Summary
This is a simple example showing how to implement encrypted communication between Windows Phone 7 and standalone .NET application.
Introduction
The example below implements a simple .NET application as a service and a Windows Phone 7 application as a client. The service counts the number of words in the given text. The client then uses the service to get amount of words in the text and displays the result.
The communication between the client and the service is protected by AES (Advanced Encryption Standard).
Also, since the network connection does not have to be stable (e.g. weak signal), the example uses the buffered messaging. The buffered messaging detects disconnections, automatically tries to reconnect and meanwhile stores sent messages in the buffer. Then, when the connection is available again, the messages stored in the buffer are sent to the receiver.
The example is based on the Eneter Messaging Framework.
(The framework is free and can be downloaded from http://www.eneter.net. The online help for developers can be found at http://www.eneter.net/OnlineHelp/EneterMessagingFramework/Index.html.)
Service Application
The service is a standalone .NET application and is responsible for the receiving of messages and counting words in the given text.
Please notice, to run the HTTP listening application, you must execute it under sufficient user rights. Otherwise the application will run, but will not listen. (The framework logs the Access denied error on the debug port.)
The whole implementation is very simple.
using System;
using System.Text.RegularExpressions;
using Eneter.Messaging.DataProcessing.Serializing;
using Eneter.Messaging.EndPoints.TypedMessages;
using Eneter.Messaging.MessagingSystems.Composites;
using Eneter.Messaging.MessagingSystems.HttpMessagingSystem;
using Eneter.Messaging.MessagingSystems.MessagingSystemBase;
namespace EncryptedService
{
class Program
{
private static IDuplexTypedMessageReceiver<int, string> myReceiver;
static void Main(string[] args)
{
IMessagingSystemFactory anUnderlyingMessaging =
new HttpMessagingSystemFactory();
IMessagingSystemFactory aBufferedMessaging =
new BufferedMonitoredMessagingFactory(
anUnderlyingMessaging,
new XmlStringSerializer(),
TimeSpan.FromMilliseconds(15000), TimeSpan.FromMilliseconds(500), TimeSpan.FromMilliseconds(5000) );
IDuplexInputChannel anInputChannel =
aBufferedMessaging.CreateDuplexInputChannel
("http://127.0.0.1:8035/MyService/");
anInputChannel.MessageReceived += OnEncryptedMessageReceived;
AesSerializer aSerializer = new AesSerializer("My secret password");
IDuplexTypedMessagesFactory aSenderFactory =
new DuplexTypedMessagesFactory(aSerializer);
myReceiver =
aSenderFactory.CreateDuplexTypedMessageReceiver<int, string>();
myReceiver.MessageReceived += OnMessageReceived;
Console.WriteLine("The service is running.");
Console.WriteLine("Press enter to stop ...");
myReceiver.AttachDuplexInputChannel(anInputChannel);
Console.ReadLine();
myReceiver.DetachDuplexInputChannel();
}
private static void OnMessageReceived
(object sender, TypedRequestReceivedEventArgs<string> e)
{
if (e.ReceivingError == null)
{
Console.WriteLine("Received message: {0}\n", e.RequestMessage);
MatchCollection aWords = Regex.Matches(e.RequestMessage, @"[\S]+");
int aNumberOfWords = aWords.Count;
myReceiver.SendResponseMessage(e.ResponseReceiverId, aNumberOfWords);
}
}
private static void OnEncryptedMessageReceived
(object sender, DuplexChannelMessageEventArgs e)
{
string anEncryptedMessage = "";
byte[] anEncryptedArray = e.Message as byte[];
foreach (byte aByte in anEncryptedArray)
{
anEncryptedMessage += aByte.ToString("x2");
}
Console.WriteLine("Encrypted Message: {0}", anEncryptedMessage);
}
}
}
Client Application
The client is a Windows Phone 7 application and is responsible for receiving text from the user and using the service to get the number of words in the given text. Then it displays the result.
(Notice, if you run the application from the Visual Studio, do not forget to set that the application shall be deployed on 'Windows Phone 7 Emulator'.)
The client application uses the assembly built for the Windows Phone 7, Eneter.Messaging.Framework.Phone.dll.
using System;
using System.Windows;
using Eneter.Messaging.DataProcessing.Serializing;
using Eneter.Messaging.EndPoints.TypedMessages;
using Eneter.Messaging.MessagingSystems.Composites;
using Eneter.Messaging.MessagingSystems.HttpMessagingSystem;
using Eneter.Messaging.MessagingSystems.MessagingSystemBase;
using Microsoft.Phone.Controls;
namespace EncryptedClientWP7
{
public partial class MainPage : PhoneApplicationPage
{
private IDuplexTypedMessageSender<int, string> mySender;
public MainPage()
{
InitializeComponent();
OpenConnection();
}
private void OpenConnection()
{
IMessagingSystemFactory anUnderlyingMessaging =
new HttpMessagingSystemFactory();
IMessagingSystemFactory aBufferedMessaging =
new BufferedMonitoredMessagingFactory(
anUnderlyingMessaging,
new XmlStringSerializer(),
TimeSpan.FromMinutes(1), TimeSpan.FromMilliseconds(500), TimeSpan.FromMilliseconds(1000) );
IDuplexOutputChannel anOutputChannel =
aBufferedMessaging.CreateDuplexOutputChannel
("http://127.0.0.1:8035/MyService/");
AesSerializer aSerializer = new AesSerializer("My secret password");
IDuplexTypedMessagesFactory aSenderFactory =
new DuplexTypedMessagesFactory(aSerializer);
mySender = aSenderFactory.CreateDuplexTypedMessageSender<int, string>();
mySender.ResponseReceived += OnResponseReceived;
mySender.AttachDuplexOutputChannel(anOutputChannel);
}
private void PhoneApplicationPage_Unloaded(object sender, RoutedEventArgs e)
{
mySender.DetachDuplexOutputChannel();
}
private void SendButton_Click(object sender, RoutedEventArgs e)
{
mySender.SendRequestMessage(TextMessage.Text);
}
private void OnResponseReceived
(object sender, TypedResponseReceivedEventArgs<int> e)
{
if (e.ReceivingError == null)
{
Result.Text = e.ResponseMessage.ToString();
}
}
}
}
And the communicating applications are displayed here:
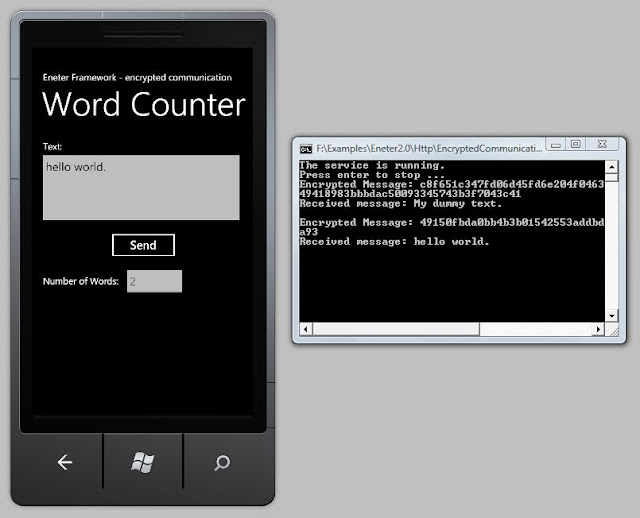