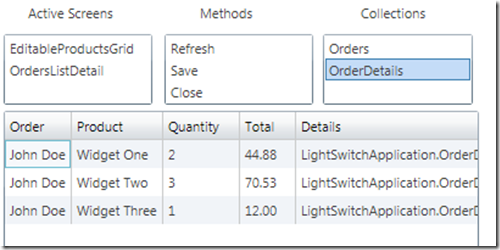
Introduction
When you write code in the LightSwitch client layer, you have programmatic access to most of the application. This also applies when you use Silverlight Custom Controls. This is important because it saves you from writing a lot of code. A professional developer can be far more productive when creating a LightSwitch application than when they are coding a Silverlight application without using LightSwitch.
You have the option of Running a LightSwitch Application with a Blank Shell. When you do that, you still have access to all the features described in this article.
In this article, we will take a random walk through of the LightSwitch API (Application Programming Interface) to give you an idea of the programmatic access available to you from a LightSwitch Silverlight Custom Control (this also applies to LightSwitch Control Extensions).

This page describes the LightSwitch Data Model. There are a lot of classes covered, and the diagram is a very broad overview. However, it does describe the relationship between the major parts.
Note: You must have Visual Studio Professional (or higher) to create a Silverlight control using the method described in this article.
The LightSwitch Explorer Application
We will take our random walk through of the LightSwitch API by creating a sample LightSwitch application. We will create a Silverlight Custom Control called “LightSwitch Explorer” that will display information about the collections of the screen that it is displayed on, raise methods, and switch screens.

We create a simple LightSwitch application that allows Products
and Orders
to be managed.

One screen for Products
.

One screen for Orders
.
The Silverlight Control

Add a new project to the Solution.

We add a Silverlight Class Library.

We select Silverlight 4.

The Project will show in the Solution Explorer, we right-click on the Class1.cs file and delete it.

We add a New Item to the SilverlightControlLibrary
project.

We add a Silverlight User Control, and call it LightSwitchExplorer.xaml.

We right-click on References, and select Add Reference.

We add references to:
Microsoft.LightSwitch
Microsoft.LightSwitch.Base.Client
Microsoft.LightSwitch.Client
This will allow access to the LightSwitch API in the code-behind file in the LightSwitchExplorer.xaml control.

We open the LightSwitchExplorer.xaml.cs file, and add the following using
statements:
using Microsoft.LightSwitch;
using Microsoft.LightSwitch.Client;
using Microsoft.LightSwitch.Details;
using Microsoft.LightSwitch.Details.Client;
using Microsoft.LightSwitch.Model;
using Microsoft.LightSwitch.Presentation;
using System.Windows.Data;
using System.Collections;

We open the LightSwitchExplorer.xaml file, and change the markup to the following:
This add a Grid
and three ListBox
es.
<UserControl x:Class="SilverlightControlLibrary.LightSwitchExplorer"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d"
d:DesignHeight="300" d:DesignWidth="400">
<Grid x:Name="LayoutRoot" Background="White">
<Grid.ColumnDefinitions>
<ColumnDefinition Width="122*" />
<ColumnDefinition Width="122*" />
<ColumnDefinition Width="122*" />
</Grid.ColumnDefinitions>
<Grid.RowDefinitions>
<RowDefinition Height="20*" />
<RowDefinition Height="100*" />
<RowDefinition Height="177*" />
</Grid.RowDefinitions>
<ListBox HorizontalAlignment="Stretch"
VerticalAlignment="Stretch" Margin="5,5,5,5"
Name="lstMethods" Grid.Row="1"
Grid.Column="1" />
<ListBox HorizontalAlignment="Stretch"
VerticalAlignment="Stretch" Margin="5,5,5,5"
Name="lstActiveScreens" Grid.Row="1" />
<ListBox HorizontalAlignment="Stretch"
VerticalAlignment="Stretch" Margin="4,5,5,5"
Name="lstApplicationDetailsProperties"
Grid.Column="2" Grid.Row="1" />
</Grid>
</UserControl>

We drag and drop a DataGrid
on the page.
This will automatically add the needed assemblies to support the DataGrid
.

We click and drag the corners of the grid to size it so it fills the entire bottom half of the page.

In the Properties for the DataGrid
, we check the box next to AutoGenerateColumns
.

We drag and drop a Label
above the first box and change its Content
to “Active Screens”.

We add two more Label
s and name them Methods
and Collections
.
Add Code to the Silverlight Control

Open the LightSwitchExplorer.xaml.cs file, and add the following code:
public static readonly DependencyProperty ExplorerControlDataContextProperty =
DependencyProperty.Register("DummyProperty", typeof(IContentItem),
typeof(LightSwitchExplorer),
new PropertyMetadata(OnExplorerControlDataContextPropertyChanged));

Next, add this line after the InitializeComponent();
line:
this.SetBinding(ExplorerControlDataContextProperty, new Binding());
This sets the binding for ExplorerControlDataContextProperty
.
Add the following methods:
#region ShowMethodsOnScreen
private static void ShowMethodsOnScreen(LightSwitchExplorer EC,
IContentItem contentItem)
{
foreach (var item in contentItem.Screen.Details.Commands.All())
{
EC.lstMethods.Items.Add(item.Name);
}
}
#endregion
#region ShowActiveScreens
private static void ShowActiveScreens(LightSwitchExplorer EC,
IContentItem contentItem)
{
foreach (var item in contentItem.Screen.Details.Application.ActiveScreens)
{
EC.lstActiveScreens.Items.Add(item.Screen.Name);
}
}
#endregion
#region ShowScreenCollections
private static void ShowScreenCollections(LightSwitchExplorer EC,
IContentItem contentItem)
{
foreach (var item in contentItem.Screen.Details.Properties.All())
{
EC.lstApplicationDetailsProperties.Items.Add(
String.Format("{0}", item.Name));
}
}
#endregion
Finally, add the following method that will raise the previous methods when the DataContext
is set or changed:
private static void OnExplorerControlDataContextPropertyChanged(
DependencyObject d, DependencyPropertyChangedEventArgs e)
{
LightSwitchExplorer EC = (LightSwitchExplorer)d;
IContentItem contentItem = (IContentItem)EC.DataContext;
ShowActiveScreens(EC, contentItem);
ShowMethodsOnScreen(EC, contentItem);
ShowScreenCollections(EC, contentItem);
}
Insert the Silverlight Control into the Application

Build the Solution.

Open the EditableProductsGrid
screen, and add a New Custom Control.

Click the Add Reference button.

Create a Project reference to the SilverlightControlLibrary
project.

We will now be able to select the Custom Control we created.

In the Properties for the control, set the Label Position to None.

When we run the application, we see that the control displays the Active Screens, the methods on the current screen, and the Collections on the current screen.
Make the Explorer Control Interactive

Return to the Silverlight Control, and double-click on the Active Screens ListBox.

The method will be wired-up.
Use the following code for the method:
private void lstActiveScreens_SelectionChanged(object sender, SelectionChangedEventArgs e)
{
IContentItem contentItem = (IContentItem)this.DataContext;
string strScreenName = (sender as ListBox).SelectedValue.ToString();
var SelectedScreen = (from ActiveScreens in
contentItem.Screen.Details.Application.ActiveScreens.AsQueryable()
where ActiveScreens.Screen.Name == strScreenName
select ActiveScreens).FirstOrDefault();
if (SelectedScreen != null)
{
SelectedScreen.Activate();
}
}

Double-click on the Methods ListBox
.
Use the following code for the method:
private void lstMethods_SelectionChanged(object sender, SelectionChangedEventArgs e)
{
IContentItem contentItem = (IContentItem)this.DataContext;
string strMethodName = (sender as ListBox).SelectedValue.ToString();
var Method = (from Commands in contentItem.Screen.Details.Commands.All()
where Commands.Name == strMethodName
select Commands).FirstOrDefault();
if (Method != null)
{
var Screen =
(Microsoft.LightSwitch.Client.IScreenObject)contentItem.Screen;
Screen.Details.Dispatcher.BeginInvoke(() =>
{
Method.Execute();
});
}
}

Double-click on the Collections ListBox
.
Use the following code for the method:
private void lstApplicationDetailsProperties_SelectionChanged(object sender,
SelectionChangedEventArgs e)
{
IContentItem contentItem = (IContentItem)this.DataContext;
string strPropertyName = (sender as ListBox).SelectedValue.ToString();
var Property = (from Properties in contentItem.Screen.Details.Properties.All()
where Properties.Name == strPropertyName
select Properties).FirstOrDefault();
dataGrid1.ItemsSource = (IEnumerable)Property.Value;
}
The Explorer Control

Add the Explorer Control to the other pages of the application.
- You can click on the Active Screens to switch screens
- You can click on the Methods to execute them
- You can click on the Collections to see their contents (and edit them)
This is Barely Scratching the Surface
This example barely touches upon all that is available to the LightSwitch developer. Information about the user, the complete database schema and data, and the entire application is available.
Operations that would normally take hundreds of lines of code to manually create in a Silverlight application can be achieved with half a dozen when using LightSwitch.
Special Thanks
A special thanks to Sheel Shah of Microsoft, because otherwise this article would not have been possible.
Further Reading
More LightSwitch Content

You can find a ton of articles on advanced Visual Studio LightSwitch programming at http://lightswitchhelpwebsite.com/.
History
- 10th September, 2011: Initial version