Here I am going to discuss about Any
method. One purpose of this method is to check whether the collection has element or not.
Example
List<string> members =
new List<string>() { "pranay", "Hemang" };
bool ISCollectionEmpty = members.Any();
So by using method, I get to know my collection has any element or not.
So when I run the above code, I get true
in my boolean variable, if there is no element it returns false
.
Now consider the below Database Table and LINQ to SQL DBML file.
Department Table
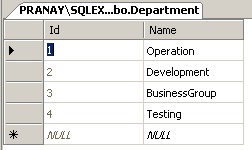
Employee Table


As you can see, there is one to many relationship between Department
and Employee
.
Problem Statement
Now here, I want to list out only those department
s which have employee
.
Solution
Most of the people do the group by
and make use of the join
and then try to find out the department
which has a solution.
But the better solution to this is to make use of Any()
method available in the System.Linq
for the collection as below:
var deptList = from dept in dbcontext.Departments
where dept.Employees.Any()
select dept;
foreach (var item in deptList)
{
Console.WriteLine(item.Name + " : " + item.Employees.Count());
}
Output

As you can see, the above query fetches those department
s only which has employee
and removes the department
s that don't have any.
I am easily able to get the count of the employee
s in department
using count
method.
SQL Query
When you see the SQL profiler or get the query in Visual Studio by watching variable.
SELECT [t0].[Id], [t0].[Name]
FROM [dbo].[Department] AS [t0]
WHERE EXISTS(
SELECT NULL AS [EMPTY]
FROM [dbo].[Employee] AS [t1]
WHERE [t1].[DeptId] = [t0].[Id]
)
CodeProject