Introduction
When working with SQL joins, we can chose among from various choices, depending on the requirement. Well, each join sequence may differ from one another, and serves a special purpose. But few among these join queries differ in syntax /performance but they produce the same thing. To avoid confusion, here we will see the basic differences and similarities between various joins of MS SQL Server.
Background
Let’s say we have two tables:
CREATE Table Student
(
Id BIGINT,
Name VARCHAR(100)
);
CREATE Table StudentAge
(
Student_Id BIGINT,
Name VARCHAR(100),
Age INT
);
Yes, there is no real foreign key between them, but let’s say we populated these tables with relational data like:
INSERT INTO Student
VALUES
(1, 'Dipon'),
(2, 'Dip'),
(3, 'Dipa'),
(4, 'Dipika'),
(5, 'Shamim'),
(6, 'Shatu'),
(6, 'Shatu')
INSERT INTO StudentAge
VALUES
(1, 'Dipon', 10),
(2, 'Dip', 20),
(5, 'Shamim', 40),
(5, 'Shamim', 50),
(6, 'Shatu', 50),
(7, 'Jamal', 50),
(8, 'Kamal', 60)
As we can see, considering these two tables, some of the rows have relational data and for few there is none.
Let’s Start Joining
Gentlemen like to do hand shake
Sometimes, they shake hand if they only know each other.
Sometimes, they do the same even if they don’t know each other.
Sometimes, one may feel embarrassed, if he does find anyone to shake hands with.
Shakes hand - join made between rows
Know each other - has any data relation
Embarrassment revealed - no row found to join, NULL
Regular Joins
Shake hand if they know each other, no embarrassment revealed.
Join: JOIN
SELECT *
FROM Student
JOIN StudentAge ON Student.Id = StudentAge.Student_Id;
Join: INNER JOIN
SELECT *
FROM Student
INNER JOIN StudentAge ON Student.Id = StudentAge.Student_Id;
Join: WHERE
SELECT *
FROM Student, StudentAge
WHERE Student.Id = StudentAge.Student_Id;
CROSS APPLY
SELECT *
FROM Student
CROSS APPLY(
SELECT *
FROM StudentAge
WHERE Student.Id = StudentAge.Student_Id
)StudentAges
They all produced the same thing:
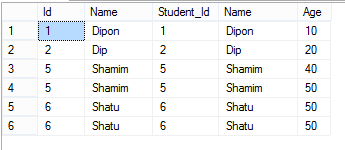
http://www.sqlfiddle.com/#!3/517ad/1
Left Joins
Each person shakes hands if they know each other, embarrassment revealed for the left man.
Join: LEFT JOIN
SELECT *
FROM Student
LEFT JOIN StudentAge ON Student.Id = StudentAge.Student_Id;
Join: LEFT OUTER JOIN
SELECT *
FROM Student
LEFT OUTER JOIN StudentAge ON Student.Id = StudentAge.Student_Id;
OUTER APPLY
SELECT *
FROM Student
OUTER APPLY(
SELECT *
FROM StudentAge
WHERE Student.Id = StudentAge.Student_Id
)StudentAges
They all produced the same thing.

http://www.sqlfiddle.com/#!3/517ad/2
Right Joins
Each person shakes hand if they know each other, embarrassment revealed for the right man.
Join: RIGHT JOIN
SELECT *
FROM Student
RIGHT JOIN StudentAge ON Student.Id = StudentAge.Student_Id;
Join: RIGHT OUTER JOIN
SELECT *
FROM Student
RIGHT OUTER JOIN StudentAge ON Student.Id = StudentAge.Student_Id;
OUTER APPLY
SELECT Students.*, StudentAge.*
FROM StudentAge
OUTER APPLY(
SELECT *
FROM Student
WHERE StudentAge.Student_Id = Student.Id
)Students
They all produced the same thing.

http://www.sqlfiddle.com/#!3/517ad/3
Full Joins
Each person shakes hand if they know each other, embarrassment revealed for both men.
Join: FULL JOIN
SELECT *
FROM Student
FULL JOIN StudentAge ON Student.Id = StudentAge.Student_Id;
Join: FULL OUTER JOIN
SELECT *
FROM Student
FULL OUTER JOIN StudentAge ON Student.Id = StudentAge.Student_Id;
They all produced the same thing.

http://www.sqlfiddle.com/#!3/517ad/4
Cross Joins
Each one shakes hand with everyone.
Join: CROSS JOIN
SELECT *
FROM Student
CROSS JOIN StudentAge;
Join: TABLE
SELECT *
FROM Student, StudentAge;
CROSS APPLY
SELECT *
FROM Student
CROSS APPLY(
SELECT *
FROM StudentAge
)StudentAges
They all produced the same thing.


http://www.sqlfiddle.com/#!3/517ad/5
Other Joins!!!
When working with joins, we may face a situation where we want to do few more things like:
- LEFT JOIN EXCLUDING INNER JOIN
- RIGHT JOIN EXCLUDING INNER JOIN
- OUTER JOIN EXCLUDING INNER JOIN
- Etc. or some real basics
Check it out at http://www.codeproject.com/Articles/33052/Visual-Representation-of-SQL-Joins, which is a great article by C.L. Moffatt.
Cross/Outer Apply !!!
The only intention to use Cross/ Outer Apply is performance issue. It performs faster than the normal joins. For more, take some overview from:
Limitations
Yes, there could be something which I misunderstood or presented. So if you find anything, just let me know.