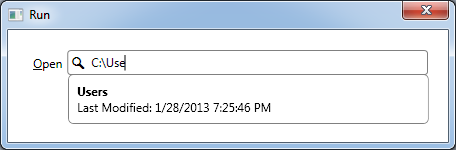
Introduction
In this article I will show you how to use an auto-complete/auto-suggest TextBox control in WPF. I have tried to keep the control simple and easy to use, yet fully featured and supporting MVVM.
Background
I was once required to use a control in my project which can show suggestions to user while he/she is typing in the test box. I found my controls over the internet after googling for few hours, however none of them met my requirements. Finally I decided to create my own from the scratch.
I decided to keep it open-source and publised the source code at CodePlex. You may download the latest source code from http://wpfautocomplete.codeplex.com/.
Using the code
The control uses ISuggestionProvider
interface for displaying suggestions. ISuggestionProvider
contains just one method GetSuggestions
.
Let's create a simple suggestion provider for file-system.
Public Class FilesystemSuggestionProvider
Implements ISuggestionProvider
Public Function GetSuggestions(ByVal filter As String) As System.Collections.IEnumerable Implements ISuggestionProvider.GetSuggestions
If String.IsNullOrEmpty(filter) Then
Return Nothing
End If
If filter.Length < 3 Then
Return Nothing
End If
If filter(1) <> ":"c Then
Return Nothing
End If
Dim lst As New List(Of IO.FileSystemInfo)
Dim dirFilter As String = "*"
Dim dirPath As String = filter
If Not filter.EndsWith("\") Then
Dim index As Integer = filter.LastIndexOf("\")
dirPath = filter.Substring(0, index + 1)
dirFilter = filter.Substring(index + 1) + "*"
End If
Dim dirInfo As IO.DirectoryInfo = New IO.DirectoryInfo(dirPath)
lst.AddRange(dirInfo.GetFileSystemInfos(dirFilter))
System.Threading.Thread.Sleep(2000)
Return lst
End Function
End Class
Now we have created our suggestion provider. Let's add the control to our view. Adding the control to your view is very simple.
First of all you need to import the namespace of library in your view to add the control in your view.
xmlns:wpf="http://wpfcontrols.com/"
Adding control to view:
<wpf:AutoCompleteTextBox VerticalAlignment="Top"
SelectedItem="{Binding Path=SelectedPath, Mode=TwoWay}"
DisplayMember="FullName"
ItemTemplate="{StaticResource ResourceKey=fsTemplate}"
Watermark="Search here"
IconPlacement="Left"
IconVisibility="Visible"
Provider="{StaticResource ResourceKey=fsp}">
<wpf:AutoCompleteTextBox.LoadingContent>
<TextBlock Text="Loading..."
Margin="5"
FontSize="14" />
</wpf:AutoCompleteTextBox.LoadingContent>
<wpf:AutoCompleteTextBox.Icon>
<Border Width="20"
Height="20">
<TextBlock Text="??"
FontSize="14"
FontFamily="Segoe UI Symbol"
HorizontalAlignment="Center"
VerticalAlignment="Center" />
</Border>
</wpf:AutoCompleteTextBox.Icon>
</wpf:AutoCompleteTextBox>
Adding ItemTemplate and FileSystemSuggestionProvider
in Resources.
<local:FilesystemSuggestionProvider x:Key="fsp" />
<DataTemplate x:Key="fsTemplate">
<Border Padding="5">
<StackPanel Orientation="Vertical">
<TextBlock Text="{Binding Path=Name}"
FontWeight="Bold" />
<TextBlock Text="{Binding Path=LastWriteTime, StringFormat='Last Modified: {0}'}" />
</StackPanel>
</Border>
</DataTemplate>
History
Please visit CodePlex for version history and downloading latest version of the AutoCompleteTextBox.