Recently, I had a task wherein I had to create PDF dynamically by merging data and template. I have been using lots of PDF libraries since I started programming to do such tasks. The previous one I admired a lot was Jasper Reports. But after researching for some more time, I found out that IText with Openoffice Draw is the simplest way we can generate PDF forms.
This visual tutorial first explains how to create PDF forms in Openoffice Draw:

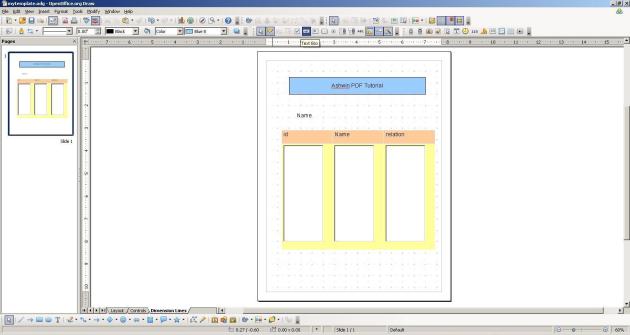



Save PDF to folder where you have your program or just change path for input source in program.

Now here is java code which can convert this PDF to final PDF by filling in Values using IText:
package com.linkwithweb.reports;
import java.io.ByteArrayOutputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import com.lowagie.text.DocumentException;
import com.lowagie.text.pdf.PdfReader;
import com.lowagie.text.pdf.PdfStamper;
public class ITextStamperSample {
public static void main(String[] args) throws IOException,
DocumentException {
generateAshwinFriends();
}
private static void generateAshwinFriends() throws IOException,
FileNotFoundException, DocumentException {
PdfReader pdfTemplate = new PdfReader("mytemplate.pdf");
FileOutputStream fileOutputStream = new FileOutputStream("test.pdf");
ByteArrayOutputStream out = new ByteArrayOutputStream();
PdfStamper stamper = new PdfStamper(pdfTemplate, fileOutputStream);
stamper.setFormFlattening(true);
stamper.getAcroFields().setField("name", "Ashwin Kumar");
stamper.getAcroFields().setField("id", "1\n2\n3\n");
stamper.getAcroFields().setField("friendname",
"kumar\nsirisha\nsuresh\n");
stamper.getAcroFields().setField("relation", "self\nwife\nfriend\n");
stamper.close();
pdfTemplate.close();
}
}
Here is a sample pom.xml:
<project xmlns=http://maven.apache.org/POM/4.0.0
xmlns:xsi=http://www.w3.org/2001/XMLSchema-instance
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.linkwithweb.reports</groupId>
<artifactId>ReportTemplateTutorial</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>ReportTemplateTutorial</name>
<description>ReportTemplateTutorial</description>
<dependencies>
<dependency>
<groupId>com.lowagie</groupId>
<artifactId>itext</artifactId>
<version>2.1.7</version>
</dependency>
</dependencies>
</project>
A PDF file with data filled in placeholders is created with name test.pdf:
