Introduction
This article will show you how to import an Excel file to SQL Server, dynamically, without the need to open the Import wizard from SQL Server. This could be of great help if you have a lot of Excel files to import.
Using the code
First, create the Datamanage
class that holds the import code. The “excelCommand
” will create a new table in the SQL Server database, taking into consideration the names and data types of the Excel file fields.
Public Class Datamanage
Public Sub importToServer(ByVal ExcelPath As String, _
ByVal ServerName As String, _
ByVal DBName As String, ByVal UserName As String, _
ByVal Password As String, ByVal InsertedTableName As String)
Try
Dim ExceCon As String = _
"Provider=Microsoft.ACE.OLEDB.12.0;Data Source=" & _
ExcelPath & "; Extended Properties=Excel 8.0"
Dim excelConnection As System.Data.OleDb.OleDbConnection = _
New System.Data.OleDb.OleDbConnection(ExceCon)
excelConnection.Open()
"Server=Hoss;Database=Excel_Test;Uid=sa;Pwd=sa2008; ].[myTable]
FROM [Sheet1$];"
Dim OleStr As String = "SELECT * INTO [ODBC; Driver={SQL Server};Server=" _
& ServerName & ";Database=" & DBName & ";Uid=" & _
UserName & ";Pwd=" & Password & "; ].[" & _
InsertedTableName & "] FROM [Sheet1$];"
Dim excelCommand As New System.Data.OleDb.OleDbCommand(OleStr, _
excelConnection)
excelCommand.ExecuteNonQuery()
excelConnection.Close()
Catch ex As Exception
Throw New Exception("Error: " & ex.Message)
End Try
End Sub
End Class
Now, the main form:
Imports System.IO
Public Class ImportExcel
Dim dm As New Datamanage
Private Sub Button2_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles Button2.Click
OpenFileDialog1.ShowDialog()
txtexcelPath.Text = OpenFileDialog1.FileName
End Sub
Private Sub Button1_Click(ByVal sender As System.Object,
ByVal e As System.EventArgs) Handles Button1.Click
Try
Dim ExcelPath As String = txtexcelPath.Text.ToLower()
Dim Ext As String = _
ExcelPath.Substring(ExcelPath.LastIndexOf(".") + 1)
If File.Exists(ExcelPath) AndAlso (Ext.Equals("xls") _
Or Ext.Equals("xlsx")) Then
dm.importToServer(OpenFileDialog1.FileName, txtServer.Text,_
txtDbName.Text, txtusername.Text, txtpassword.Text,_
txtInsertedTableName.Text)
ProgressBar1.Visible = False
MessageBox.Show("File imported successfully")
End If
Catch ex As Exception
ProgressBar1.Visible = False
MessageBox.Show(ex.Message)
End Try
End Sub
End Class
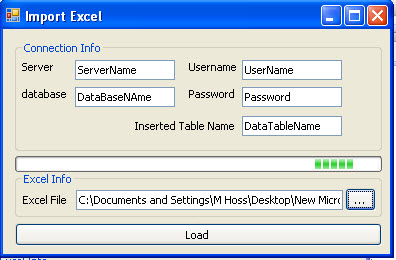
I hope this helps!
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.