The native Windows Forms control datagridview doesn’t support Master-Detail views within a single control. By majority approach, this should be done through two separate datagridview controls. In this tip, I will discuss my kind of approach on how to display Master-Detail data views inside a single datagridview control. The typical layout we need is shown in a screenshot and the full code overview has been provided.
Credits
This tip is the C# translate version of Master Detail Datagridview. Review the original article for further details.
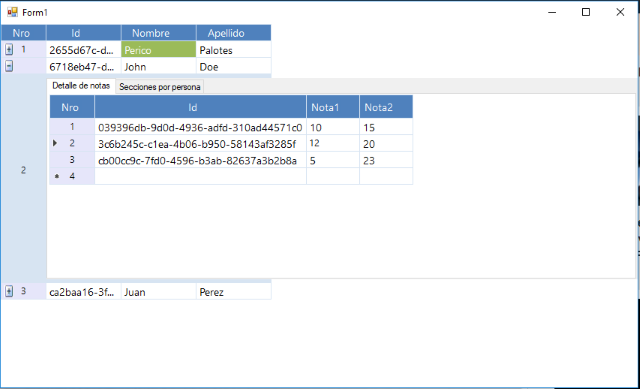
Introduction
Many of us developers come across a problem on how to display Master-Detail views within a single Datagridview
control like the third party applications Devexpress.
The native Windows Forms control datagridview
doesn’t support Master-Detail views within a single control. By majority approach, this should be done through two separate datagridview
controls.
In this tip, I will discuss with you my kind of approach on how to display Master-Detail data views inside a single datagridview
control. Although an alternate Datagrid
control supports master detail datagridview
, expanding a child datagridview
will occupy the whole control which will give you not a good layout datagridview
s to your data as needed for data validation. The typical layout we need is what we could see in the attached screenshot.
Improvements
- The grids are populated with
List<>
objects. - The grids are fully editable.
- The grids show a tooltip based on description attribute of every property of the class.
- Fixed an issue that prevents moving through the child grid with arrow keys.
Using the Code
In this sample demo, I used a single List<>
collection of objects.
Follow these very simple steps:
- Declare a variable referenced to
MasterControl
:
MasterGridView masterGridView1
- Load data:
private void Form1_Load(object sender, EventArgs e)
{
masterGridView1.DataSource = Persona.getPersonas();
}
- Set child
TabControl
on the same hierarchy than parent datagridview
:
masterGridView1.SetChildTabcontrol();
Full Code Overview
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace WindowsFormsApplication3
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
masterGridView1.SetChildTabcontrol();
}
private void Form1_Load(object sender, EventArgs e)
{
masterGridView1.DataSource = Persona.getPersonas();
}
}
}
History
- 13th February, 2020: Initial version