDataSet("ORG_CHART ", null).Tables[0];
}