Introduction
Sometimes, you have to wonder what the folks at Microsoft are thinking. If you have tried the offline installation mode with the new Visual Studio 2017 installation mechanism, you will see that on subsequent update downloads, your offline folder size will increase, that is to say the old versions are not deleted and the full install 28Gb directory will just get bigger.
To help relieve you of the burden on disk, I have written a small program that will cleanup that folder (it should be in the VS installer, but it's not).
How To Use It
The usage is pretty simple, just copy the EXE and the DLL file into the offline installation folder and run from there, and you will see the output like below:
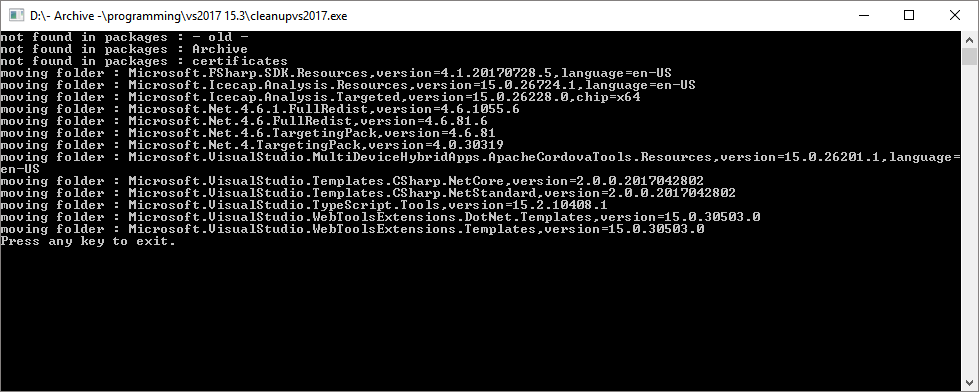
What's in the Code
The code is very simple, it reads the catalog.json file and goes through all the folders of VS2017 and tries to match the package id
and version
number, if none is found, it presumes it is an old version and moves that folder to the - old -
folder, where you can delete it later.
class Program
{
static void Main(string[] args)
{
string path = Directory.GetCurrentDirectory();
if (path.EndsWith("\\") == false) path += "\\";
string catalog = path + "catalog.json";
if (File.Exists(catalog) == false)
{
Console.WriteLine("Please copy this program to the VS2017 offline folder.");
return;
}
string old = Directory.CreateDirectory(path + "- old -").FullName;
var o = fastJSON.JSON.ToDynamic(File.ReadAllText(catalog));
var folders = Directory.GetDirectories(path);
var packages = o["packages"];
foreach (var folder in folders)
{
var foldername = Path.GetFileName(folder);
var splitstring = foldername.Split(',');
bool found = false;
if (splitstring.Length > 1)
{
foreach (var package in packages)
{
if (package["id"].ToString() == splitstring[0] &&
package["version"] == splitstring[1].Split('=')[1].Trim())
{
found = true;
break;
}
}
}
else
{
found = true;
Console.WriteLine("not found in packages : " + foldername);
}
if (found == false)
{
Console.WriteLine("moving folder : " + foldername);
Directory.Move(folder, old + "\\" + foldername);
}
}
Console.WriteLine("Press any key to exit.");
Console.ReadKey();
}
}
Points of Interest
I'm using my fastJSON
library to parse the catalog.json file in the installation folder and using ToDynamic()
to simplify the coding.
History
- 21st August 2017: Initial version 1.0.0