Creating List using Code
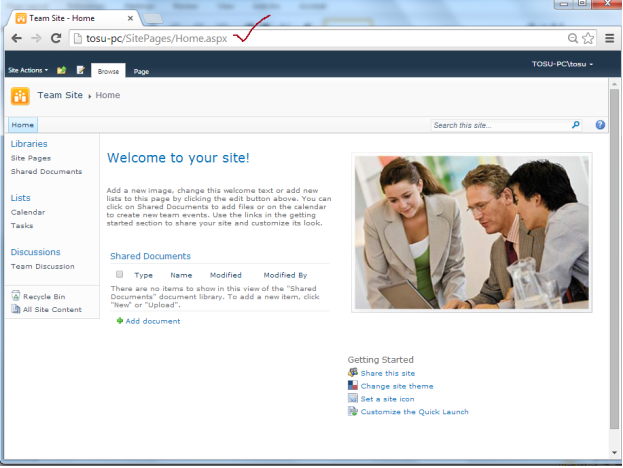
For this demo, you are using the above team site.
Part A: Create and Delete a List of Authors
(1) Open Visual Studio 2010
(2) File > new > Project

- Select Windows
- Windows Form Application
- Name: WindowsFormsApplications1
- Click Ok button
(3) In Solution Explorer
- Right click on Reference > Add

- Select .NET tab
- Select Microsoft.SharePoint
- Click Ok
(4) In Solution Explorer
- Right click on Properties > Open
- In the properties window, set the properties as shown below in images.


(5) Open Form1 page

- Create two button names and one listbox as shown in the above figure.
(6) Form1.cs page
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using Microsoft.SharePoint;
namespace WindowsFormsApplication1
{
public partial class Form1 : Form
{
private const string SiteUrl = "http://tosu-pc/SitePages/Home.aspx";
public Form1()
{
InitializeComponent();
}
private void CreateListButton_Click(object sender, EventArgs e)
{
using (var site = new SPSite(SiteUrl))
{
var web = site.RootWeb;
var listId = web.Lists.Add("Authors", string.Empty, SPListTemplateType.GenericList);
var list = web.Lists[listId];
list.OnQuickLaunch = true;
list.Update();
listBox1.Items.Add("Authors list created");
}
}
private void DeleteListButton_Click(object sender, EventArgs e)
{
using (var site = new SPSite(SiteUrl))
{
var web = site.RootWeb;
var list = web.Lists.TryGetList("Authors");
if (list != null)
{
list.Delete();
}
listBox1.Items.Add("Authors list deleted");
}
}
}
}
(7) Run (F5)

- Click Create List button

(8) Now browse the team site (http://tosu-pc/SitePages/Home.aspx)
- Refresh the page

- Authors list is created as shown in above figure
- Click Authors list

Delete a List of Authors
(9) Return to Visual Studio Run mode

- Now click the Delete List button

- Authors list deleted is display in listBox1
(10) Browse the Team site
- Refresh it
- Authors list is deleted from list as shown in figure below:

Part B: Change the Name of Default Column (Title) to User Name
(1) Update the Form1.cs
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using Microsoft.SharePoint;
namespace WindowsFormsApplication1
{
public partial class Form1 : Form
{
private const string SiteUrl = "http://tosu-pc/SitePages/Home.aspx";
public Form1()
{
InitializeComponent();
}
private void CreateListButton_Click(object sender, EventArgs e)
{
using (var site = new SPSite(SiteUrl))
{
var web = site.RootWeb;
var listId = web.Lists.Add("Authors", string.Empty, SPListTemplateType.GenericList);
var list = web.Lists[listId];
list.OnQuickLaunch = true;
list.Update();
var title = list.Fields["Title"];
title.Title = "User Name";
title.Update();
listBox1.Items.Add("Authors list created");
}
}
private void DeleteListButton_Click(object sender, EventArgs e)
{
using (var site = new SPSite(SiteUrl))
{
var web = site.RootWeb;
var list = web.Lists.TryGetList("Authors");
if (list != null)
{
list.Delete();
}
listBox1.Items.Add("Authors list deleted");
}
}
}
}
(2) Run F5
(3) Check in Browser

- Title column is replaced by User Name
Part B: Add New Column in List
(1) Update the Form1.cs
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using Microsoft.SharePoint;
namespace WindowsFormsApplication1
{
public partial class Form1 : Form
{
private const string SiteUrl = "http://tosu-pc/SitePages/Home.aspx";
public Form1()
{
InitializeComponent();
}
private void CreateListButton_Click(object sender, EventArgs e)
{
using (var site = new SPSite(SiteUrl))
{
var web = site.RootWeb;
var listId = web.Lists.Add("Authors", string.Empty, SPListTemplateType.GenericList);
var list = web.Lists[listId];
list.OnQuickLaunch = true;
list.Update();
var title = list.Fields["Title"];
title.Title = "User Name";
title.Update();
var empFieldName = list.Fields.Add("Employee", SPFieldType.Boolean, false);
var rateFieldName = list.Fields.Add("Salary/Rate" , SPFieldType.Currency, true);
var bioFieldName = list.Fields.Add("Bio", SPFieldType.Note, false);
var bio = list.Fields[bioFieldName] as SPFieldMultiLineText;
bio.NumberOfLines = 8;
bio.RichText = false;
bio.Update();
var view = list.DefaultView;
view.ViewFields.Add(empFieldName);
view.ViewFields.Add(rateFieldName);
view.ViewFields.Add(bioFieldName);
view.Update();
listBox1.Items.Add("Authors list created");
}
}
private void DeleteListButton_Click(object sender, EventArgs e)
{
using (var site = new SPSite(SiteUrl))
{
var web = site.RootWeb;
var list = web.Lists.TryGetList("Authors");
if (list != null)
{
list.Delete();
}
listBox1.Items.Add("Authors list deleted");
}
}
}
}
(2) F5

(3) Check in the Browser
- Refresh it

- List Authors is created with column name: User Name, Employee, Salary/Rate and Bio
- Click Add New Item

- After entering Item in pop up and finally click save
- The view being populatated as shown below:

Part C: Use Validation
- If not Employee, then salary is less than $50
(1) Update the Form1.cs
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using Microsoft.SharePoint;
namespace WindowsFormsApplication1
{
public partial class Form1 : Form
{
private const string SiteUrl = "http://tosu-pc/SitePages/Home.aspx";
public Form1()
{
InitializeComponent();
}
private void CreateListButton_Click(object sender, EventArgs e)
{
using (var site = new SPSite(SiteUrl))
{
var web = site.RootWeb;
var listId = web.Lists.Add("Authors", string.Empty, SPListTemplateType.GenericList);
var list = web.Lists[listId];
list.OnQuickLaunch = true;
list.ValidationFormula = "IF([Employee], TRUE, [Salary/Rate] <=50)";
list.ValidationMessage = "The maximum rate for a contributor is $50";
list.Update();
var title = list.Fields["Title"];
title.Title = "User Name";
title.Update();
var empFieldName = list.Fields.Add("Employee", SPFieldType.Boolean, false);
var rateFieldName = list.Fields.Add("Salary/Rate" , SPFieldType.Currency, true);
var bioFieldName = list.Fields.Add("Bio", SPFieldType.Note, false);
var bio = list.Fields[bioFieldName] as SPFieldMultiLineText;
bio.NumberOfLines = 8;
bio.RichText = false;
bio.Update();
var view = list.DefaultView;
view.ViewFields.Add(empFieldName);
view.ViewFields.Add(rateFieldName);
view.ViewFields.Add(bioFieldName);
view.Update();
listBox1.Items.Add("Authors list created");
}
}
private void DeleteListButton_Click(object sender, EventArgs e)
{
using (var site = new SPSite(SiteUrl))
{
var web = site.RootWeb;
var list = web.Lists.TryGetList("Authors");
if (list != null)
{
list.Delete();
}
listBox1.Items.Add("Authors list deleted");
}
}
}
}
(2) F5
- Make sure Authors list is deleted before creating

- View in the browser

- Click Add new item

Part D: Add Items into the List using VS
(1) Update the Form1.cs
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using Microsoft.SharePoint;
namespace WindowsFormsApplication1
{
public partial class Form1 : Form
{
private const string SiteUrl = "http://tosu-pc/SitePages/Home.aspx";
public Form1()
{
InitializeComponent();
}
private void CreateListButton_Click(object sender, EventArgs e)
{
using (var site = new SPSite(SiteUrl))
{
var web = site.RootWeb;
var listId = web.Lists.Add("Authors", string.Empty, SPListTemplateType.GenericList);
var list = web.Lists[listId];
list.OnQuickLaunch = true;
list.ValidationFormula = "IF([Employee], TRUE, [Salary/Rate] <=50)";
list.ValidationMessage = "The maximum rate for a contributor is $50";
var titleFildName = "Title";
var title = list.Fields[titleFildName];
title.Title = "User Name";
title.Update();
var empFieldName = list.Fields.Add("Employee", SPFieldType.Boolean, false);
var rateFieldName = list.Fields.Add("Salary/Rate" , SPFieldType.Currency, true);
var bioFieldName = list.Fields.Add("Bio", SPFieldType.Note, false);
var bio = list.Fields[bioFieldName] as SPFieldMultiLineText;
bio.NumberOfLines = 8;
bio.RichText = false;
bio.Update();
list.Update();
var view = list.DefaultView;
view.ViewFields.Add(empFieldName);
view.ViewFields.Add(rateFieldName);
view.ViewFields.Add(bioFieldName);
view.Update();
var item = list.Items.Add();
item[titleFildName] = "Abhishek";
item[empFieldName] = false;
item[rateFieldName] = 30;
item.Update();
item = list.Items.Add();
item[titleFildName] = "Rajkumar";
item[empFieldName] = true;
item[rateFieldName] = 50;
item.Update();
item = list.Items.Add();
item[titleFildName] = "Rahul";
item[empFieldName] = true;
item[rateFieldName] = 60;
item.Update();
item = list.Items.Add();
item[titleFildName] = "milind";
item[empFieldName] = false;
item[rateFieldName] = 40;
item.Update();
listBox1.Items.Add("Authors list created");
}
}
private void DeleteListButton_Click(object sender, EventArgs e)
{
using (var site = new SPSite(SiteUrl))
{
var web = site.RootWeb;
var list = web.Lists.TryGetList("Authors");
if (list != null)
{
list.Delete();
}
listBox1.Items.Add("Authors list deleted");
}
}
}
}
(2) F5
- Make sure list is deleted first.

- Check in the browser.

- Click on milind.

Part E: Transfer the Code to Actual SharePoint
(1) Open the another instance of Visual Studio 2010
(2) File > New > Project



(3) In solution Explorer
- Right click on Features

- Add Feature
(4) Rename the Features as CreateList

- Make changes as shown in the above pic and save

- Right click on CreateList > Add Event Receiver

(5) Update the CreateList.EventReceiver.cs
using System;
using System.Runtime.InteropServices;
using System.Security.Permissions;
using Microsoft.SharePoint;
using Microsoft.SharePoint.Security;
namespace ListAndLibraries1.Features.CreateList
{
[Guid("cc22c160-bb85-4906-a4ee-8f49d4e93203")]
public class CreateListEventReceiver : SPFeatureReceiver
{
public override void FeatureActivated(SPFeatureReceiverProperties properties)
{
var web = properties.Feature.Parent as SPWeb;
if (web == null) return;
var listId = web.Lists.Add("Authors", string.Empty, SPListTemplateType.GenericList);
var list = web.Lists[listId];
list.OnQuickLaunch = true;
list.ValidationFormula = "IF([Employee], TRUE, [Salary/Rate] <=50)";
list.ValidationMessage = "The maximum rate for a contributor is $50";
var titleFildName = "Title";
var title = list.Fields[titleFildName];
title.Title = "User Name";
title.Update();
var empFieldName = list.Fields.Add("Employee", SPFieldType.Boolean, false);
var rateFieldName = list.Fields.Add("Salary/Rate", SPFieldType.Currency, true);
var bioFieldName = list.Fields.Add("Bio", SPFieldType.Note, false);
var bio = list.Fields[bioFieldName] as SPFieldMultiLineText;
bio.NumberOfLines = 8;
bio.RichText = false;
bio.Update();
list.Update();
var view = list.DefaultView;
view.ViewFields.Add(empFieldName);
view.ViewFields.Add(rateFieldName);
view.ViewFields.Add(bioFieldName);
view.Update();
var item = list.Items.Add();
item[titleFildName] = "Abhishek";
item[empFieldName] = false;
item[rateFieldName] = 30;
item.Update();
item = list.Items.Add();
item[titleFildName] = "Rajkumar";
item[empFieldName] = true;
item[rateFieldName] = 50;
item.Update();
item = list.Items.Add();
item[titleFildName] = "Rahul";
item[empFieldName] = true;
item[rateFieldName] = 60;
item.Update();
item = list.Items.Add();
item[titleFildName] = "milind";
item[empFieldName] = false;
item[rateFieldName] = 40;
item.Update();
}
public override void FeatureDeactivating(SPFeatureReceiverProperties properties)
{
var web = properties.Feature.Parent as SPWeb;
if (web == null) return;
var list = web.Lists.TryGetList("Authors");
if (list != null)
{
list.Delete();
}
}
}
}
(6) Change the Feature Receiver not activated automatically

- Now Feature is not activated automatically, we have to activate it manually.
(7) Deploy

- Refresh the page
- Site Action > Site Settings

- Inside Site Actions
- Select Manage Site template

- Author List is created with Activate Feature
- Click Activate

- Authors List is created
- Click Authors list

(8) To check the validation

- Click List tab
- Click List Settings in the Ribbon

- Click Validation Settings

(9) Deactivated the Feature
- Site Actions > Site Settings
- Inside Site Actions > Manage Site Features

- Click Deactivate as shown in the above figure.

- Click Deactivate this feature
