If you are interested in exploring alternatives to the BooleanToVisibiltyConverter
, then this post is for you. Suppose you have two user interface elements. You want to display the first if a boolean value is true, and the second if the boolean value is false.
Posts in this series:
The Old Way
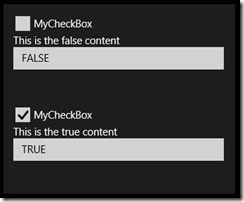
The standard way of doing this is to use a
BooleanToVisibilityConverter
as shown here:
OldWay.xaml
<UserControl
x:Class="VisibilityDemoApp.OldWay"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:vcl="using:VisibilityControlLibrary">
<UserControl.Resources>
<vcl:BooleanToVisibilityConverter
x:Key="BooleanToVisibilityConverter" />
<vcl:BooleanToInvisibilityConverter
x:Key="BooleanToInvisibilityConverter" />
</UserControl.Resources>
<StackPanel>
<CheckBox
x:Name="MyCheckBox"
Content="MyCheckBox" />
<StackPanel
Orientation="Vertical"
Visibility="{Binding ElementName=MyCheckBox, Path=IsChecked, Converter={StaticResource BooleanToInvisibilityConverter}}">
<TextBlock
Text="This is the false content" />
<TextBox
Text="FALSE" />
</StackPanel>
<StackPanel
Orientation="Vertical"
Visibility="{Binding ElementName=MyCheckBox, Path=IsChecked, Converter={StaticResource BooleanToVisibilityConverter}}">
<TextBlock
Text="This is the true content" />
<TextBox
Text="TRUE" />
</StackPanel>
</StackPanel>
</UserControl>
Notice that both the “true” content and the “false
” content are bound separately using the
BooleanToVisibility
and the BooleanToInvisibility
converters.
The New Way
I am offering an alternative to this by introducing a custom control called
VisibilityControl
.
Install VisibilityControl
from NuGet.org and add to your existing Windows 8 application:
- Open your application in Visual Studio
- Select Manage NuGet Packages from the Project menu.
- Click Online. Search for
VisibilityControl
. Click Install.
Once the VisibilityControl library is added to your project, you can re-write the XAML to look like this:
NewWay.xaml
<UserControl
x:Class="VisibilityDemoApp.NewWay"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:vcl="using:VisibilityControlLibrary">
<StackPanel>
<CheckBox
x:Name="MyCheckBox"
Content="MyCheckBox" />
<vcl:VisibilityItemsControl
IsTrue="{Binding ElementName=MyCheckBox, Path=IsChecked}">
<StackPanel
Orientation="Vertical">
<TextBlock
Text="This is the false content" />
<TextBox
Text="FALSE" />
</StackPanel>
<StackPanel
Orientation="Vertical">
<TextBlock
Text="This is the true content" />
<TextBox
Text="TRUE" />
</StackPanel>
</vcl:VisibilityItemsControl>
</StackPanel>
</UserControl>
Notice that the only binding is to the IsTrue
dependency property of the
VisibilityControl
. The first user interface element in the items control will be displayed if the boolean value is false, otherwise the second user interface element will be displayed.
That’s it!
Is It Better?
Well, I would not have written this post if I did not think so – at least in some situations. If you prefer the old way, well, that’s fine by me. I can see advantages of both ways. Of course, there is always method of using VisualStates – with its own list of advantages and disadvantages.
Source and Binaries
VisibilityItemsControl binaries reside here: http://nuget.org/packages/visibilitycontrol/
VisibilityItemsControl source resides here: http://visibilitycontrol.codeplex.com/