1. Overview
The purpose of this document is to
help you understand the basics of different styles of programming. In my
opinion, the best programming style is object oriented programming. In this document
I will discuss different kinds of developing, like Functional Programming,
Modular Programming and eventually, Object Oriented Programming. Of course, all
keywords and relevant information belonging to each style will be discussed.
In this document, different styles of programming will be
explained:
- Functional programming
- Modular programming
- Object Oriented Programming
2. Functional programming
Functional programming is a style of programming that
emphasizes the evaluation of expressions rather than the execution of commands.
This means that a program, written in a functional programming language,
consists of a set function definitions and an expression, whose value is output
as the program’s result. There are no side results (like an assignment) to
expression evaluation, so an expression will always evaluate to the same value,
if its evaluation terminates.
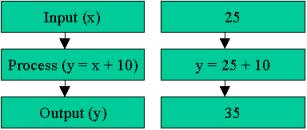
Because of this, an expression can always be replaced by its
value without changing the overall result. This is called referential
transparency.
The word ‘function’ actually can be replaced with the term
‘action’. A function executes something, for example
Drive(brand_of_car)
Referential transparency
Pure functional languages achieve referential transparency
by forbidding assignment to global variables. Each expression is a constant or
a function application whose evaluation has no side effect, it only returns a
value and that value depends only on the definition of the function and the
values of its arguments.
3. Modular programming
What is modular programming
A module, in modular programming, is a series of functions
(or procedures) that are related in some way. This way of programming is used
in many applications.
Way of work
Given a problem, you begin with analysis of the problem. You
carefully look at the requirements of the problem and you make sure all
questions are answered before you begin with design of the modules. Then, you
break the problem in subproblems. Each of these problems you solve in one or
more modules. All the modules together solve the complete problem. Modules
itself may be divided into smaller modules.
you divide the problem in sub-problems.
In the same way as you break the problem in smaller problems, you break a
module in sub-modules.
A program (module) is divided in sub-modules.
As said, each sub-problem will be
solved in the different sub-modules. In the end the whole problem is solved in
the whole module.
A module is a set of functions (actions, see chapter two)
that are related to each other. For example, a module called “Driving” contains
the functions drive(brand_of_car)
,
accelerate(speed)
and stop()
. A modulair program is always more clearly
than a pure functional program because the different modules are classified on
base of relevance.
References
Modular programming
http://www.eee.bham.ac.uk/dsvp_gr/roxby/eem1e1/lecture7/sld004.htm
Modular design and programming
http://www.cs.runet.edu/~jchase/pdl.html
4. Object Oriented Programming (OOP)
What is OOP?
Object Oriented Programming (or OOP) is a revolutionary
concept that changed the rules in computer program development. OOP is
organized around objects rather than actions. Normally, a program has a simple
flow: input data, process the data and output a result. OOP has a different
view; what we really care about are the objects we want to manipulate, not the
logic required to manipulate them. Examples of such objects can be human beings
(for example, described by name, address and so on), or buildings and floors
(whose properties can be described and managed) or even little things, like a
button or a toolbar.
Object Oriented Programming is an alternative to modular
programming. The design technique associated with OOP is Object Oriented
Design. In an object oriented program, the modules are classes rather then
procedures. A class is a collection of objects.
As said, OOP is all about objects. An object is actually a
container filled with information. You can see an object as a black box, which sends and receives messages. A
black box, or object, contains code
and data. Code are sequences of
computer instructions, and data is information on which the instructions
operate. In C ++ units code are called functions
and units of data are called structures.
Functions and structures are not connected to each other. For example, a
function can operate on more than one structure, and a structure can be used in
more than one function.
A structure can be public data, these can be accessed by
another object. An object can also contain private data. This data can only be
accessed by the object itself, and not by another object. The private data is
implemented so that an outstanding object cannot modify data while it’s not
necessary or data that is not supposed to be modified.
An object
Messages
All the communication between objects,
is done by messages. An object sends, but also
receives messages. The object, to which the
message is sent, is the receiver of the message. Messages define the interface of the
object. At least everything an object can do is represented by his messages.
Actually, an object usually can do more, because an object also can have
private functions.
Messages
Normally, messaging is done between the objects. One
object receives a message from an other object, and sends a message back to the
same, or to an other object.
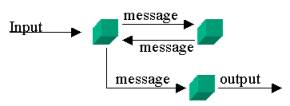
This is just a simple presentation of messaging
between the objects. Even in a small program, the amount of objects can be very
large. In this example, the first object receives input. This can be a message
from another object or, for example, something the user types. The first
objects processes this input, sends a message to the second object. This
message can be a result of a function, for example. The second objects
processes the message and sends a result back to the first object. With this
result, the first object does a new calculation, for example, and sends the
result of this to the third object. This object also processes the data and
gives output. This output can be a new message to another object, or simply
print something on the user’s monitor.
Providing access to an object by sending and receiving
messages, while keeping the details of the object private, is called information hiding. Another word, which
means the same, is incapsulation. Information hiding is a good thing; one object should
only know that things about another object that are relevant. Make members in
an object private or protected when possible.
Methods
An object is defined by his class. A class determines
everything about the object. Objects are individual instances of a class. It’s possible to have, for example, more
buttons on one dialog. Each button is an instance of the buttonclass. Another
example: consider the class ‘Dog’. An object
from this class is called ‘Spot’. The ‘Dog’ class defines what the object is.
It is possible to have more than one object of this class; you might want to
call them ‘Fido’, ‘Rover’ and so on. Each one of them is an instance of the
class ‘Dog’. Now, let’s say that the ‘Dog’ class defines they can understand
messages like ‘Bark’ and ‘Roll-over’. The action that the message carries out
is called a method. This is, in fact,
the code that’s getting executed when the message is received by an object.
spot.bark();
Arguments are
often supplied as a part of a message. For example, the message ‘Roll-over’,
may need the arguments ‘how fast’ and a second argument, ‘how many times’.
Arguments specify the way an action behaves.
Inheritance also means, that you reuse. You don’t have to write all the code again, you just reuse
one or more classes that have the behavior you want.
Now, what if we want to create a class (named ‘Wolf’) that
can do exactly what ‘Dog’ does? We don’t have to rewrite the entire class; we
just create a class derived from
‘Dog’. This class inherits all the
existing messages of the so-called base-class;
it has the same behavior.
In the class ‘Wolf’ we may create new (extra) messages, for
example ‘hunt’. This class supports al the
messages of ‘Dog’, plus some new ones, including ’hunt’.
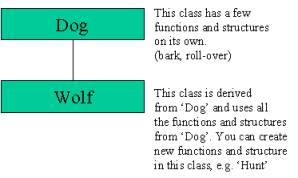
Data modeling
Data modeling is a first step in designing an object
oriented program. As a result of data modeling, you can define the objects. A
simple approach to creating a data model that allows you to visualize the
model, is to draw a square to represent each individual data item that you know
about, and then to express relationships between each of these data items. The
relationships between the data items, can be expressed with words like ‘is part
of’, or ‘is used by’ or ‘uses’ and so forth. From this total description, you
can create a set of classes and subclasses that define all the general
relationships.
With this data model, you can easily create a set of classes
or even a complete program, because this data model defines the working of the
program.
Differences between OOP and functional & modular
programming
The difference between OOP and functional and modular
programming, is that the objects in OOP only manipulate their own condition. For
example: Peugeot::Drive()
, Citroen::Drive()
,
Car::Accelerate(speed)
, and car::Stop()
. The condition of all the objects together specify the
condition of the complete program. An object oriented program is always easier
to read than a modular program, because all the objects represents their
information. The way an OO program works can be defined by drawing the
relations between the objects. That is exactly why it is always a good idea to
draw a data model with the objects, and their relations, you need.
Besides that, an OO program is easier to maintain, because
changing the functionality of an object will (usually) have no effect on the
state of other objects. For implementing new functionality, it might be enough
to simply add on or more objects to the application and define their relations.
The fact of reusing code can be seen with the possibility to
base specialized information (Peugeot, Citroen) on general information (car).
In C we had to code our own bugs. In C++ we can inherit
them.
Object Oriented Programming
http://www.whatis.com/oop.htm
What is Object Oriented Software:
http://www.soft-design.com/softinfo/objects.html
About the Author
Alex Marbus is a developer who lives in Harderwijk, the
Netherlands. His CE website is at www.cewebserver.com.