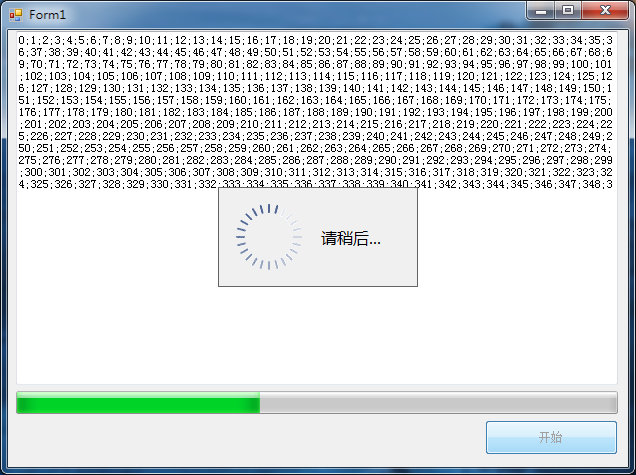
Introduction
This article describes a simple method to display a waiting dialog and not block the main form.
Background
When I create a WinForm application by C#, it's very troublesome to show a waiting dialog. Sometimes I do it, but not beautiful or hard to change style.
Structure
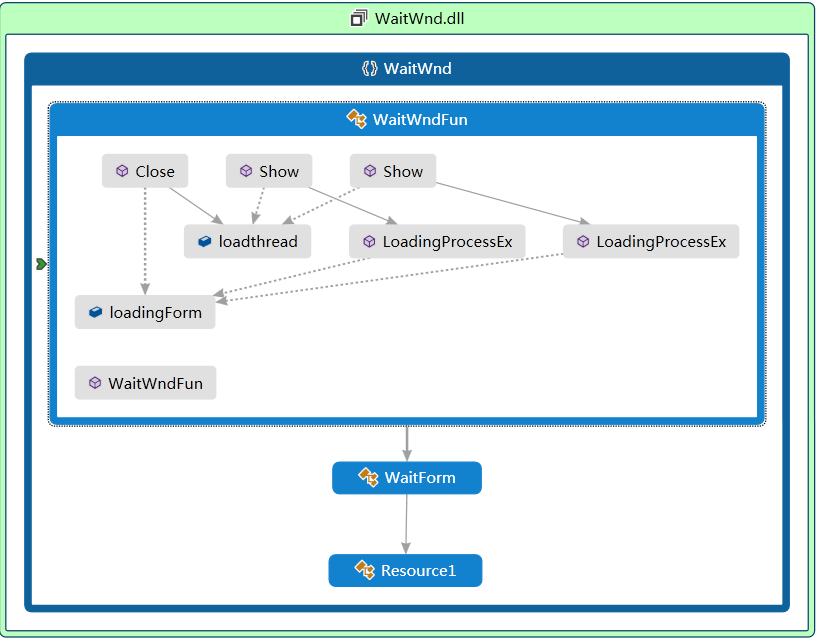
I use a class library, WaitForm
is a waiting dialog, and WaitWndFun
is a class to show the form or close by create thread,
WaitWndFun Class Function
Show Function
...
public void Show(Form parent)
{
loadthread = new Thread(new ParameterizedThreadStart(LoadingProcessEx));
loadthread.Start(parent);
}
...
Close Function
public void Close()
{
if (loadingForm != null)
{
loadingForm.BeginInvoke(new System.Threading.ThreadStart(loadingForm.CloseLoadingForm));
loadingForm = null;
loadthread = null;
}
}
Thread Method
private void LoadingProcessEx()
{
loadingForm = new WaitForm();
loadingForm.ShowDialog();
}
private void LoadingProcessEx(object parent)
{
Form Cparent = parent as Form;
loadingForm = new WaitForm(Cparent);
loadingForm.ShowDialog();
}
When closing dialog, there is a function to close and dispose the form in WaitForm
.
public void CloseLoadingForm()
{
this.DialogResult = DialogResult.OK;
this.Close();
if (label1.Image != null)
{
label1.Image.Dispose();
}
}
And when there is a parent form, we should show dialog in center of parent.
public WaitForm(Form parent)
{
InitializeComponent();
if (parent != null)
{
this.StartPosition = FormStartPosition.Manual;
this.Location = new Point(parent.Location.X + parent.Width / 2 - this.Width / 2,
parent.Location.Y + parent.Height / 2 - this.Height / 2);
}
else
{
this.StartPosition = FormStartPosition.CenterParent;
}
}
How to Use
It is very easy to use.
- Reference the WaitWnd.dll or add sources file to your project.
- Change the style of waiting form, you can change the picture and text of label to your liking in the form designer.
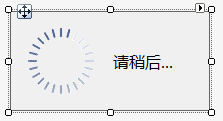
- Create a new
WaitWndFun
object. When you call show function, the waiting form will appear, and when you call close
function, the waiting form will close.
WaitWnd.WaitWndFun waitForm = new WaitWnd.WaitWndFun();
private void button1_Click(object sender, EventArgs e)
{
waitForm.Show(this);
waitForm.Close();
}