My goal is to make adding Settings Charm Flyouts to a C# Metro application as easy as possible. To that end, I created a custom control called CharmFlyout
.
Posts in this series:
Install CharmFlyout from NuGet.org and add to your existing Metro application:
- Install the NuGet Package Manager if you have not already.
- Open your Metro application in Visual Studio
- Select Manage NuGet Packages from the Project menu.
- Click Online. Search for CharmFlyout. Click Install.
If you have a single page application with a MainPage.xaml, then edit MainPage.xaml to:
- Add the using:CharmFlyoutLibrary.
- Add the CharmFlyout custom control.
- Add content to the CharmFlyout. In the example below, the content is a StackPanel.
MainPage.xaml
<Page
x:Class="CharmDemoApp.MainPage"
IsTabStop="false"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:CharmDemoApp"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:cfo="using:CharmFlyoutLibrary"
mc:Ignorable="d">
<Grid>
<cfo:CharmFlyout
x:Name="cfoSettings"
Heading="My Flyout"
HeadingBackgroundBrush="#FF4E0000">
<StackPanel>
<TextBlock
FontSize="16">CharmFlyout by John Michael Hauck</TextBlock>
<TextBlock
FontSize="16">For support:</TextBlock>
<HyperlinkButton
Click="OnMailTo">support@bing.com</HyperlinkButton>
</StackPanel>
</cfo:CharmFlyout>
<TextBlock
VerticalAlignment="Center"
HorizontalAlignment="Center"
FontSize="25"
Foreground="Blue">
Swipe the right side of the screen to show the Settings Charm
<LineBreak />
<LineBreak />
CharmFlyout Demo
<LineBreak />
By John Michael Hauck
<TextBlock.Transitions>
<TransitionCollection>
<EntranceThemeTransition
FromVerticalOffset="400"
FromHorizontalOffset="0" />
</TransitionCollection>
</TextBlock.Transitions></TextBlock>
</Grid>
</Page>
The location of the CharmFlyout
should be such that it occupies the entire MainPage. That is, don’t embed it inside of a
StackPanel
or otherwise restrict its size.
Add the code-behind in
MainPage.xaml.cs as shown here:
MainPage.xaml.cs
using System;
using Windows.UI.ApplicationSettings;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
namespace CharmDemoApp
{
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
SettingsPane.GetForCurrentView().CommandsRequested += CommandsRequested;
}
private void CommandsRequested(SettingsPane sender, SettingsPaneCommandsRequestedEventArgs args)
{
args.Request.ApplicationCommands.Add(new SettingsCommand("s",
"My Flyout", (p) => { cfoSettings.IsOpen = true; }));
}
}
}
That's it. When you run your application, you will see "My Flyout" in the settings charm, and when you click “My Flyout”, this will fly out:
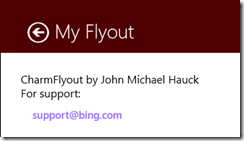
Here is a comparison of the CharmFlyout vs. the Permissions flyout:

CharmFlyout binaries reside here: http://nuget.org/packages/charmflyout/
CharmFlyout source resides here: http://charmflyout.codeplex.com/.
Further Reading
If you are developing an application based on Grid App where there is no MainPage,
then consider reading: CharmFrame – Adding CharmFlyout to GridApps.
If you are supporting sub-flyouts (like the Accounts in the Mail application),
then consider reading: CharmFlyout – Supporting sub-flyouts.