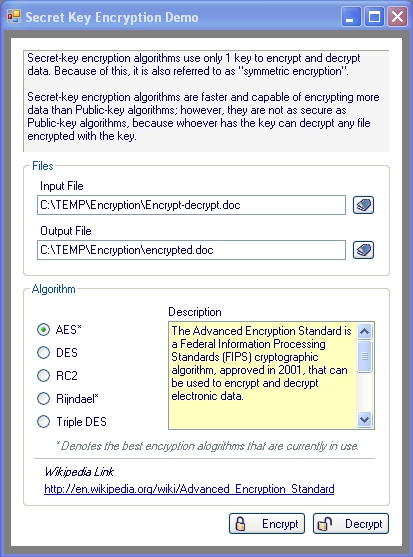
Introduction
Encryption is the process of transforming electronic data from a "plain text" state to an unreadable state by use of a cipher, also known as an algorithm.
Although formerly pretty complex, encryption can now be implemented very easily in any .NET application using the various encryption classes in the System.Security.Cryptography
namespace.
I've put together an encryption demo program that demonstrates how to encrypt and decrypt documents using the following encryption methods:
- AES (Advanced Encryption Standard)
- DES (Data Encryption Standard)
- RC2 ("Ron's Code" or "Rivest Cipher")
- Rijndael
- Triple DES (Triple Data Encryption Standard)
This application uses persistent keys in combination with the respective algorithms to encrypt and decrypt data.
When appropriate, you will see a C# snippet first, and then a VB version of the same.
Using the Code
- Pass the selected file to the
Encrypt
and Decrypt
methods below:
Dim path As String = "C:\temp\Encryption\Encrypt-decrypt.doc"
Dim encrytedPath As String = String.Empty
encrytedPath = Encrypt(path)
MessageBox.Show("The following file has been encrypted:" & _
vbCrLf & vbCrLf & encrytedPath)
path = Decrypt(encrytedPath)
MessageBox.Show("The following file has been decrypted:" & _
vbCrLf & vbCrLf & path)
Declare the instance of the AES class that will be used to encrypt and decrypt:
private System.Security.Cryptography.Aes mAes = System.Security.Cryptography.Aes.Create();
Private mAes As System.Security.Cryptography.Aes = _
System.Security.Cryptography.Aes.Create()
The code for the Encrypt
method:
public override void Encrypt(string inputFilePath, string outputFilePath)
{
byte[] key = this.GetKey();
byte[] iv = this.GetIV();
ICryptoTransform encryptor = mAes.CreateEncryptor(GetKey(), GetIV());
base.PerformEncryption(encryptor, inputFilePath, outputFilePath);
}
Private Function Encrypt(ByVal path As String) As String
Dim outputPath As String = String.Concat(path, ".enc")
Dim key As Byte() = GetKey()
Dim iv As Byte() = GetIV()
Dim encryptor As ICryptoTransform = mAes.CreateEncryptor(GetKey(), GetIV())
Dim reader As New FileStream(path, FileMode.Open, FileAccess.Read)
Dim writer As New FileStream(outputPath, FileMode.Create, FileAccess.Write)
Dim encryptedStream As _
New System.Security.Cryptography.CryptoStream(writer, _
encryptor, CryptoStreamMode.Write)
Dim bites(1023) As Byte
Dim bitesRead As Int32 = 0
Dim totalBites As Int64 = 0
Do Until totalBites >
The code for the Decrypt
method:
public override void Decrypt(string inputFilePath, string outputFilePath)
{
byte[] key = this.GetKey();
byte[] iv = this.GetIV();
ICryptoTransform decryptor = mAes.CreateDecryptor(key, iv);
base.PerformDecryption(decryptor, inputFilePath, outputFilePath);
}
Private Function Decrypt(ByVal path As String) As String
Dim decryptedPath As String = path.Replace(".enc", "")
Dim key As Byte() = GetKey()
Dim iv As Byte() = GetIV()
Dim decryptor As ICryptoTransform = mAes.CreateDecryptor(key, iv)
Dim reader As New FileStream(path, FileMode.Open, FileAccess.Read)
Dim writer As New FileStream(decryptedPath, _
FileMode.Create, FileAccess.Write)
Dim decryptedStream As _
New System.Security.Cryptography.CryptoStream(writer, _
decryptor, CryptoStreamMode.Write)
Dim bites(1023) As Byte
Dim bitesRead As Int32 = 0
Dim totalBites As Int64 = 0
Do Until totalBites >
Persisted Key and Vector (IV):
private byte[] GetKey()
{
byte[] key = new byte[32/span>];
key[<span class="code-digit">0] = 195;
key[1] = 201;
key[2] = 141;
key[3] = 238;
key[4] = 118;
key[5] = 179;
key[6] = 108;
key[7] = 7;
key[8] = 37;
key[9] = 109;
key[10] = 7;
key[11] = 48;
key[12] = 66;
key[13] = 141;
key[14] = 2;
key[15] = 65;
key[16] = 213;
key[17] = 136;
key[18] = 141;
key[19] = 210;
key[20] = 78;
key[21] = 234;
key[22] = 83;
key[23] = 34;
key[24] = 41;
key[25] = 238;
key[26] = 152;
key[27] = 88;
key[28] = 228;
key[29] = 72;
key[30] = 160;
key[31] = 174;
return key;
}
private byte[] GetIV()
{
byte[] IV = new byte[16];
IV[0] = 30;
IV[1] = 185;
IV[2] = 3;
IV[3] = 73;
IV[4] = 26;
IV[5] = 247;
IV[6] = 159;
IV[7] = 124;
IV[8] = 211;
IV[9] = 112;
IV[10] = 251;
IV[11] = 231;
IV[12] = 69;
IV[13] = 25;
IV[14] = 132;
IV[15] = 1;
return IV;
}
Private Function GetKey() As Byte()
Dim key(31) As Byte
key(0) = 195
key(1) = 201
key(2) = 141
key(3) = 238
key(4) = 118
key(5) = 179
key(6) = 108
key(7) = 7
key(8) = 37
key(9) = 109
key(10) = 7
key(11) = 48
key(12) = 66
key(13) = 141
key(14) = 2
key(15) = 65
key(16) = 213
key(17) = 136
key(18) = 141
key(19) = 210
key(20) = 78
key(21) = 234
key(22) = 83
key(23) = 34
key(24) = 41
key(25) = 238
key(26) = 152
key(27) = 88
key(28) = 228
key(29) = 72
key(30) = 160
key(31) = 174
Return key
End Function
Private Function GetIV() As Byte()
Dim IV(15) As Byte
IV(0) = 30
IV(1) = 185
IV(2) = 3
IV(3) = 73
IV(4) = 26
IV(5) = 247
IV(6) = 159
IV(7) = 124
IV(8) = 211
IV(9) = 112
IV(10) = 251
IV(11) = 231
IV(12) = 69
IV(13) = 25
IV(14) = 132
IV(15) = 1
Return IV
End Function
Points of Interest
The process for encrypting and decrypting each of the different algorithms is the same. One of the things you will find different is the number of bytes that are used in the different key and vector arrays.
Hopefully, this demo is helpful to you!