Introduction
When we are using knockout validation and bootstrap together, we may face problems with the alignment of error messages with bootstrap input-group session. Like below:
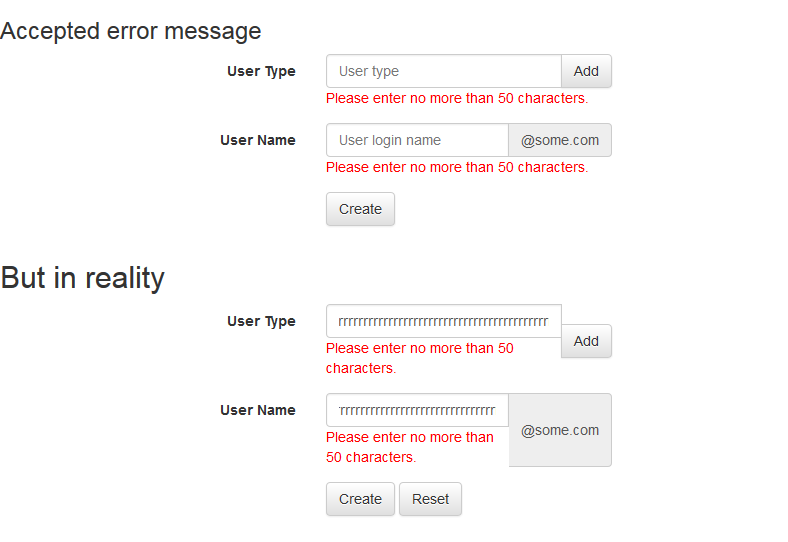
Background
Let’s say we have a .from-group div
which contains an input field, inside a .input-group div
.
<div class="form-group">
<label class="col-sm-2 control-label">User Type</label>
<div class="col-sm-3">
<!--
<div class="input-group">
<input type="text" class="form-control"
placeholder="User type" data-bind="value: userType"/>
<span class="input-group-btn">
<button class="btn btn-default" type="button">Add</button>
</span>
<!--
</div>
</div>
</div>
Now what are the options to solve such a problem?
- Using a custom binding handler to populate error message holder
- Using inline error message holder for each field in HTML DOM
1. Using a Custom bindingHandler
jsfiddle
Here is the custom binding handler, with specifications:
- What type of error message holder should be used (
span
) - What would be the class of error message (
.validatationMessage
) - If the input is inside a holder (of
.input-group
) append error mgs just after it. - If not, append error mgs just after itself
ko.bindingHandlers.validationCore = {
init: function(element, valueAccessor, allBindingsAccessor, viewModel, bindingContext) {
var span = document.createElement('SPAN');
span.className = 'validationMessage';
var parent = $(element).parent().closest
(".input-group");
if (parent.length > 0) {
$(parent).after(span);
} else {
$(element).after(span);
}
ko.applyBindingsToNode(span, { validationMessage: valueAccessor() });
}
};
2. Using Inline Error Message Holder
Place error message holder at desirable place. The "validationMessage: userType
" is important, to specify this holder is for which field.
<div class="form-group">
<label class="col-sm-2 control-label">User Type</label>
<div class="col-sm-3">
<!--
<div class="input-group">
<input type="text" class="form-control"
placeholder="User type" data-bind="value: userType"/>
<span class="input-group-btn">
<button class="btn btn-default" type="button">Add</button>
</span>
</div>
<!--
<!--
<span class="validationMessage"
data-bind="validationMessage: userType"></span>
</div>
</div>
Now, specify not to add error message automatically:
/*error span would not be inserted
and we have to specify the location of error message*/
ko.validation.init({ insertMessages: false });