Introduction
This article describes how to implement the functionality of saving changes on browser close or navigating away from the current web application. It also discusses the various ways that the user can exit a page and how to save data on those events.
Background
Many have been trying to implement the functionality of saving data on closing the browser. I was also one of them trying to find a way to implement the same. I had been to many forums and discussion boards, but couldn't find a way to implement this. I finally landed on a piece of JavaScript code (attached below) to trap the close event of an IE window, which actually helped me in implementing this functionality.
Using the Code
Navigation in a site can happen in two ways. Following is a list of actions by which users can navigate:
- Navigating to pages using controls provided within the site.
- Navigating to pages using browser controls, shortcut keys, or right click options.
Following are ways through which the user can navigate away from a page. All these are covered in this solution.
Navigation or postback using controls available in the application:
- Image buttons
- Link buttons
- Submit buttons
- Server controls with the "Autopostback" option set to true
Navigation through user actions, browser buttons, shortcut keys, closing the browser. Following is a list of ways by which this is achieved:
- Back button on the browser Toolbar
- Refresh button on the browser Toolbar
- Close button on the browser Toolbar
- Home button on the browser Toolbar
- ALT + F4 to close the browser
- CTRL + F4 (for tabbed browsers)
- F5 or CTRL + R or Right click for refresh
- ALT + Home button (to navigate to client's Home page)
- Click backspace (alternative for Back button)
- Typing a URL and navigating to a different page
- Selecting a URL from the Favorites button
- Right click and select Refresh
Identifying the different actions and enabling save for each action is a tedious process. The save functionality can be implemented in a simpler way using the JavaScript onbeforeunload
event.
Reasons to use onbeforeunload
The onbeforeunload
event (which is called before the unload event) is called when:
- Navigating to another page (as mentioned above)
- Postback due to a control event
- Using controls on the browser
- Closing the page
Thus, onbeforeunload
is the appropriate event at which the save functionality can be implemented.
Implementation of the Save functionality
To differentiate between a user initiated event and a control initiated event, an attribute named tag
is added with a value "DonotCallSaveonLoad
" to all the known controls in the application, using which the Save
method can be called without prompting the user to save the page.
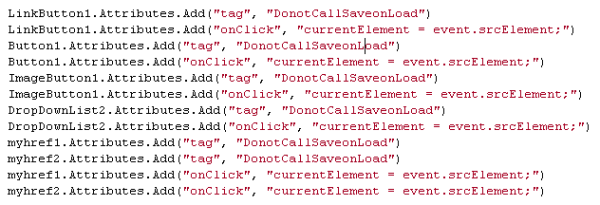
event.srcElement
will set the object of the control to the variable currentElement
which initiated the postback. This will be set on the onClick
event of the control.
Note: The onClick
event is the only point where the Control object can be retrieved because the object of the control is destroyed when the process reaches the onbeforeUnload
event.
The onbeforeUnload
event calls the HandleOnClose
function which checks if the variable currentElement
has a value or not.

The JavaScript function HandleOnClose
checks for this attribute tag of the currentElement
object. If currentElement
is null or the value of the tag
attribute of currentElement
is not DonotCallSaveonLoad
, then it calls the function FunctiontoCallSaveData
; otherwise, the save functionality on the code-behind is called.

The implementation of the function FunctiontoCallSaveData
is the same as previously sent, which is as follows:

form.submit
will in turn call Page_Load
in which the Save functionality can be called. Error handling can also be performed for this functionality. The same is shown in the code snippet below:

Points to Note
This functionality cannot be debugged using breakpoints. This functionality can be verified by checking if the data has been updated in the data source. In the attached demo, there is a SQL script which creates a temporary table to which data is stored on the close of the browser. Please make necessary changes to the function SaveDataonPage
on the .vb file as per your convenience. Exception Handling is implemented using Enterprise Library 2.0. Customizations can be done to its Exception Handling mechanism also.
A highly experienced and influential Technical Manager / Team Leader and Architect, an inspirational leader who has effectively managed, developed and motivated internal and external teams. Strong ability to quickly understand and analyze business strategies ensuring technology solutions meet business requirement, to quickly adapt to all new technologies and challenges.
My attention to detail, high technical aptitude and hands on approach together with a high degree of motivation and professionalism would make me an asset to any progressive organization or demanding position within IT industry.
What do I do?
I work at Spozzle(www.spozzle.me) as a Software Architect and currently working on the following technologies.
Web Technologies using
Java
PHP
XCode 4 and IPhone Development
HTML 5 and CSS
Android Development
My Experience
I have spent my last 7 years working on .NET Technology building application both on the Web and Windows.
I provide Technical consultations to people on
- .NET Development, Best Practices and Issues
- Best Practices on Web based Architecture and Hosting applications on Cloud.
I have not moved on to PHP, Java and Mobile Development. I now work at Spozzle developing really cool application for the Sports Fanatics on Facebook. Checkout http://apps.facebook.com/spozzle