Introduction
This tip discusses how to consume external services inside AX 365. In this post, I am using channel advisor restful service to be consumed from Microsoft Dynamics 365. This will also help you know about calling GET
, POST
of restful service.
Background
I got a requirement of importing sales orders from channel advisor in AX , but after researching many websites, I haven't got a proper solution. I got everything in bits and pieces, so I thought of writing everything in one article.
Prerequisite
Create an account on "https://developer.channeladvisor.com/the-developer-console" for application key, secret key and refresh token.
Using the Code
- Create a new Class Library project in Visual Studio, and name it
CAIntegration
as shown in the screenshot:
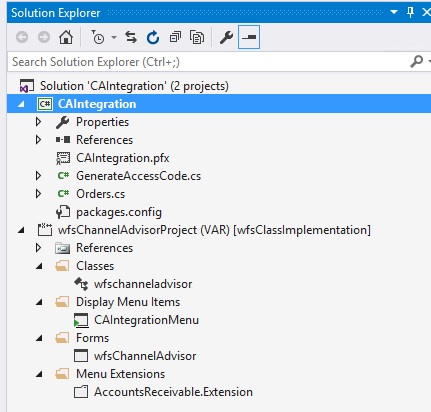
- Create a Class Generateaccesscode.cs as shown below:
public string GetAccessTokenByKey()
{
string content, accesstoken;
var bytes = Encoding.UTF8.GetBytes
("[application id]:[secret key]");
var base64 = Convert.ToBase64String(bytes);
Console.WriteLine(base64);
var client = new RestClient("https://api.channeladvisor.com/oauth2/token");
var request = new RestRequest(Method.POST);
request.AddParameter("Content-Type",
"application/x-www-form-urlencoded", ParameterType.HttpHeader);
request.AddParameter("Cache-Control", "no-cache", ParameterType.HttpHeader);
request.AddParameter("Authorization", "Basic " + base64, ParameterType.HttpHeader);
request.AddParameter("application/x-www-form-urlencoded",
"grant_type=refresh_token&refresh_token=[RefreshToken]", ParameterType.RequestBody);
IRestResponse response = client.Execute(request);
content = response.Content;
string json = content;
XmlDocument doc =
(XmlDocument)JsonConvert.DeserializeXmlNode(json, "Channeladvisor");
XmlElement root = doc.DocumentElement;
XmlNodeList nodes = root.SelectNodes("//Channeladvisor");
accesstoken = "";
foreach (XmlNode node in nodes)
{
accesstoken = node["access_token"].InnerText;
Console.WriteLine(node["access_token"].InnerText);
}
return accesstoken;
}...
- Create another class for getting sales order from channel advisor by passing access token above methods.
public string GetAllOrders(string accesstoken)
{
string xml;
var client =
new RestClient
("https://api.channeladvisor.com/v1/orders?access_token=" + accesstoken);
var request = new RestRequest(Method.GET);
IRestResponse response = client.Execute(request);
var content = response.Content;
string json = content;
XmlDocument doc =
(XmlDocument)JsonConvert.DeserializeXmlNode(json, "Channeladvisor");
xml = doc.InnerXml.ToString();
return xml;
}
...
- Deploy the class library.
- Create a new Dynamics project in Visual Studio as shown in the screenshot.

- Add CAIntegration.dll as a reference.
- In the X++ class, you can use the external web services that were referenced in CAIntegration.dll.
[FormControlEventHandler(formControlStr
(wfsChannelAdvisor, FormButtonControl1), FormControlEventType::Clicked)]
public static void FormButtonControl1_OnClicked(FormControl sender, FormControlEventArgs e)
{
Notes getOrdersXML;
CAIntegration.GenerateAccessCode accessCode = new CAIntegration.GenerateAccessCode();
String255 accessCode1 = accessCode.GetAccessTokenByKey();
info(strFmt("Access code %1",accessCode1));
CAIntegration.Orders orders = new CAIntegration.Orders();
getOrdersXML = orders.GetAllOrders(accessCode1);
info(strFmt("orderxml %1",getOrdersXML));
} ...
History
- 28th July, 2017: Initial version