Introduction
This article is the Part-6 Article of my series Hack Proof your asp.net and asp.net mvc applications.
In this article i will describe how to obfuscate your JavaScript code (Your written business logic in JavaScript or those JavaScript libraries you don't want to expose to others ) in asp.net application with visual studio.
Background
You can read previous article of this series from below links :
- Secure your ASP.NET applications from SQL Injection
- Secure your ASP.NET applications from XSS Attack
- Secure your ASP.NET applications from CSRF Attack
- Secure your ASP.NET applications from Sensitive Data Exposure and Information Leakage
- Secure your ASP.NET applications from Session Hijacking
Obfuscation
Obfuscation is the process which involves the process to convert your code to a equivalent or specific format such that it becomes difficult to understand and difficult to reverse engineering.
Confustion b/w Minification of JavaScript and Obfuscation of JavaScript files:
Minification is the process to remove the unnecessary spaces from a file where as obfuscation is the process to make code difficult to understand.
Minification
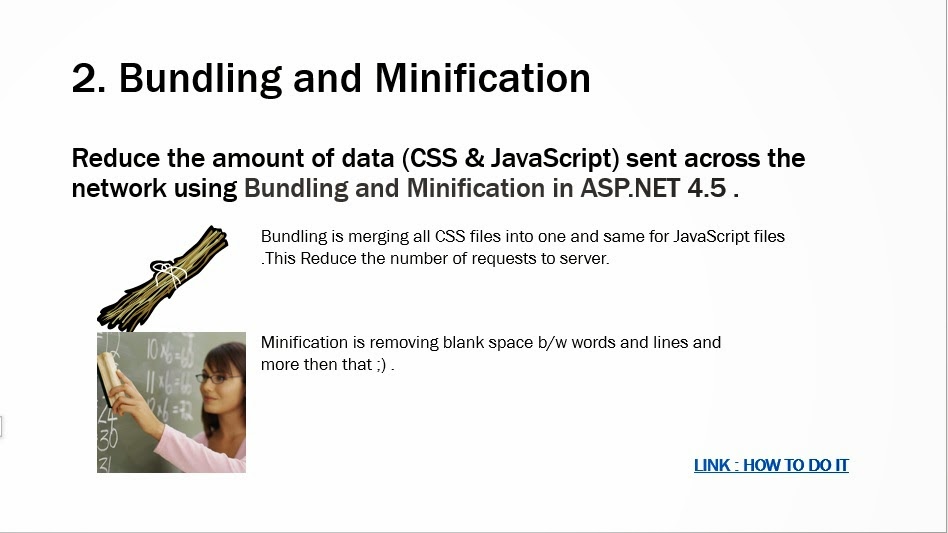
Above picture is from my article : Tips and Tricks for Faster Asp.NET and Asp.net MVC applications
Obfuscation

Why we need Obfuscation
Code obfuscation scrambles the symbols, code of a program, rendering it diificult to understand while at the same time preserving the program's functionality.
we can do obfuscation of .NET assemblies and JAVA Code and Javascripts.In this article i am just covering Javascript and obfuscation is not limited to source code , you can use it for your data too and in real life :P :P :D .
Benefits of Obfuscation
- Protection of intellectual property(Your own written code)
- Reduced security threats(By Pervention of the code exposure in a descriptive manner)
- Reduced size of the file(Minification and shorten the variables name)
- No network delays
How to apply JavaScript Obfuscation in ASP.NET Application
Prerequisite :
- Visual Studio 2010 , 2012 , 2013
- Asp.Net framework 4 and 4.5 and above (whenever will come)
- Obviously a ASP.NET and ASP.NET MVC application
in my case i am using VS 2012 and asp.net framework 4.5.
Step 1: Install Bundle Transformer nuget package
Using package manager console install this Bundle Transformer.
Go To Tools > Library package manager > Package manager console
Install-Package BundleTransformer.UglifyJs
BundleTransformer contains many minifiers , but we are here going to cover only Uglify to achieve Obfuscation.For more details about BundleTrasformer Minifiers , Translators and Postprocessors visit https://bundletransformer.codeplex.com/.

Step 2 : Install-Package JavaScriptEngineSwitcher.Msie
using the same package manager console install the JavaScriptEngineSwitcher.Msie.As a JS-engine BundleTransformer use the JavaScript Engine Switcher library . For correct working of this module is recommended to install one of the following NuGet packages: JavaScriptEngineSwitcher.Msie or JavaScriptEngineSwitcher.V8.
Install-Package JavaScriptEngineSwitcher.Msie

Step 3 : Do the Web.Config Setting for uglify
When you installed the bundletransformer its automatically have created a node <bundleTransformer> .Under this node add the following configuration code for uglify.
<uglify>
<js screwIe8="false" severity="0">
<parsing strict="false" />
<compression compress="true" sequences="true" propertiesDotNotation="true"
deadCode="true" dropDebugger="true" unsafe="false"
conditionals="true" comparisons="true" evaluate="true"
booleans="true" loops="true" unused="true"
hoistFunctions="true" keepFunctionArgs="false" hoistVars="false"
ifReturn="true" joinVars="true" cascade="true"
globalDefinitions="" pureGetters="false" pureFunctions=""
dropConsole="false" angular="false" />
<mangling mangle="true" except="" eval="false"
sort="false" topLevel="false" />
<codeGeneration beautify="false" indentLevel="4" indentStart="0"
quoteKeys="false" spaceColon="true" asciiOnly="false"
inlineScript="false" width="80" maxLineLength="32000"
bracketize="false" semicolons="true"
comments="false" preserveLine="false"
unescapeRegexps="false" />
</js>
<jsEngine name="MsieJsEngine" />
</uglify>

If you will see i have added name="MsieJsEngine" under <uglify> node of <JsEngine> .Yo can use JavaScriptEngineSwitcher.V8 also.
Step 4 - Modify the BundleConfig
When you create a new web form application or MVC application , asp.net framework 4.5 templates automatically create a folder App_Start for code that runs on application startup.
Folder App_Start > BundleConfig
1. Add following namespaces
using BundleTransformer.Core.Builders;
using BundleTransformer.Core.Orderers;
using BundleTransformer.Core.Resolvers;
using BundleTransformer.Core.Transformers;
2. Initialize Script and Style transformer , nullbuilder and nullorder class
bundles.UseCdn = true;
var nullBuilder = new NullBuilder();
var styleTransformer = new StyleTransformer();
var scriptTransformer = new ScriptTransformer();
var nullOrderer = new NullOrderer();
3. create your own ScriptBundle to which you want to Obfuscate
var scriptbundleToObfuscate = new Bundle("~/bundles/WebFormsJs");
scriptbundleToObfuscate.Include("~/Scripts/WebForms/WebForms.js",
"~/Scripts/WebForms/WebUIValidation.js",
"~/Scripts/WebForms/MenuStandards.js",
"~/Scripts/WebForms/Focus.js",
"~/Scripts/WebForms/GridView.js",
"~/Scripts/WebForms/DetailsView.js",
"~/Scripts/WebForms/TreeView.js",
"~/Scripts/WebForms/WebParts.js");
scriptbundleToObfuscate.Builder = nullBuilder;
scriptbundleToObfuscate.Transforms.Add(scriptTransformer);
scriptbundleToObfuscate.Orderer = nullOrderer;
bundles.Add(scriptbundleToObfuscate);
For Demo purpose i am using the WebForms.js and the bundle for the same which is created by VisualStudio Automatically.
4. Enableoptimization True to see the result.
BundleTable.EnableOptimizations = true;
Make it false at the time of development so it will not bundle , minify and obfuscate the JS files.Never forget to make it True before publishing the application.
Final Step : Include the bundle in your application and See the results:
Asp.Net Web forms :
<%: Scripts.Render("~/bundles/WebFormsJs") %>
Asp.Net MVC :
@Scripts.Render("~/bundles/WebFormsJs")
Before Obfuscation :

After Obfuscation :

yay :) :) ...
Thanks for reading this article.For code Verification and if you are facing issue in Configuration you can download and see the code from here : https://github.com/sarveshkushwaha/JavaScriptObfuscationInAspNET
References and further readings:
https://bundletransformer.codeplex.com/wikipage?title=Bundle%20Transformer%201.9.24
http://www.mcpressonline.com/security/general/protect-your-intellectual-property-using-obfuscation.html