UITextField
has a property called inputAccessoryView
that can be set to a UIToolbar
to give the user something to do after inputting text.
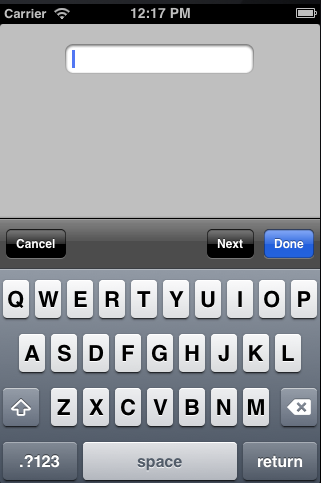
I have already set up text field as a synthesized property of my view controller, called nameTextField
, and linked it to by nib.
viewDidLoad
is a good place to create this toolbar, and I’ll call it keyboardToolBar
.
UIToolbar * keyboardToolBar = [[UIToolbar alloc] initWithFrame:CGRectMake(0, 0, 320, 50)];
keyboardToolBar.barStyle = UIBarStyleBlackTranslucent;
This gives us a black, translucent UIToolbar
that is 320 x 50. We can set the items by passing an array of UIBarButtonItem
to [keyboardToolBar setItems:(NSArray*)]
. The constructor of a UIBarButtonItem
that I am using [[UIBarButtonItem alloc] initWithTitle:(NSString *)title style:(UIBarButtonItemStyle)style target:(id)target action:(SEL)action];
UIBarButtonItemStyle
are predefined styles you can use, I suggest you browse through the options. The target is the object to send action (a method/message) to, which is triggered when the button is pressed. You need to implement these methods to avoid a crash.
I have decided on three buttons, and a spacer in between. The spacer is simply a UIBarButtonItem
initialized with [UIBarButtonItem alloc] initWithBarButtonSystemItem: UIBarButtonSystemItemFlexibleSpace target:nil action:nil];
. This pushes all previous buttons to be left aligned and all buttons after it to be right aligned.
All together, we have:
[keyboardToolBar setItems:
[NSArray arrayWithObjects:[[UIBarButtonItem alloc]initWithTitle:@"Cancel"
style:UIBarButtonItemStyleBordered target:self
action:@selector(cancelNumberPad)],
[[UIBarButtonItem alloc]initWithBarButtonSystemItem:
UIBarButtonSystemItemFlexibleSpace
target:nil action:nil],
[[UIBarButtonItem alloc] initWithTitle:@"Next"
style:UIBarButtonItemStyleBordered target:self
action:@selector(nextTextField)],
[[UIBarButtonItem alloc]initWithTitle:@"Done"
style:UIBarButtonItemStyleDone target:self
action:@selector(doneWithTextField)], nil]];
Finally, we make the connection from our text field to the new toolbar with:
nameTextField.inputAccessoryView = keyboardToolBar;
You can grab the Xcode project here.
The post Simple UIToolbar as Keyboard Accessory appeared first on Code and Prose.