Introduction
KeePass is an open source password manager. The passwords are stored in a database with the .kdbx extension. The database can be on your local file system or on a web server.
If you put the database on an IIS webserver, you can access it from KeePass, but if you try to save, you get a 404 error. This is because the StaticFile
HTTP handler in IIS only supports the GET
method and KeePass requires PUT
, MOVE
and DELETE
, in addition to GET
. To add support for this, a custom HTTP handler has to be added to the webserver.
I have tested the solution in IIS 7.5, but it will probably work in IIS 7 as well.
Using the Code
The HTTP handler is a C# library project with a class that implements IHttpHandler
. A reference to System.Web
needs to be added.
using System.IO;
using System.Linq;
using System.Web;
public class UploadHandler : IHttpHandler
{
public void ProcessRequest(HttpContext context)
{
string path = Path.Combine(context.Request.PhysicalApplicationPath,
context.Request.Url.Segments.Last());
if (context.Request.RequestType == "DELETE")
{
File.Delete(path);
}
else if (context.Request.RequestType == "MOVE")
{
string destination = Path.Combine(context.Request.PhysicalApplicationPath,
context.Request.Headers["Destination"]
.Split(new char[] { '/' }).Last());
File.Move(path, destination);
}
else if (context.Request.RequestType == "PUT")
{
byte[] fileBytes;
using (var reader = new BinaryReader(context.Request.InputStream))
{
fileBytes = reader.ReadBytes((int)context.Request.InputStream.Length);
}
File.WriteAllBytes(path, fileBytes);
}
}
public bool IsReusable
{
get { return false; }
}
}
To add the handler, put the following in web.config:
<configuration>
<system.webServer>
<staticContent>
<mimeMap mimeType="application/octet-stream" fileExtension=".kdbx" />
</staticContent>
<handlers>
<add name="UploadHandler_tmp" requireAccess="Read"
resourceType="Unspecified" type="UploadHandler"
verb="PUT,DELETE,MOVE" path="*.tmp" />
<add name="UploadHandler_kdbx" requireAccess="Read"
resourceType="Unspecified" type="UploadHandler"
verb="DELETE" path="*.kdbx" />
</handlers>
</system.webServer>
</configuration>
Put the library DLL in a folder named "bin" on the web site.
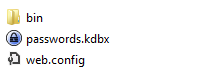
Now, you should be able to save your KeePass database!
History