In this article, we will create a Responsive jQuery 3D Content Image Slider in ASP.NET MVC using Adaptor.
You can find the plugin here.
Adaptor content slider aims to provide a simple interface for developers to create cool 2D or 3D slide animation transitions.
You can download the plugin from Github.
Implementation
Create a new project in ASP.NET MVC named JQueryImageSlider
.
After that, copy the CSS, img, js folders and paste in JQueryImageSlider
project.
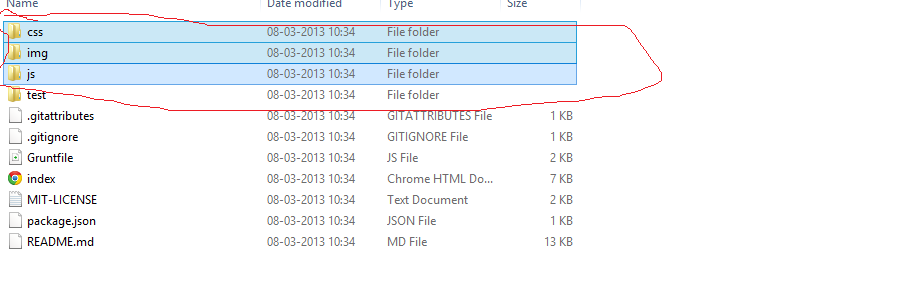
jquery image slider 3d

jquery image 3d slider
In the _Layout.cshtml page, reference the Adaptor 3D slider files.

jquery image slider reference files
Now create class models ImageModel
that holds images from database.
public class ImageModel
{
public int ImageID { get; set; }
public string ImageName { get; set; }
public string ImagePath { get; set; }
}
Get the images list from the repository and bind it to slider.
public ActionResult Index()
{
List imageList = new List();
imageList.Add(new ImageModel { ImageID = 1,
ImageName = "Image 1", ImagePath = "/img/the-battle.jpg" });
imageList.Add(new
ImageModel { ImageID = 2,
ImageName = "Image 2", ImagePath = "/img/hiding-the-map.jpg"
});
imageList.Add(new ImageModel { ImageID = 3,
ImageName = "Image 3", ImagePath = "/img/theres-the-buoy.jpg" });
imageList.Add(new ImageModel { ImageID = 4,
ImageName = "Image 4", ImagePath = "/img/finding-the-key.jpg" });
imageList.Add(new ImageModel { ImageID = 5,
ImageName = "Image 5", ImagePath = "/img/lets-get-out-of-here.jpg"});
return View(imageList);
}
In the View page, add the following code:
<div id="viewport-shadow">
<a href="#" id="prev"
title="go to the next slide"></a>
<a href="#" id="next"
title="go to the next slide"></a>
<div id="viewport">
<div id="box">
@*Bind images here*@
@foreach (var item in Model)
{
<figure class="slide">
<img src=@item.ImagePath>
</figure>
}
</div>
</div>
<div id="time-indicator"></div>
</div>
@* here we are binding the slider controls navigation *@
<footer>
<nav class="slider-controls">
<ul id="controls">
@{int index = 0;}
@foreach (var item in Model)
{
string cssClass = index.Equals(0) ?
"goto-slide current" : "goto-slide";
<li><a class="@cssClass"
href=" #" data-slideindex="@index"></a></li>
index= index +1;
}
</ul>
</nav>
</footer>
Adding Caption for the Slider
We can add the caption for image slider using the figcaption
tag.
<figure class="slide">
<img src=@item.ImagePath class="img-responsive">
<figcaption>Static Caption</figcaption>
</figure>
Output

Image Slider
Responsive Output

You can download the source code from GitHub.
The post jQuery 3D Content Image Slider in ASP.NET MVC appeared first on Venkat Baggu Blog.