Introduction
The purpose of this article is to create a C # FTP client in Secure mode, so if you don’t have much knowledge of FTPS, I advise you to take a look at this: FTPS.
In the .NET Framework, to upload a file in FTPS mode, we generally use the FtpWebRequest
class, but you can not send commands with « quote » arguments, and even if you search on the web, you will not find a concrete example of a secured C# FTP client.
It’s for those reasons I decided to create this article.
SSL Stream?
To send a socket in Secure Socket Layer (SSL) mode, we use the class System.Net.Security.SslStream
.
“Provides a stream used for client-server communication that uses the Secure Socket Layer (SSL) security protocol to authenticate the server and optionally the client”.
For more information, refer to MSDN.
Using the Code
1. Pre-Authenticate
FTPFactory ftp = new FTPFactory();
ftp.setDebug(true);
ftp.setRemoteHost(Settings.Default.TargetFtpSource);
ftp.setRemotePort(990);
ftp.loginWithoutUser();
string cmd = "AUTH SSL";
ftp.sendCommand(cmd);
Before connecting to the FTP server in SSL mode, you have to define the 990 SSL port and send the « AUTH SSL » command authentication using SSL.
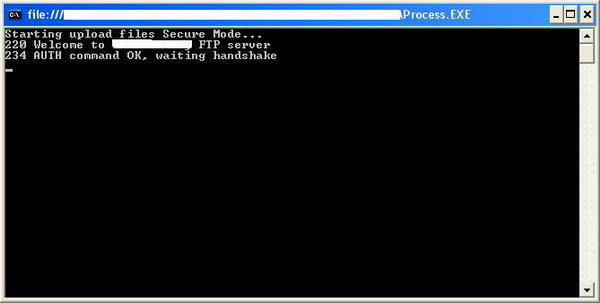
2. Create SSL Stream
ftp.getSslStream();
ftp.setUseStream(true);
ftp.setRemoteUser(Settings.Default.TargetFtpSecureUser);
ftp.setRemotePass(Settings.Default.TargetFtpSecurePass);
ftp.login();
ftp.getSslStream()
creates an SSL stream from client socket which will be used for exchange between the client and the server FTPS. Then, you have to enter a login and password to authenticate on the FTPS server.
Note: if the SSL stream is well established, then I display all the information about the server certificate.

3. Upload File
ftp.setBinaryMode(false);
ftp.uploadSecure(@"Filepath", false);
ftp.close();
Before uploading a file, you have to specify the mode: ftp.setBinaryMode(bool value)
.
value
is false
--> ASCII modevalue
is true
--> Binary mode
By default, it’s binary. Now, you upload the file using:
ftp.uploadSecure(@"Filepath", false)
Note: you can upload in non secured mode using ftp.upload
().
Points of Interest
I learned how an SSL stream and the RAW FTP communication works between a client and an FTP server. Searching on the web, I found many who were stuck on the issue of SSL communication with an FTP server, so I hope this article will be of great help.