Introduction
This article discusses how to create an order page and shopping cart page using GridView ASP.NET C# and SQL Server
- Create three tables:
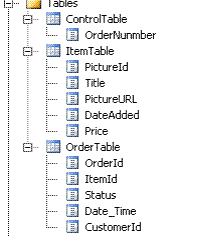
- Create a web Project
- Create an Images Folder in the project solution
- Add some image file into the Images folder

- Rename the Default.aspx web page to OrderPage.aspx
- Drag and drop
GridView
object from the toolbox on to the web form. - Create the following
GridView
Columns and set the GridView AutoGenerateColumn
to false
. 
- Where
AddToCart
is a button, Picture ID, Title and Date Added are text fields and PictureURL
is Image field. GridView
will look like this:

- When the order page is loaded, all the items have to be loaded to the
GridView
for the user to select, copy the following code to the load page event:
string Sel = "Select * from ItemTable";
SqlConnection Con = new SqlConnection(Cn);
SqlCommand cmd = new SqlCommand(Sel, Con);
Con.Open();
DataTable dt = new DataTable();
dt.Columns.Add(new DataColumn("PictureID", typeof(int)));
dt.Columns.Add(new DataColumn("PictureURL", typeof(string)));
dt.Columns.Add(new DataColumn("Title", typeof(string)));
dt.Columns.Add(new DataColumn("DateAdded", typeof(DateTime)));
SqlDataReader reader = cmd.ExecuteReader();
while (reader.Read())
{
DataRow dr = dt.NewRow();
dr["PictureID"] = Convert.ToInt32(reader["PictureId"]);
dr["PictureURL"] = ResolveUrl("~/Images/" +reader["PictureURL"]);
dr["Title"] = reader["Title"];
dr["DateAdded"] = reader["DateAdded"];
dt.Rows.Add(dr);
}
Con.Close();
GridView1.DataSource = dt;
GridView1.DataBind();
Where Cn Is the connection string.
- Enter some data to the SQL table:


- Build and run the application.
- The following result will be displayed:

AddToCart
button click event:

Set the AddToCart
button property.
- Add this to the
GridView
property using the source page or the HTML page
OnRowCommand="GridView1_RowCommand"
- Add these lines of code to the page code behind:
protected void GridView1_RowCommand(object sender, GridViewCommandEventArgs e)
{
if (e.CommandName == "AddToCart")
{
int index = Convert.ToInt32(e.CommandArgument);
GridViewRow row = GridView1.Rows[index];
AddShopCart(row.Cells[1].Text.ToString());
}
}
- After you click the
AddToCart
button, you need to move the item to the shopping cart. To do that, you need to write code to make a data entry to OrderTable
, since ItemId
is unique. Based on ItemId
we insert a data entry to the OrderTable
. - Before we make an entry to the table we need order Number, we can get the order number from
ControlTable
since ControlTable
holds the last used order number. - The method to insert selected item to shopping cart will get the last used order number from
ControlTable
, and after inserting the values to the OrderTable
will update the order number in the ControlTable
.
private void AddShopCart(string ItemId)
{
string ord = OrderNumber();
if (ord != "Bad order")
{
int ordernumber = Convert.ToInt32(ord);
ordernumber += 1;
SqlConnection C_n = new SqlConnection(Cn);
SqlCommand cm = new SqlCommand("Insert INTO OrderTable VALUES
('" + ordernumber + "', '" + ItemId + "', '" + "101" +
"', '" + Convert.ToDateTime("2/19/2007") + "','" + "1" + "')", C_n);
C_n.Open();
SqlDataReader dr = cm.ExecuteReader();
C_n.Close();
UpdateOrderNumber(ordernumber);
}
}
- The method to get the last used order number.
private string OrderNumber()
{
SqlConnection Or_Cn = new SqlConnection(Cn);
SqlCommand Or_Cm = new SqlCommand("Select OrderNumber from ControlTable", Or_Cn);
Or_Cn.Open();
SqlDataReader Or_rd = Or_Cm.ExecuteReader();
if (Or_rd.Read())
{
return Or_rd["OrderNumber"].ToString();
}
else
{
return "Bad order";
}
}
- The method to update the order number in the
ControlTable
:
private void UpdateOrderNumber(int UpdatedNumber)
{
SqlConnection Op_Cn = new SqlConnection(Cn);
SqlCommand Op_Cm = new SqlCommand
("Update ControlTable Set OrderNumber=" + UpdatedNumber, Op_Cn);
Op_Cn.Open();
SqlDataReader Op_rd = Op_Cm.ExecuteReader();
Op_Cn.Close();
}
- Add new page to the project and name it ShoppingCart.aspx.
- Drag and drop
GridView
object from the toolbox on to the web form. - Create the following
GridView
columns and set the GridView AutoGenerateColumn
to false
.

- Where Delete is a button, Picture ID, Title, Price and Date Added are text fields and PictureURL is Image field.
GridView
will look like this:

- Under page load event, copy the following code:
string Sel = "Select a.* from ItemTable as a INNER JOIN
OrderTable as b ON a.PictureId=b.ItemId";
SqlConnection Con = new SqlConnection(Cn);
SqlCommand cmd = new SqlCommand(Sel, Con);
Con.Open();
DataTable dt = new DataTable();
dt.Columns.Add(new DataColumn("PictureID", typeof(int)));
dt.Columns.Add(new DataColumn("Title", typeof(string)));
dt.Columns.Add(new DataColumn("Price", typeof(string)));
dt.Columns.Add(new DataColumn("DateAdded", typeof(DateTime)));
dt.Columns.Add(new DataColumn("PictureURL", typeof(string)));
SqlDataReader reader = cmd.ExecuteReader();
while (reader.Read())
{
DataRow dr = dt.NewRow();
dr["PictureID"] = Convert.ToInt32(reader["PictureId"]);
dr["Title"] = reader["Title"];
dr["Price"] = reader["Price"];
dr["DateAdded"] = reader["DateAdded"];
dr["PictureURL"] = ResolveUrl("~/Images/" + reader["PictureURL"]);
dt.Rows.Add(dr);
}
Con.Close();
GridView1.DataSource = dt;
GridView1.DataBind();
- Build and run. After placing some order, make the shopping cart startup page and you will see all the orders you have placed listed there.