Introduction
This tip explains some intricacies around system overlay permissions introduced in Android M.
Background
Android has allowed applications to draw over all other applications using the SYSTEM_ALERT_WINDOW
permission. This was allowed as long as the permission was declared in the manifest.
="1.0"="utf-8"
<manifest xmlns:android="http://schemas.android.com/apk/res/android">
<application
android:allowBackup="true"
android:icon="@drawable/ic_launcher"
android:label="@string/app_name"
android:theme="@style/AppTheme" >
...
</application>
...
<uses-permission android:name="android.permission.SYSTEM_ALERT_WINDOW" />
</manifest>
This technique was used by several applications that required drawing over other apps. A good example is the Facebook chatheads that float over other apps.
What Happened in M?
In Android M, the SYSTEM_ALERT_WINDOW
permission was categorized as a 'dangerous' permission. This is understandable because you can imagine a transparent application intercepting your touches or inputs. So, instead of allowing the applications to use the overlay with just the manifest declaration, in Android M, the user needs to provide specific permission in Android settings for the app to draw over other apps.
This setting could be found in Settings -> Apps -> Advanced (settings icon) -> Draw over other apps
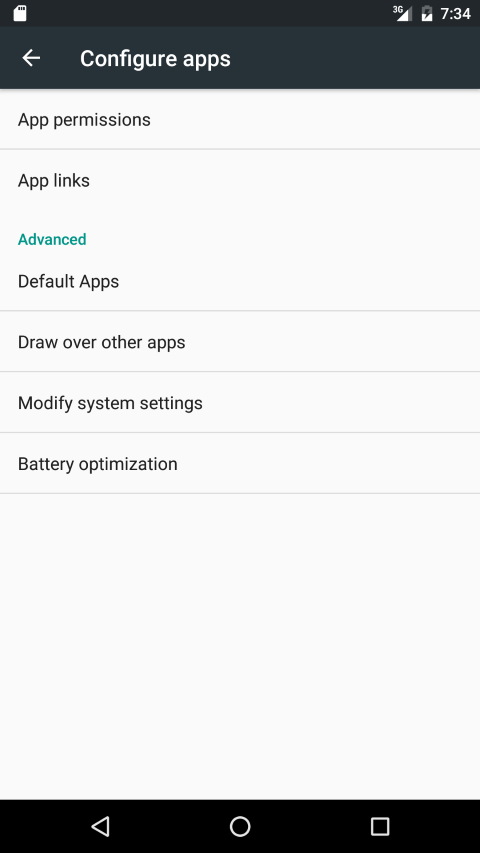
As you may be aware, Android M did introduce fine grained application permissions that the application can request for. But, as this is classified as a dangerous permission, applications cannot request this permission to the user specifically.
Solution
One possible solution is to direct the user to the above screen if the user is running Android M or above. Fortunately, Android does provide a ready made intent action that you could use to launch the above Activity.
Code:
if(Build.VERSION.SDK_INT >= Build.VERSION_CODES.M){
Intent myIntent = new Intent(Settings.ACTION_MANAGE_OVERLAY_PERMISSION);
startActivity(myIntent);
}
That's it. The action ACTION_MANAGE_OVERLAY_PERMISSION
directly launches the 'Draw over other apps' permission screen.