Introduction
Finding Fibonacci sequence can be done by a simple logic:
int fibonaci(int n){
int i,next,a=0,b=1;
for(i=0;i<n;++i){
if(i<=1){
next = i;
}else{
next = a + b;
a = b;
b= next;
}
printf("%d ",next);
}
}
But here's a math formula. It can solve the case too.
The formula : Fn = (x1n – x2n) / root(5) (Fn is rounded down)
where X1 and X2 are the roots of x2 - x - 1=0 equation
to find X1 and X2 , we can use the following formula:
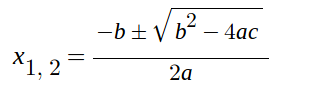
So that X1,X2 => 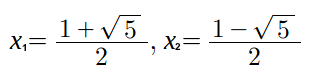
int fibonaci(int n){
int i;float tmp;
float x1 = ( 1 + sqrt(5) ) / 2;
float x2 = (1 - sqrt(5) ) / 2;
for(i=0;i<n;i++){
tmp = (pow(x1,i) - pow(x2,i)) / sqrt(5);
printf("%d ",(int)floor(tmp)); }
printf("\n");
}
Output: 0 1 1 2......so on
Of course, we don't need to use the math formula in programming, this is just an example.