Introduction
I have been needing an easy Gantt Chart for work and found there are almost no examples out there for C# and Winforms, but I found a good one in Visual Basic and even though I don't write in Visual Basic, I decided to convert it to C#.
Background
I found Adagio.81's Gantt Chart posted on October 2, 2008 and started there. You can see it at Gantt-Chart. This Gantt Chart has all the same features of Adagio81's Gantt Chart but I did fix a few little things and I updated the bar's values after a bar is moved and you can see the new values when you hover over in the tool tip.
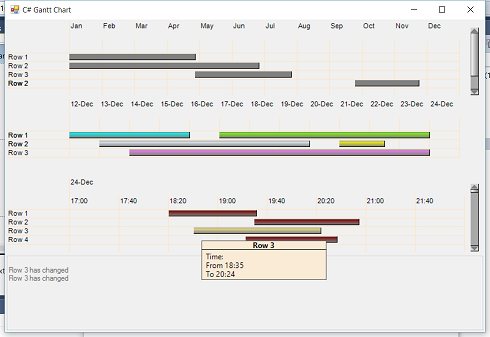
Using the Code
I add my code in the Form1.cs. There are three examples in Load
and you have to hook up your events there also.
ganttChart1 = new GanttChart();
ganttChart1.AllowChange = false;
ganttChart1.Dock = DockStyle.Fill;
ganttChart1.FromDate = new DateTime(2015, 12, 12, 0, 0, 0);
ganttChart1.ToDate = new DateTime(2015, 12, 24, 0, 0, 0);
tableLayoutPanel1.Controls.Add(ganttChart1, 0, 1);
ganttChart1.MouseMove += new MouseEventHandler(ganttChart1.GanttChart_MouseMove);
ganttChart1.MouseMove += new MouseEventHandler(GanttChart1_MouseMove);
ganttChart1.MouseDragged += new MouseEventHandler(ganttChart1.GanttChart_MouseDragged);
ganttChart1.MouseLeave += new EventHandler(ganttChart1.GanttChart_MouseLeave);
ganttChart1.ContextMenuStrip = ContextMenuGanttChart1;
List<BarInformation> lst1 = new List<BarInformation>();
lst1.Add(new BarInformation("Row 1", new DateTime(2015, 12, 12),
new DateTime(2015, 12, 16), Color.Aqua, Color.Khaki, 0));
lst1.Add(new BarInformation("Row 2", new DateTime(2015, 12, 13),
new DateTime(2015, 12, 20), Color.AliceBlue, Color.Khaki, 1));
lst1.Add(new BarInformation("Row 3", new DateTime(2015, 12, 14),
new DateTime(2015, 12, 24), Color.Violet, Color.Khaki, 2));
lst1.Add(new BarInformation("Row 2", new DateTime(2015, 12, 21),
new DateTime(2015, 12, 22, 12, 0, 0), Color.Yellow, Color.Khaki, 1));
lst1.Add(new BarInformation("Row 1", new DateTime(2015, 12, 17),
new DateTime(2015, 12, 24), Color.LawnGreen, Color.Khaki, 0));
foreach (BarInformation bar in lst1)
{
ganttChart1.AddChartBar
(bar.RowText, bar, bar.FromTime, bar.ToTime, bar.Color, bar.HoverColor, bar.Index);
}