Introduction
In this project, I have explained navigation between two pages in Windows 8 app with data. Here, the most important thing is “Public Static
” Keyword. I have created public static Imagesource
(or “int
” or “string
”) reference in mainpage.xaml.cs. After that, assign to the value of the object.
I have explained simple but very useful two examples.
In the first example, I have transferred image source between one page and another page.
In the second example, I have transferred string
value between one page and another page.
Using the Code
Example 1 (Transfer Imagesource)
Step 1
Open Visual Studio and create a new project. Select blank app. After that meaning full assign name. Then ok.
Step 2
Paste your four images in Asstes folder.
Step 3
After that four image control drag and drop in mainpage.xaml like this.
<Grid Background="{StaticResource ApplicationPageBackgroundThemeBrush}">
<Image HorizontalAlignment="Left" Height="251" Margin="88,108,0,0"
VerticalAlignment="Top" Width="403"/>
<Image HorizontalAlignment="Left" Height="251"
Margin="713,123,0,0" VerticalAlignment="Top" Width="403"/>
<Image HorizontalAlignment="Left" Height="251"
Margin="88,416,0,0" VerticalAlignment="Top" Width="403"/>
<Image HorizontalAlignment="Left" Height="251"
Margin="713,416,0,0" VerticalAlignment="Top" Width="403"/>
</Grid>
Step 4
After that, define the Image control name like 1st Image control name “Image1
”, 2nd one is “Image2
”, 3rd one is “Image3
” and 4th
id is “Image4
”.
<Grid Background="{StaticResource ApplicationPageBackgroundThemeBrush}">
<Image x:Name="Image1" HorizontalAlignment="Left" Height="251"
Margin="88,108,0,0" VerticalAlignment="Top" Width="403"/>
<Image x:Name="Image2" HorizontalAlignment="Left" Height="251"
Margin="713,123,0,0" VerticalAlignment="Top" Width="403"/>
<Image x:Name="Image3" HorizontalAlignment="Left" Height="251"
Margin="88,416,0,0" VerticalAlignment="Top" Width="403"/>
<Image x:Name="Image4" HorizontalAlignment="Left" Height="251"
Margin="713,416,0,0" VerticalAlignment="Top" Width="403"/>
</Grid>
Step 5
After that, all images set the source and all images fire tapped event. So code looks like this:
<Grid Background="{StaticResource ApplicationPageBackgroundThemeBrush}">
<Image x:Name="Image1" HorizontalAlignment="Left" Height="251" Source=" Assets/1.jpg" Tapped="Image_Tapped_1"
Margin="88,108,0,0" VerticalAlignment="Top" Width="403"/>
<Image x:Name="Image2" HorizontalAlignment="Left" Height="251" Source=" Assets/2.jpg" Tapped="Image_Tapped_2"
Margin="713,123,0,0" VerticalAlignment="Top" Width="403"/>
<Image x:Name="Image3" HorizontalAlignment="Left" Height="251" Source=" Assets/3.jpg" Tapped="Image_Tapped_3"
Margin="88,416,0,0" VerticalAlignment="Top" Width="403"/>
<Image x:Name="Image4" HorizontalAlignment="Left" Height="251" Source=" Assets/4.jpg" Tapped="Image_Tapped_4"
Margin="713,416,0,0" VerticalAlignment="Top" Width="403"/>
</Grid>
Step 6
Create one reference of ImageSource
class name ImageSource1
above of the main page contructor.
public static ImageSource ImageSource1;
public MainPage()
{
this.InitializeComponent();
}
Step 7
After that project, right click add > add new item and select basic page.
Build the solution.
Step 8
After that Image_Tapped_1
event, code like this:
ImageSource1 = Image1.Source;
this.Frame.Navigate(typeof(BasicPage1));
Step 9
Similar to Image_Tapped_2
, Image_Tapped_3
, Image_Tapped_4
event, but only one change. Assign value of ImageSource1
change.
private void Image_Tapped_2(object sender, TappedRoutedEventArgs e)
{
ImageSource1 = Image2.Source;
this.Frame.Navigate(typeof(BasicPage1));
}
private void Image_Tapped_3(object sender, TappedRoutedEventArgs e)
{
ImageSource1 = Image3.Source;
this.Frame.Navigate(typeof(BasicPage1));
}
private void Image_Tapped_4(object sender, TappedRoutedEventArgs e)
{
ImageSource1 = Image4.Source;
this.Frame.Navigate(typeof(BasicPage1));
}
Step 10
After that, take one image control in Basicpage1.xaml and meaning full assign name like “mycatchimage
”.
<Image Name="mycatchimage" HorizontalAlignment="Left" Height="193" Margin="110,40,0,0" Grid.Row="1" VerticalAlignment="Top" Width="356"/>
Step 11
Assign the source of mycatchimage
in BasicPage1.xaml.cs.
public BasicPage1()
{
this.InitializeComponent();
mycatchimage.Source = MainPage.ImageSource1;
}
Step 12
You have run the code which is something like this:
MainPage
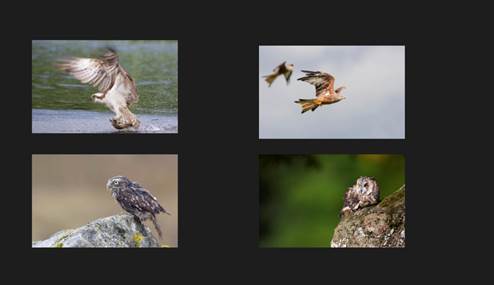
BasicPage1

Example 2 (Transfer String value)
Step 1
Open Visual Studio and create a new project. Select blank app. After that meaning full assign name. Then ok.
Step 2
After that one TextBox
and Button
control drag and drop in mainpage.xaml like this:
<Grid Background="{StaticResource ApplicationPageBackgroundThemeBrush}">
<TextBox HorizontalAlignment="Left" Margin="351,172,0,0" TextWrapping="Wrap"
Text="TextBox" VerticalAlignment="Top" Height="66" Width="412"/>
<Button Content="Click" HorizontalAlignment="Left"
Margin="496,307,0,0" VerticalAlignment="Top"/>
</Grid>
Step 3
Meaningful name of TextBox
control like “myText
”. And fire the button click event.
<Grid Background="{StaticResource ApplicationPageBackgroundThemeBrush}">
<TextBox Name="myText" HorizontalAlignment="Left" Margin="351,172,0,0"
TextWrapping="Wrap" Text="TextBox" VerticalAlignment="Top"
Height="66" Width="412"/>
<Button Content="Click" HorizontalAlignment="Left"
Margin="496,307,0,0"
VerticalAlignment="Top" Click="Button_Click"/>
</Grid>
Step 4
Create one reference of string
class name “Data
” above of the main page constructor.
public static string Data;
public MainPage()
{
this.InitializeComponent();
}
Step 5
After that project, right click add > add new item and select basic page.
Build the solution.
Step 6
After that Button_Click
event code like this:
private void Button_Click(object sender, RoutedEventArgs e)
{
Data = myText.Text;
this.Frame.Navigate(typeof(BasicPage1));
}
Step 7
After that, take one Textblock
control in Basicpage1.xaml and meaning full assign name like “mycatchtext
”.
<TextBlock Name="mycatchtext" HorizontalAlignment="Left"
Margin="172,78,0,0"
Grid.Row="1" TextWrapping="Wrap" Text="TextBlock"
VerticalAlignment="Top" Height="440" Width="815"/>
Step 8
Assign the Text
of mycatchtext
in BasicPage1.xaml.cs.
public BasicPage1()
{
this.InitializeComponent();
mycatchtext.Text = MainPage.Data;
}
Step 9
You have run the code which is something like this:
MainPage

BasicPage1

This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.