In this tip, you will learn a universal and short method to merge GridView rows if neighboring cells show equal values.
Introduction
There are a lot of methods on the Internet solving the problem of how to merge GridView
rows if neighboring cells show equal values. My approach is not the first; however, I think, it is rather universal and very short - less than 20 lines of code.
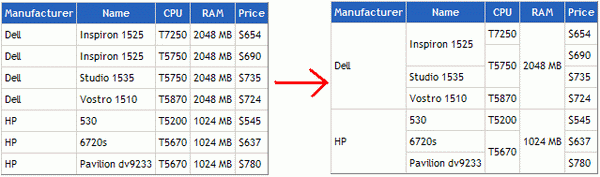
The algorithm is simple: to bypass all the rows, starting from the second at the bottom, to the top. If a cell value is the same as a value in the previous (lower) row, then increase RowSpan
and make the lower cell invisible, and so forth.
The code that merges the cells is very short:
public class GridDecorator
{
public static void MergeRows(GridView gridView)
{
for (int rowIndex = gridView.Rows.Count - 2; rowIndex >= 0; rowIndex--)
{
GridViewRow row = gridView.Rows[rowIndex];
GridViewRow previousRow = gridView.Rows[rowIndex + 1];
for (int i = 0; i < row.Cells.Count; i++)
{
if (row.Cells[i].Text == previousRow.Cells[i].Text)
{
row.Cells[i].RowSpan = previousRow.Cells[i].RowSpan < 2 ? 2 :
previousRow.Cells[i].RowSpan + 1;
previousRow.Cells[i].Visible = false;
}
}
}
}
}
The last action is to add an OnPreRender
event handler for the GridView
:
protected void gridView_PreRender(object sender, EventArgs e)
{
GridDecorator.MergeRows(gridView);
}
History
- 20th March, 2009: Initial version