Introduction
I am writing the article Develop Angular2 Application using Visual Studio Code using TypeScript based Angular2. Somebody asked me why not use the pure JavaScript version Angular2? I know that using vanilla JavaScript is very cool because everything we are using in JavaScript is based on it. But in some cases, it doesn't tell you right or wrong. It will tell you "I AM NOT SURE". But I am sure sometimes it will definitely make you CRAZY. See if you will get crazy after you finish reading this.
Some Test of VanillaJS
console.log("==================================");
var myValueIsNull = null;
console.log("typeof myValueIsNull is " + typeof myValueIsNull);
var myValueIsObject = new Object();
console.log("typeof myValueIsObject is " + typeof myValueIsObject);
var iAmAFunction = new function() {};
console.log("typeof iAmAFunction is " + typeof iAmAFunction);
var iAmAnArray = [];
console.log("typeof iAmAnArray is " + typeof iAmAnArray);
var myTypeIsInt = 1;
console.log("typeof myTypeIsInt is " + typeof myTypeIsInt);
var myTypeIsString = "abc";
console.log("typeof myTypeIsString is " + typeof myTypeIsString);
var myTypeIsDate = new Date();
console.log("typeof myTypeIsDate is " + typeof myTypeIsDate);
console.log("==================================");
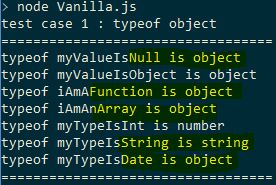
So the result is: null
is object, function is object, array is object ......, you may think, that's cool, they are actually objects. Yes, they are. But string
is string
. That's OK, because string
is special... Suppose you asked me "what's your name?", I told you "my father's name is XXX". I think you are going to be crazy.
If you still think type is not important, let's see the code below:
console.log("==================================");
iAmAnUndefinedDate = new Date();
console.log("typeof iAmAnUndefinedDate is " + typeof iAmAnUndefinedDate);
iAmAnUndefinedDate = '08/15/2008';
console.log("typeof iAmAnUndefinedDate is " + typeof iAmAnUndefinedDate);
var i = Math.floor(Math.random() * (100 - 1) + 1);
console.log(i);
if (i % 2 === 0) {
iAmAnUndefinedDate = '08/15/2008';
} else {
iAmAnUndefinedDate = new Date();
}
console.log("is iAmAnUndefinedDate an object? " + typeof iAmAnUndefinedDate === "object");
console.log("==================================");
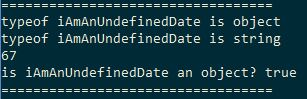
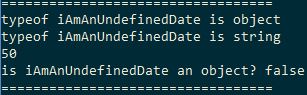
Yes, the type of a var
can be changed dynamically! So cool! But you will be crazy if someone changed your var
type in a large project.
If you still think this one is OK, let's see the next one.
console.log("==================================");
function return1()
{
return {
str1: "Hi 1"
};
}
function return2()
{
return
{
str2: "Hi 2"
};
}
function return3()
{
return;
{
str3: "Hi 3"
};
}
console.log(return1);
console.log(return2);
console.log(return3);
console.log(return1());
console.log(return2());
console.log(return3());
console.log("==================================");
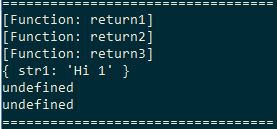
No exception! But unexpected result. Crazy! See return1
and return2
, don't you think they are the same code? In fact, return2
is the same as return3
.
Another crazy thing is the number.
console.log("==================================");
console.log(1 + 1);
console.log(1.1 + 1.1);
console.log(0.1 + 0.1);
console.log(0.1 + 0.2);
console.log(0.2 + 0.2);
console.log(0.2 + 0.2 == 0.4);
console.log(0.1 + 0.2 == 0.3);
console.log("==================================");
Let's see the result.
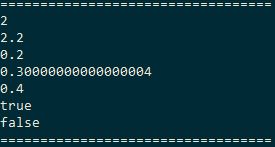
It's real.
But these are just the tip of the iceberg. That's why we need TypeScript to help us reduce crazy results. Why not use it? In the next chapter, I will continue to make some changes on the NgTweet Angular2 app.
Summary
Try to hug new things, life may become better.
History