Introduction
Communicating with USB modem using C# is shown in this example. It detects and lists the ports at which USB modem is connected, communicates with USB Modem using AT Commands. retrieves the basic information from USB device. You can use the WMI Code Creator tool to generate WMI Query script in VBScript, C#, and VB.NET.
To download WMI, click here.
Screenshot of Example
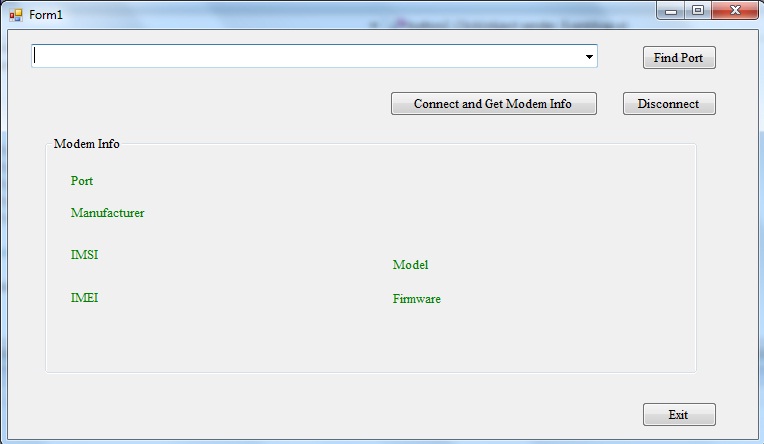
Commands Used in Example
Here are the commands used in this example:
- AT+CGMM
To Get the USB Model Identification
Product Name: EC122
Input: AT+CGMM
Output: EC122
- AT+CGMR: To get Firmware version of the modem
- AT+CGSN: To get modem IMEI
- AT+CIMI: To get IMSI Number
- AT+CGMI: To get Manufacturer of USB Model
- AT: To get Modem's Attention
Code Sections
- Detecting and listing the port names to which USB Modem is connected. Here, we are using the WMI Query to get the names of port to which USB modem is connected.
ManagementObjectSearcher searcher = new ManagementObjectSearcher
("root\\CIMV2", "SELECT * FROM Win32_PnpEntity ");
ManagementObjectSearcher searcher1 = new ManagementObjectSearcher
("root\\CIMV2", "SELECT * FROM Win32_POTSModem ");
- The section of code shown below accomplishes the task of opening the appropriate port, and communicating with it using the AT commands, arranging data elements in array, displaying it. Here, we are using the function
WriteLine()
to write at output Buffer and ReadExisting()
to read the data from input Buffer. After successful reading of the data, we use Array
to arrange the data elements.
sp.PortName = (string)comboBox1.SelectedItem;
if (!sp.IsOpen)
{
sp.Open();
if (sp.IsOpen)
{
MessageBox.Show("Connected to Port" + portname);
sp.WriteLine("AT"); sp.WriteLine("ATI"); sp.WriteLine("AT+CGMM"); sp.WriteLine("AT+CGMI"); sp.WriteLine("AT+CIMI"); sp.WriteLine("AT+CGSN"); sp.WriteLine("AT+CGMR"); }
}
t = sp.ReadExisting()).Contains("OK")
string[] f = new string[100];
string[] c = t.Split(z);
int m = 0;
for(int i = 0; i < c.Length; i++)
{
if (!(c[i].Equals("")))
{
f[m] = c[i];
m++;
}
}
if ((f[i].Equals("AT+CGMI")))
{
label6.Text = f[i + 1]; }
if ((f[i].Equals("AT+CIMI")))
{
label7.Text = f[i + 1]; }
if ((f[i].Equals("AT+CGSN")))
{
label8.Text = f[i + 1]; }
if ((f[i].Equals("AT+CGMM")))
{
label11.Text = f[i + 1]; }
if ((f[i].Equals("AT+CGMR")))
{
label12.Text = f[i + 1]; }
How It Works
Visual Studio 2008 is used for developing this code.
- On clicking "Find Port" button, it lists names of port to which USB modem is connected.
- Select the appropriate port from dropdownlist.
- Then click on "Connect and Get Modem Info" button to get the information from modem.
- It retrieves the information such as Manufacturer, IMSI Number, IMEI Number, Model of USB Device, Port to which modem is connected, Firmware version.
- On clicking "Disconnect" button, it disconnects the modem from the port.
- Clicking on "Exit" button, it exits the application.
This code works Fine on EC122 CDMA USB Modem.