In this article I will show you probably the easiest way to play, snapshot and record RTSP stream of any IP camera using the VLC library and VlcControl. I will also show you all the necessary dependencies and requirements. Source code included, feel free to use it for your purposes.
Links
For testing purposes you can use my demo IP camera RTSP stream:
rtsp://stream.szep.cz/user=test_password=test_channel=1_stream=1
Introduction
RTSP stands for Real-Time Streaming Protocol, a network protocol designed for transmitting multimedia data over a network. It is encountered in audio/video transmission, it works on the application layer of the ISO/OSI reference model and the default port is 554. Practically every IP camera or NVR supports RTSP. Remember, sometimes you have to enable RTSP on the camera and the specific RTSP address may be different depending on the IP camera manufacturer.
You can easily play the RTSP stream in your C# WinForm application using the VLC library and VlcControl.
The example project is able to play, snapshot, record and save video stream to file. It also checks if 32-bit VLC Media Player is installed on your computer - if not, it will direct you to download it.
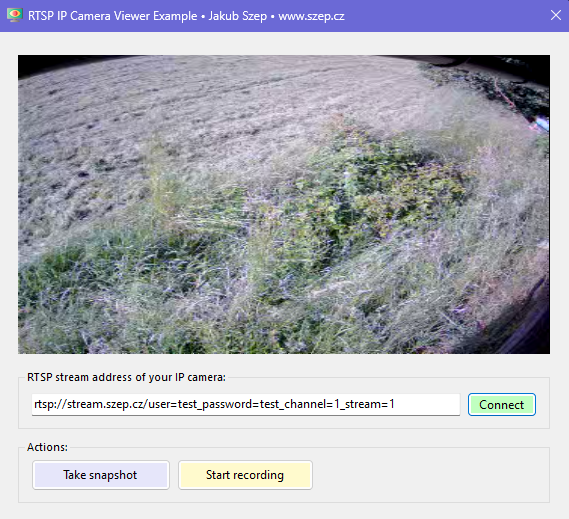
Necessary Things to Do
You must have VLC Media Player already installed on computer where you want to execute this project.
If you want to use VLCControl in your own project, first of all you have to install NuGet package 'VLC.DotNet.Forms':
Project > Manage NuGet Packages > Browse > Type 'VLC.DotNet.Forms' > Install

Then add VlcControl to your formr:
Toolbox > VlcControl > drop VlcControl somewhere into your form


All VLC Controls needs to use vlclib.dll located in VLC installation folder, in my case C:\Program Files (x86)\VideoLAN\VLC.
This path must be always set in VlcLibDirectory
property (you can easily set this property in designer). In example project, VlcLibDirectory
is already set.

Using the code
At the moment we already have 32-bit VLC Media Player installed on computer, NuGet package installed and VlcControl, in my case named VLCPlayer, added to the form.
1. How to play RTSP stream
VLCPlayer.Stop();
VLCPlayer.SetMedia(new Uri("rtsp://something.xx/"), string.Empty);
VLCPlayer.Play();
or you can also use
VLCPlayer.Play(new Uri("rtsp://something.xx/"));
2. How to play and record RTSP stream, then save recording to file
You have to use double backslash when defining the path, otherways it doesn't work.
bool IsRecording;
if (!IsRecording)
{
if (VLCPlayer.IsPlaying)
{
string path = $@"c:\\Users\\{Environment.UserName}\\Desktop\\{Guid.NewGuid()}.mp4";
var mediaOptions = new[] { ":sout=#duplicate{dst=display,dst=std{access=file,mux=mp4,dst=\"" + path + "\"}" };
VLCPlayer.SetMedia(new Uri("rtsp://something.xx/"), mediaOptions);
VLCPlayer.Play();
IsRecording = true;
}
}
else
{
IsRecording = false;
VLCPlayer.Stop();
VLCPlayer.SetMedia(new Uri("rtsp://something.xx/"), string.Empty);
VLCPlayer.Play();
}
3. How to take snapshot, then save to file
You have to use double backslash when defining the path, otherways it doesn't work.
if (VLCPlayer.IsPlaying)
{
string path = $@"c:\\Users\\{Environment.UserName}\\Desktop\\{Guid.NewGuid()}.png";
VLCPlayer.TakeSnapshot(path);
}
or if you want to specify output image size in pixels (for example 400x300):
if (VLCPlayer.IsPlaying)
{
string path = $@"c:\\Users\\{Environment.UserName}\\Desktop\\{Guid.NewGuid()}.png";
VLCPlayer.TakeSnapshot(path, 400, 300);
}
4. How to set the audio volume
VLCPlayer.Audio.Volume = 69;
5. How to mute the audio
VLCPlayer.Audio.IsMute = true;
6. How to set custom aspect ratio
VLCPlayer.Video.AspectRatio = "16:9";