Introduction
The Karnaugh map, also known as the K-map, is a method to simplify boolean algebra.
Karnaugh maps are used to facilitate the simplification of Boolean algebra functions. Take the Boolean function described by the following truth table.
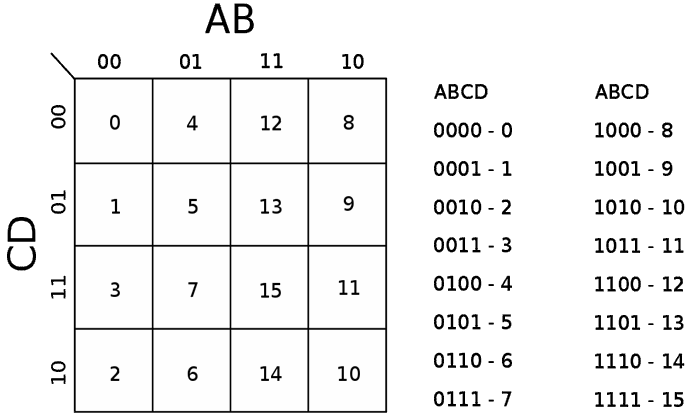
Figure1 truth table [1]
This article, and especially the attached code, is for those want to know how KARNAUGH table reduice an algebra expression.
Requirements
The reader should have basic notions of boolean algebra; Knowledge of the Quine McKluskey algorithm is optimal. Basic notions of C# are required for understanding the code.
Using of the Application
First, we have to introduce the boolean expression in the textbox. This one must use only (a;b:c:d;!,+) characters, then we click the button of table truth generation to fill our listview by the appropriate values.
Once the truth table is filled, we can process to generation of the Karnaugh table by clicking the button named Kanaugh.

To simplify the expression in codesource, we use two methods, the first is to simplify two terms and return the simplified expression.
public string simplifier1(int i,int j,int order)
{
int pos = -1;
var dd = Form1.local_tab[i];
var ff = new StringBuilder(dd);
if (shift(Form1.local_tab[i], Form1.local_tab[j]) == 1)
{
ff.Remove(pos, 1);
ff.Insert(pos, "-");
lstTerms[order].SetItemChecked(i, true);
lstTerms[order].SetItemChecked(j, true);
}
return ff.ToString();
}
The second makes all simplifications possible in the Karnaugh table.
public void evaluer(int order)
{
int s = 0;
for (int i = 0; i < Form1.j; i++)
{
for (int k = i; k < Form1.j; k++)
{
simplifier1(i, k, order);
if ((lstTerms[order].GetItemCheckState(i) == CheckState.Checked) &&
(lstTerms[order].GetItemCheckState(k) == CheckState.Checked))
{
if (!tab.Contains(simplifier1(i, k, order)) &&
!Form1.local_tab.Contains(simplifier1(i, k, order)))
{
tab[s] = simplifier1(i, k, order);
lstTerms[order+1].Items.Add(tab[s]);
lstTerms[order+1].SetItemChecked(s, false);
s++;
}
}
}
}
for (int i = 0; i < Form1.j; i++)
{
Form1.local_tab[i] = " ";
}
Form1.j = s;
for (int i = 0; i < Form1.j; i++)
{
Form1.local_tab[i] = tab[i];
tab[i] = " ";
}
if (s == 0)
arret = true;
}
This is what we have as result:

Reference an Attribution
[1} https://commons.wikimedia.org/wiki/File:K-map_minterms_A.svg
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.