‘MERGE
’ statement is a new feature in SQL Server 2008. It can be used to perform insert
, update
and delete
operation on a destination table simultaneously based on the results of a join with a source table. Well, it sounds a bit confusing, but let's see an example of how it can help us.
Assume we have the following two tables:
Both tables are identical in structure (Structure does not need to be identical).
STUDENT_A
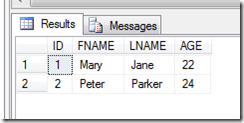
STUDENT_B

And we have to update ‘STUDENT_A
’ with the details of ‘STUDENT_B
’. We need to compare and if student IDs are matched, ‘A
’ table should be updated with the ‘B
’ table. And if the IDs in ‘B
’ Table are new, then we have to insert those to the ‘A
’ table.

So using the ‘MERGE
’ statement, we can achieve this in one execution.
Syntax:
MERGE <Target> [AS T]
USING <Source> [AS S]
ON <Condition>
[WHEN MATCHED THEN <Execution>]
[WHEN NOT MATCHED BY TARGET <Execution>]
[WHEN NOT MATCHED BY SOURCE <Execution>]
And to do the above operation, use the following code:
MERGE STUDENT_A AS T
USING STUDENT_B AS S
ON T.ID = S.ID
WHEN MATCHED THEN UPDATE SET T.AGE = S.AGE
WHEN NOT MATCHED THEN INSERT (ID, FNAME, LNAME, AGE) VALUES(S.ID,S.FNAME,S.LNAME,S.AGE);
**Please note that semicolon ‘;’ is mandatory.
So after executing the above code, and if you inspect the Table ‘A
’, you can see that it’s updated the way we wanted.

And also, you can use additional rules other than your condition. To illustrate that, first we insert a record to both the tables.
insert into STUDENT_A
select 10, 'John','Doe',30
insert into STUDENT_B
select 10, 'John','Doe',30
And using the following code, you can remove the record which matches the condition and have the value 10
.
MERGE STUDENT_A AS T
USING STUDENT_B AS S
ON T.ID = S.ID
WHEN MATCHED and S.ID < 5 THEN UPDATE SET T.AGE = S.AGE
WHEN MATCHED and S.ID = 10 THEN DELETE
WHEN NOT MATCHED BY TARGET THEN INSERT (ID, FNAME, LNAME, AGE)
VALUES(S.ID,S.FNAME,S.LNAME,S.AGE);
And if you inspect the table A
, you can see that it has the same following results:
