Introduction
Standard pagingtoolbar
implements paging functionality for grid panel, docs here. Number items per page gets from pageSize
of store. This example shows how to do it in runtime.
Actually
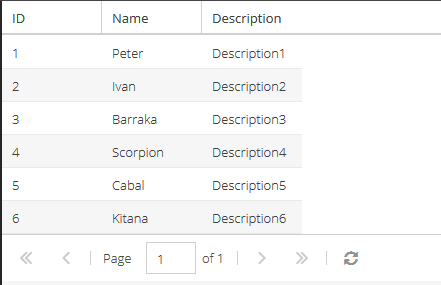
Expected

Using the Code
The full code of example can be found here.
I extend standard pagingtoolbar
:
Ext.define('PagingToolbar', {
extend: 'Ext.toolbar.Paging',
alias: 'widget.resizer.pagingtoolbar',
requires: [
"PagingToolbarResizer"
],
toolbarResizer: null,
initComponent: function () {
var me = this;
me.callParent(arguments);
var pluginClassName = "PagingToolbarResizer";
me.toolbarResizer = Ext.create(pluginClassName);
if (Ext.isEmpty(me.plugins)) {
me.plugins = [me.toolbarResizer];
}
else {
var pushTbResizer = true;
Ext.each(me.plugins, function (plug in) {
if (Ext.getClassName(plugin) == pluginClassName) {
pushTbResizer = false;
}
});
if (pushTbResizer) {
me.plugins.push(me.toolbarResizer);
}
}
},
bindStore: function (store, initial, propertyName) {
var me = this;
me.callParent(arguments);
if (!Ext.isEmpty(me.toolbarResizer) &&
!Ext.isEmpty(me.toolbarResizer.recordsPerPageCmb) && !Ext.isEmpty(store)) {
me.toolbarResizer.recordsPerPageCmb.setValue(store.pageSize);
}
}
});
Create plugun for tollbar:
Ext.define('PagingToolbarResizer', {
extend: 'Ext.AbstractPlugin',
alias: 'plugin.pagingtoolbarresizer',
options: [5, 10, 15, 20, 25, 30, 50, 75, 100, 200, 300, 500, 1000],
mode: 'remote',
displayText: 'Records per Page',
recordsPerPageCmb: null,
constructor: function (config) {
Ext.apply(this, config);
this.callParent(arguments);
},
init: function (pagingToolbar) {
var me = this;
var comboStore = me.options;
me.recordsPerPageCmb = Ext.create('Ext.form.field.ComboBox', {
typeAhead: false,
triggerAction: 'all',
forceSelection: true,
lazyRender: true,
editable: false,
mode: me.mode,
value: pagingToolbar.store.pageSize,
width: 80,
store: comboStore,
listeners: {
select: function (combo, value, i) {
pagingToolbar.store.pageSize = value.data.field1;
pagingToolbar.store.load();
}
}
});
var index = pagingToolbar.items.indexOf(pagingToolbar.refresh);
pagingToolbar.insert(++index, me.displayText);
pagingToolbar.insert(++index, me.recordsPerPageCmb);
pagingToolbar.insert(++index, '-');
pagingToolbar.on({
beforedestroy: function () {
me.recordsPerPageCmb.destroy();
}
});
}
});
Add this toolbar in grid. This allows to select number of records per page on runtime.
Ext.define('GridView', {
extend: 'Ext.grid.Panel',
requires: [
'Ext.grid.column.Number',
'Ext.grid.column.Date',
'Ext.view.Table',
'PagingToolbar',
'Ext.selection.RowModel'
],
viewModel: {
stores: {
itemsStore: {
proxy: {
type: 'ajax',
url: 'users.json',
reader: {
type: 'json',
rootProperty: 'users'
}
},
autoLoad: true
}
}
},
bind: {
store: '{itemsStore}'
},
columns: [
{
xtype: 'numbercolumn',
dataIndex: 'id',
text: 'ID',
format: '0,000'
},
{
xtype: 'gridcolumn',
dataIndex: 'name',
text: 'Name'
},
{
xtype: 'gridcolumn',
dataIndex: 'description',
text: 'Description'
}
],
dockedItems: [
{
xtype: 'pagingtoolbar',
dock: 'bottom',
displayInfo: true
}
],
selModel: {
selType: 'rowmodel',
mode: 'MULTI'
}
});