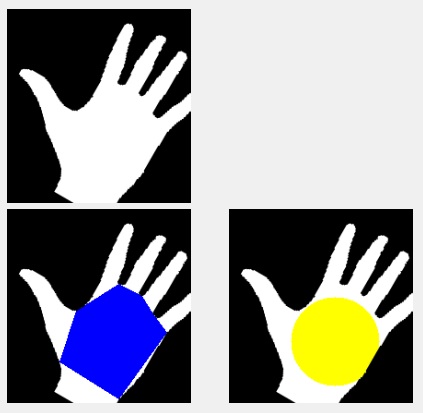
Introduction
Get the palm hand region using convexity defect. Convexity defect is used to detect the base of a finger.
Output: The original region of palm hand or circle region of the palm. Circle region is often used on palm hand recognition to support hand rotation.
Input: binary image, so you need to do segmentation first.
Background
This process runs based on the convexity defect method. (Source: http://opencv.willowgarage.com )

- First, it finds the hull of the object; in the image, the hull is the red line.
- Then from the hull, it finds the convexity defect.
- Convexity defect consist of four elements:
- start points: points where the defect begin
- end points: points where the defect end
- depth points: points that are farthest from the hull within the defect (between its start point and depth point)
- distance: distance between
depth point to the hull
- From every depth point we treat it as the boundary of the palm. Depth point should be on the palm region, but often depth points are detected on the finger. So, I use some parameter
to filter the depth point that is detected on the finger based on its distance.
- After I filter the depth points, I draw it to the image.
Using the code
To use this code, you just simply create the object from the class palmHandSegmentation
. Here is the example:
palmHandSegmentation PHS = new palmHandSegmentation();
Image<Gray, byte> binaryHandImage = new Image<Gray, byte>("img/hand1.bmp");
Image<Gray, byte> palmOfHand = PHS.getPalm(binaryHandImage);
Image<Gray, byte> circlePalmOfHand = PHS.getCirclePalm(binaryHandImage);
You can also make adjustments on the parameter. The parameters are on the class palmHandSegmentation
on the file 'palmHandSegmentation.cs'.
These parameters you can make adjustments on 'palmHandSegmentation.cs':
double minimumDistanceDepthPointToEndPointRatio = 0.15;
double circleRadiusMultiplier = 1.1;
Recommendation
To create the circle region, I use a minimum enclosing circle from the depth points, then I take its center to create a new circle with the same center and radius that is from the distance from the center to the contour_of the hand. You can also obtain the center by computing the central moment from the palm region.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.