Source files were corrupted. I uploaded sources again.
Introduction
I have been interested in WTL (Windows Template Library) for a very long time. So, I decided to make a small program using WTL last September. I really like the fact that the size of the executable files that WTL makes is much smaller than the ones that MFC (Microsoft Foundation Classes) makes and the source code is open for everyone to see. Some people might think that there are no advantages between WTL and MFC. But I believe that these tools are both powerful for anyone who wants to build small, fast programs.
If some errors show up related to libraries, you might have to install the run-time components below.
Visual C++ Redistributable for Visual Studio 2015
I have tested this program on Windows 7 and Windows XP. I don’t know if it will work on other Windows platforms.
Features
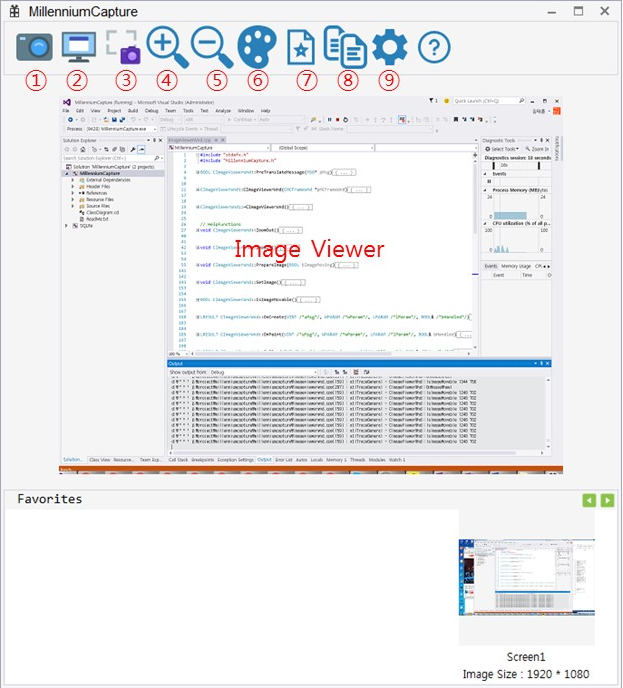
- Capture Full Screen - You can take a snapshot of the whole screen.
- Capture Window - You can capture one window on your screen.
- Capture Region - You can specify what you want to capture.
- Zoom In
- Zoom Out
- Copy the captured Image to MS Paint
- Favorites
- Copy the captured image to the Clipboard
- Environment (Hotkeys)
- Image viewer - includes panning function

Implementation
Sending the Captured Image to Microsoft Paint
.
if (OpenClipboard())
{
EmptyClipboard();
CBitmap bmpCopy;
BITMAP bm;
if (GetObject(pImageData->m_bmpOri.m_hBitmap, sizeof(BITMAP), &bm))
bmpCopy = (HBITMAP)CopyImage(pImageData->m_bmpOri.m_hBitmap, IMAGE_BITMAP, 0, 0, 0);
ATLASSERT(::GetObjectType(bmpCopy.m_hBitmap) == OBJ_BITMAP);
SetClipboardData(CF_BITMAP, bmpCopy.m_hBitmap);
CloseClipboard();
}
if (IsClipboardFormatAvailable(CF_BITMAP))
{
HWND hPaint;
STARTUPINFO si;
PROCESS_INFORMATION pi;
memset(&si, 0, sizeof(STARTUPINFO));
memset(&pi, 0, sizeof(PROCESS_INFORMATION));
si.cb = sizeof(STARTUPINFO);
wchar_t szWindowDirector[MAX_PATH] = { 0 };
CString strMspaintLoc;
GetSystemDirectory(szWindowDirector, MAX_PATH);
strMspaintLoc.Format(L"%s\\%s", szWindowDirector, L"mspaint.exe");
if (CreateProcess(strMspaintLoc,
NULL, NULL, NULL, FALSE,
NORMAL_PRIORITY_CLASS, NULL, NULL, &si, &pi))
{
=
,
;
.
while (TRUE)
{
Sleep(100);
hPaint = FindWindow(L"MsPaintApp", NULL);
ATLTRACE(L"findwindow Handle %X\n", hPaint);
if (hPaint) break;
}
}
else
{
MessageBox(L"Couldn't find mspaint program");
return;
}
if (OpenClipboard())
{
CloseClipboard();
if (SetForegroundWindow(hPaint))
{
)
INPUT ip;
ip.type = INPUT_KEYBOARD;
ip.ki.wScan = 0;
ip.ki.time = 0;
ip.ki.dwExtraInfo = 0;
y
ip.ki.wVk = VK_CONTROL;
ip.ki.dwFlags = 0; s
SendInput(1, &ip, sizeof(INPUT));
y
ip.ki.wVk = 'V';
ip.ki.dwFlags = 0; s
SendInput(1, &ip, sizeof(INPUT));
y
ip.ki.wVk = 'V';
ip.ki.dwFlags = KEYEVENTF_KEYUP;
SendInput(1, &ip, sizeof(INPUT));
y
ip.ki.wVk = VK_CONTROL;
ip.ki.dwFlags = KEYEVENTF_KEYUP;
SendInput(1, &ip, sizeof(INPUT));
}
}
}
Capturing Screen
CRect rc;
ShowWindow(SW_MINIMIZE);
Sleep(100);
)
CDC dcWindow;
dcWindow.CreateDCW(L"DISPLAY", NULL, NULL, NULL);
t
CMonitorInfo::GetMonitorRect(m_hWnd, rc);
CBitmap bmp, oldbmp;
CDC dcMem;
CClientDC dc(m_hWnd);
dcMem.CreateCompatibleDC();
bmp.CreateCompatibleBitmap(dcWindow, rc.Width(), rc.Height());
oldbmp = dcMem.SelectBitmap(bmp);
dcMem.BitBlt(0, 0, rc.Width(), rc.Height(), dcWindow, rc.left, rc.top, SRCCOPY);
SetCaptureImage(&bmp);
dcMem.SelectBitmap(oldbmp);
ShowWindow(SW_RESTORE);
Capturing a Window
A Window shows up covering your screen after the whole screen has been copied.
void CMCFrameWnd::CaptureWindow()
{
.
CRect rcMonitor;
ShowWindow(SW_MINIMIZE);
Sleep(100);
)
CDC dcWindow;
dcWindow.CreateDCW(L"DISPLAY", NULL, NULL, NULL);
t
CMonitorInfo::GetMonitorRect(m_hWnd, rcMonitor);
CBitmap bmp, oldbmp;
CDC dcMem;
CClientDC dc(m_hWnd);
dcMem.CreateCompatibleDC();
bmp.CreateCompatibleBitmap(dcWindow, rcMonitor.Width(), rcMonitor.Height());
oldbmp = dcMem.SelectBitmap(bmp);
dcMem.BitBlt(0, 0, rcMonitor.Width(),
rcMonitor.Height(), dcWindow, rcMonitor.left, rcMonitor.top, SRCCOPY);
dcMem.SelectBitmap(oldbmp);
if (m_pwndTemparyScreenWnd != NULL)
delete m_pwndTemparyScreenWnd;
m_pwndTemparyScreenWnd = new CTemparyScreenWnd(this);
m_pwndTemparyScreenWnd->mode = CTemparyScreenWnd::Mode::TEMPARYSCREEN_WINDOW;
m_pwndTemparyScreenWnd->Create(m_hWnd, rcMonitor, 0, WS_POPUP | WS_VISIBLE, WS_EX_TOPMOST);
m_pwndTemparyScreenWnd->GetWindowData();
m_pwndTemparyScreenWnd->SetScreenImage(bmp);
m_pwndTemparyScreenWnd->ShowMonitorScreen();
SetForegroundWindow(m_pwndTemparyScreenWnd->m_hWnd);
}