Introduction
Greetings from Germany! In this tip, I will show you a class that allows to encrypt and decrypt string
s with a password.
Background
If you don't know what AES or SHA2 is, please read the wikipedia articles...
First, I create a hash with SHA2 (256 bit) from the password, this hash is the key and IV in the AES Encryption. The IV can only be 128 bit, so I just cut it to the right length.
You can only decrypt it if you have the password.
Using the Code
SecurityController.cs class code:
using System;
using System.IO;
using System.Security.Cryptography;
using System.Text;
namespace Encryption
{
class SecurityController
{
public string Encrypt( string key, string data )
{
string encData = null;
byte[][] keys = GetHashKeys(key);
try
{
encData = EncryptStringToBytes_Aes( data, keys[0], keys[1] );
}
catch ( CryptographicException ) { }
catch ( ArgumentNullException ) { }
return encData;
}
public string Decrypt( string key, string data )
{
string decData = null;
byte[][] keys = GetHashKeys(key);
try
{
decData = DecryptStringFromBytes_Aes( data, keys[0], keys[1] );
}
catch ( CryptographicException ) { }
catch ( ArgumentNullException ) { }
return decData;
}
private byte[][] GetHashKeys( string key )
{
byte[][] result = new byte[2][];
Encoding enc = Encoding.UTF8;
SHA256 sha2 = new SHA256CryptoServiceProvider();
byte[] rawKey = enc.GetBytes(key);
byte[] rawIV = enc.GetBytes(key);
byte[] hashKey = sha2.ComputeHash(rawKey);
byte[] hashIV = sha2.ComputeHash(rawIV);
Array.Resize( ref hashIV, 16 );
result[0] = hashKey;
result[1] = hashIV;
return result;
}
private static string EncryptStringToBytes_Aes( string plainText, byte[] Key, byte[] IV )
{
if ( plainText == null || plainText.Length <= 0 )
throw new ArgumentNullException( "plainText" );
if ( Key == null || Key.Length <= 0 )
throw new ArgumentNullException( "Key" );
if ( IV == null || IV.Length <= 0 )
throw new ArgumentNullException( "IV" );
byte[] encrypted;
using ( AesManaged aesAlg = new AesManaged() )
{
aesAlg.Key = Key;
aesAlg.IV = IV;
ICryptoTransform encryptor = aesAlg.CreateEncryptor(aesAlg.Key, aesAlg.IV);
using ( MemoryStream msEncrypt = new MemoryStream() )
{
using ( CryptoStream csEncrypt =
new CryptoStream( msEncrypt, encryptor, CryptoStreamMode.Write ) )
{
using ( StreamWriter swEncrypt = new StreamWriter( csEncrypt ) )
{
swEncrypt.Write( plainText );
}
encrypted = msEncrypt.ToArray();
}
}
}
return Convert.ToBase64String( encrypted );
}
private static string DecryptStringFromBytes_Aes( string cipherTextString, byte[] Key, byte[] IV )
{
byte[] cipherText = Convert.FromBase64String(cipherTextString);
if ( cipherText == null || cipherText.Length <= 0 )
throw new ArgumentNullException( "cipherText" );
if ( Key == null || Key.Length <= 0 )
throw new ArgumentNullException( "Key" );
if ( IV == null || IV.Length <= 0 )
throw new ArgumentNullException( "IV" );
string plaintext = null;
using ( Aes aesAlg = Aes.Create() )
{
aesAlg.Key = Key;
aesAlg.IV = IV;
ICryptoTransform decryptor = aesAlg.CreateDecryptor(aesAlg.Key, aesAlg.IV);
using ( MemoryStream msDecrypt = new MemoryStream( cipherText ) )
{
using ( CryptoStream csDecrypt =
new CryptoStream( msDecrypt, decryptor, CryptoStreamMode.Read ) )
{
using ( StreamReader srDecrypt = new StreamReader( csDecrypt ) )
{
plaintext = srDecrypt.ReadToEnd();
}
}
}
}
return plaintext;
}
}
}
Example How To Use It
MainForm.cs class code:
using System.Windows.Forms;
namespace Encryption
{
public partial class MainForm : Form
{
private SecurityController _security;
public MainForm()
{
InitializeComponent();
_security = new SecurityController();
}
private void button_encrypt_Click( object sender, System.EventArgs e )
{
string password = textBox_password.Text;
string notEncryptedText = textBox_text.Text;
string encryptedText = _security.Encrypt( password, notEncryptedText );
textBox_text.Text = encryptedText;
}
private void button_decrypt_Click( object sender, System.EventArgs e )
{
string password = textBox_password.Text;
string encryptedText = textBox_text.Text;
string notEncryptedText = _security.Decrypt( password, encryptedText );
textBox_text.Text = notEncryptedText;
}
}
}
Before Encryption
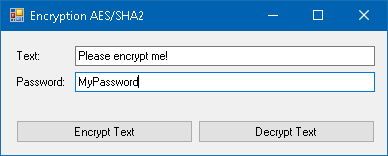
After Encryption
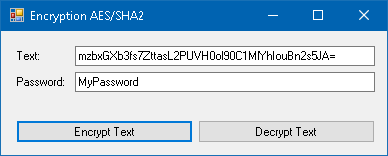
After Decryption
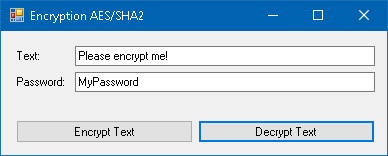
Conclusion
I know it's very simple and not a lot of code, but if you don't know much about encryption and you need it, this class will help you a lot!